在Python中,连接数据库的方式多种多样,可以使用不同的库和方法来连接不同类型的数据库,如MySQL、PostgreSQL、SQLite等。常用的库包括mysql-connector-python
、psycopg2
、sqlite3
等。以下是一些关键点:选择合适的数据库连接库、安装并导入库、配置数据库连接参数、建立连接。
选择合适的数据库连接库是连接数据库的第一步。不同的数据库通常有相应的Python库,如MySQL可以使用mysql-connector-python
或PyMySQL
,PostgreSQL可以使用psycopg2
,而SQLite可以直接使用Python标准库中的sqlite3
。每个库都有其独特的安装和使用方法,但基本的连接步骤是相似的。
一、选择并安装数据库连接库
- MySQL
要连接MySQL数据库,可以使用mysql-connector-python
库。首先,需要通过pip安装该库:
pip install mysql-connector-python
- PostgreSQL
要连接PostgreSQL数据库,可以使用psycopg2
库。通过pip安装:
pip install psycopg2
- SQLite
SQLite是Python标准库的一部分,因此无需额外安装库,直接导入即可使用。
二、配置数据库连接参数
在连接数据库之前,需要准备好数据库的连接参数。这些参数通常包括主机地址、端口号、数据库名称、用户名和密码等。
- MySQL
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
'port': 3306
}
- PostgreSQL
import psycopg2
config = {
'dbname': 'your_database',
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'port': '5432'
}
- SQLite
import sqlite3
database = 'your_database.db'
三、建立数据库连接
使用配置好的连接参数,建立与数据库的连接。
- MySQL
try:
connection = mysql.connector.connect(config)
if connection.is_connected():
print("Connected to MySQL database")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("MySQL connection is closed")
- PostgreSQL
try:
connection = psycopg2.connect(config)
cursor = connection.cursor()
cursor.execute("SELECT version();")
db_version = cursor.fetchone()
print(f"Connected to PostgreSQL database. Version: {db_version}")
except Exception as error:
print(f"Error: {error}")
finally:
if connection:
cursor.close()
connection.close()
print("PostgreSQL connection is closed")
- SQLite
try:
connection = sqlite3.connect(database)
cursor = connection.cursor()
cursor.execute("SELECT sqlite_version();")
db_version = cursor.fetchone()
print(f"Connected to SQLite database. Version: {db_version}")
except sqlite3.Error as error:
print(f"Error: {error}")
finally:
if connection:
connection.close()
print("SQLite connection is closed")
四、执行数据库操作
建立连接后,可以执行各种数据库操作,如查询、插入、更新和删除数据。
- MySQL
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
- PostgreSQL
try:
connection = psycopg2.connect(config)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except Exception as error:
print(f"Error: {error}")
finally:
if connection:
cursor.close()
connection.close()
- SQLite
try:
connection = sqlite3.connect(database)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except sqlite3.Error as error:
print(f"Error: {error}")
finally:
if connection:
connection.close()
五、处理异常和关闭连接
在与数据库交互时,处理异常情况和关闭连接是非常重要的,以确保资源的正确释放和数据的完整性。
- MySQL
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
print("MySQL connection is closed")
- PostgreSQL
try:
connection = psycopg2.connect(config)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except Exception as error:
print(f"Error: {error}")
finally:
if connection:
cursor.close()
connection.close()
print("PostgreSQL connection is closed")
- SQLite
try:
connection = sqlite3.connect(database)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table;")
rows = cursor.fetchall()
for row in rows:
print(row)
except sqlite3.Error as error:
print(f"Error: {error}")
finally:
if connection:
connection.close()
print("SQLite connection is closed")
六、使用ORM框架(如SQLAlchemy)
除了直接使用数据库连接库外,还可以使用ORM(对象关系映射)框架,如SQLAlchemy,使数据库操作更加简洁和高效。
- 安装SQLAlchemy
pip install SQLAlchemy
- 配置并连接数据库
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
DATABASE_URI = 'mysql+mysqlconnector://username:password@host:port/database'
engine = create_engine(DATABASE_URI)
Session = sessionmaker(bind=engine)
session = Session()
- 定义模型并执行操作
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(50))
email = Column(String(50))
Create tables
Base.metadata.create_all(engine)
Insert a new user
new_user = User(name='John Doe', email='john.doe@example.com')
session.add(new_user)
session.commit()
Query users
users = session.query(User).all()
for user in users:
print(user.name, user.email)
以上内容展示了如何在Python中使用不同的库连接不同类型的数据库,并执行基本的数据库操作。选择合适的库、配置连接参数、建立连接、执行操作和处理异常是连接数据库的基本步骤。使用ORM框架如SQLAlchemy可以进一步简化数据库操作,提高开发效率。
相关问答FAQs:
如何选择合适的数据库连接库?
在Python中,有多个库可以用于连接不同类型的数据库,如SQLite、MySQL、PostgreSQL等。选择合适的库取决于你的需求。例如,sqlite3
是内置库,适合小型项目;而对于大规模应用,SQLAlchemy
或PyMySQL
可能更合适。考虑数据库的类型、项目规模以及是否需要ORM支持来做出选择。
如何处理数据库连接中的异常?
在进行数据库连接时,可能会遇到各种异常,如连接超时、认证失败等。可以使用try-except语句来捕获这些异常,并进行相应的处理。确保在代码中加入适当的错误处理逻辑,例如记录错误日志或重试连接,以提高程序的稳定性。
如何优化数据库查询性能?
优化数据库查询性能可以通过多种方式实现。首先,确保数据库表的设计合理,使用索引加速查询。其次,避免在查询中使用SELECT *,而是只选择必要的字段。此外,使用连接池来管理数据库连接,可以有效减少连接时间,提高应用的响应速度。定期分析和优化SQL查询也是提升性能的关键。
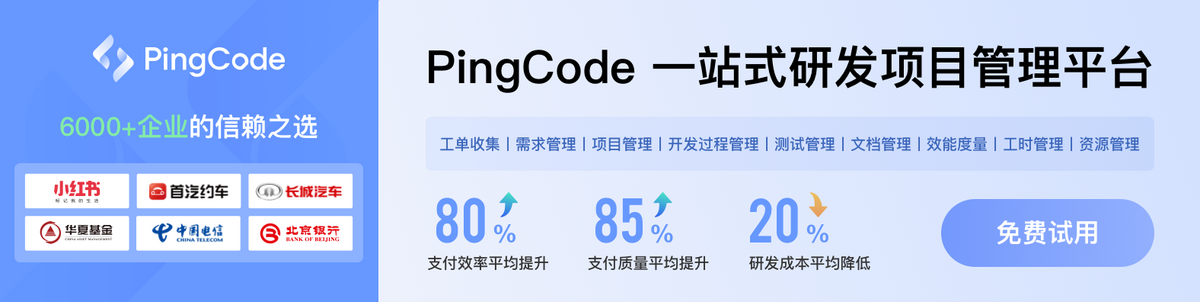