一、设置猜数个数的方法、如何实现、代码示例、提示和错误处理
在Python中设置猜数游戏的猜测次数可以通过循环和计数变量来实现。你可以使用一个计数器来跟踪用户的猜测次数,并设置一个最大猜测次数的限制。一旦用户达到最大猜测次数,游戏就会结束。下面将详细描述如何实现这一点。
一、设置猜数个数的方法
要设置猜数游戏的最大猜测次数,你可以使用一个循环来控制用户的输入,并使用一个计数器来记录用户已经猜了多少次。通过设置一个最大猜测次数,你可以确保游戏不会无限进行下去。下面是一个简单的示例:
import random
def guess_number():
number_to_guess = random.randint(1, 100)
max_attempts = 5
attempts = 0
print("Welcome to the Guess the Number game!")
print(f"You have {max_attempts} attempts to guess the number between 1 and 100.")
while attempts < max_attempts:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts.")
return
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
guess_number()
二、如何实现
- 生成随机数:首先,使用
random.randint(1, 100)
生成一个1到100之间的随机数作为目标数。 - 设置最大猜测次数:定义一个变量
max_attempts
来设置用户可以猜测的最大次数,例如5次。 - 初始化计数器:使用一个变量
attempts
来记录用户已经猜了多少次,初始值为0。 - 循环处理用户输入:使用
while
循环来处理用户的猜测,每次循环增加计数器attempts
的值。 - 判断猜测结果:在每次循环中,检查用户的输入是否与目标数相等。如果相等,则用户赢得游戏;否则,提示用户猜测的数值是高了还是低了。
- 结束游戏:如果用户在规定的次数内没有猜中目标数,则游戏结束,并显示正确的数值。
三、代码示例
这里是一个完整的代码示例,展示了如何实现一个简单的猜数游戏,并设置最大猜测次数:
import random
def guess_number():
number_to_guess = random.randint(1, 100)
max_attempts = 5
attempts = 0
print("Welcome to the Guess the Number game!")
print(f"You have {max_attempts} attempts to guess the number between 1 and 100.")
while attempts < max_attempts:
try:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts.")
return
except ValueError:
print("Invalid input. Please enter an integer.")
print(f"Sorry, you've used all {max_attempts} attempts. The number was {number_to_guess}.")
guess_number()
四、提示和错误处理
在实现猜数游戏时,考虑到用户可能会输入无效的值(例如非整数),因此添加错误处理是很重要的。上面的代码示例中使用try-except
块来捕获ValueError
,确保用户输入有效的整数。
此外,你可以根据需要调整最大猜测次数和目标数的范围。例如,如果你希望游戏更具挑战性,可以减少最大猜测次数或者增加目标数的范围。
通过这种方式,你可以轻松地设置猜数游戏的猜测次数,并为用户提供一个有趣的游戏体验。
相关问答FAQs:
如何在Python中设置猜测次数的上限?
在Python中,您可以通过在代码中定义一个变量来设置用户可猜测的次数。通常,这个变量在用户开始猜数字之前就被初始化。通过一个循环,您可以限制用户的猜测次数,并在达到上限时结束游戏。
如果用户在规定次数内猜中数字会发生什么?
如果用户在设定的猜测次数内成功猜中数字,您可以在代码中设置一个条件语句,输出恭喜消息并结束游戏。这种方式不仅让用户感到成就感,还能鼓励他们再次尝试。
如何给用户提供猜测的反馈信息?
在游戏中,您可以通过条件判断来提供反馈,比如告诉用户他们的猜测是“太高”还是“太低”。这种反馈机制能够帮助用户调整他们的下一次猜测,提高游戏的趣味性和互动性。
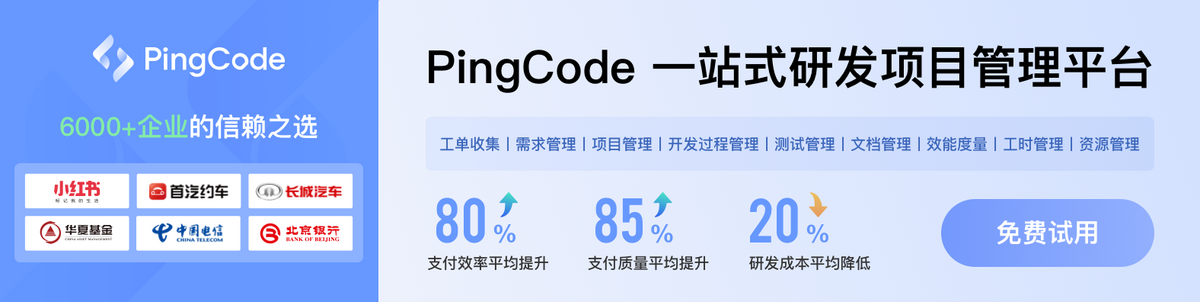