使用Python统计汉字词语词频的方法有:使用jieba库进行分词、使用collections库中的Counter进行词频统计、对统计结果进行排序和展示。其中,jieba库是一个中文分词工具,可以将文本中的汉字词语进行有效地分割,为后续的词频统计提供便利。下面我们来详细介绍具体操作方法。
一、安装和导入相关库
在开始之前,我们需要安装和导入相关的Python库。jieba库和collections库是主要的工具。
!pip install jieba
import jieba
from collections import Counter
二、读取文本数据
首先,我们需要读取包含汉字的文本数据。假设文本数据保存在一个文件中,我们可以使用以下代码读取文件内容。
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'example.txt'
text = read_text(file_path)
三、使用jieba进行分词
jieba库提供了三种分词模式:精确模式、全模式和搜索引擎模式。我们通常使用精确模式进行分词。
words = jieba.lcut(text)
jieba.lcut()函数将文本字符串分割成一个词语列表。
四、统计词频
使用collections库中的Counter类,可以快速统计词语的频率。
word_counts = Counter(words)
Counter会自动统计每个词语在列表中出现的次数,并返回一个包含词语和词频的字典。
五、排序和展示统计结果
为了更直观地展示统计结果,我们可以将词频按从高到低的顺序排列,并输出前N个高频词语。
def get_top_n_words(word_counts, n=10):
top_n_words = word_counts.most_common(n)
return top_n_words
top_words = get_top_n_words(word_counts, n=10)
for word, frequency in top_words:
print(f'{word}: {frequency}')
六、去除停用词
在中文文本处理中,停用词(如“的”、“了”等)对词频统计结果的影响较大,因此通常需要去除停用词。我们可以从网上下载一个中文停用词表并加载。
def load_stopwords(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
stopwords = set(file.read().splitlines())
return stopwords
stopwords_path = 'chinese_stopwords.txt'
stopwords = load_stopwords(stopwords_path)
words = [word for word in words if word not in stopwords]
word_counts = Counter(words)
七、完整代码示例
以下是将上述步骤整合到一起的完整代码示例。
import jieba
from collections import Counter
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def load_stopwords(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
stopwords = set(file.read().splitlines())
return stopwords
def get_top_n_words(word_counts, n=10):
top_n_words = word_counts.most_common(n)
return top_n_words
def main(file_path, stopwords_path, top_n=10):
text = read_text(file_path)
stopwords = load_stopwords(stopwords_path)
words = jieba.lcut(text)
words = [word for word in words if word not in stopwords]
word_counts = Counter(words)
top_words = get_top_n_words(word_counts, top_n)
for word, frequency in top_words:
print(f'{word}: {frequency}')
if __name__ == '__main__':
file_path = 'example.txt'
stopwords_path = 'chinese_stopwords.txt'
main(file_path, stopwords_path, top_n=10)
八、总结
通过上述步骤,我们可以使用Python高效地统计汉字词语的词频。核心步骤包括使用jieba库进行分词、使用Counter进行词频统计、去除停用词、对统计结果进行排序和展示。这种方法适用于各种中文文本处理任务,为后续的文本分析、自然语言处理等工作打下基础。
相关问答FAQs:
如何使用Python统计文本中的汉字词频?
要统计文本中的汉字词频,可以借助Python中的一些库,如jieba进行中文分词,然后使用collections库的Counter类来计算词频。代码示例如下:
import jieba
from collections import Counter
text = "这是一个用于统计汉字词语词频的示例文本。"
words = jieba.cut(text)
word_counts = Counter(words)
print(word_counts)
以上代码将输出每个词及其对应的频率。
有哪些Python库可以帮助统计汉字词频?
除了jieba外,还有其他一些库可以用于统计汉字词频,例如pkuseg、thulac和SnowNLP。这些库各有特点,pkuseg在分词准确性上表现良好,而SnowNLP则适合进行情感分析,提供更丰富的文本处理功能。
统计汉字词频时应该注意哪些问题?
在统计汉字词频时,需要注意文本的预处理,比如去除无关字符和标点符号,确保分词的准确性。此外,选择合适的分词工具和参数设置也会对最终的统计结果产生影响。考虑使用自定义词典来提高分词的准确性,特别是对于特定领域的文本。
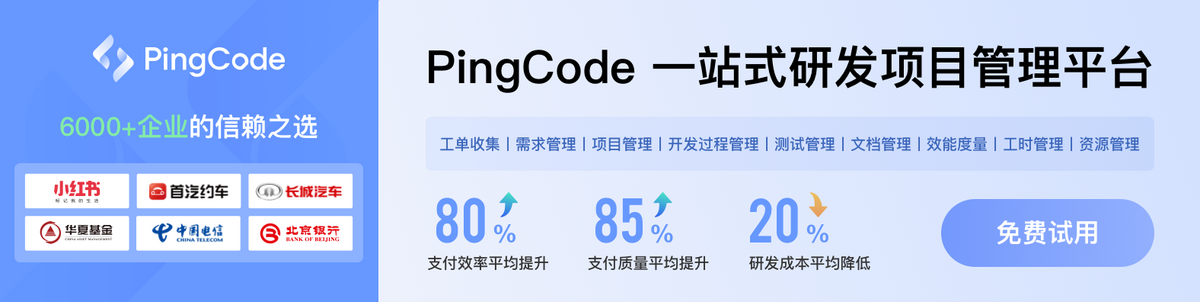