如何用Python实现邮件客户端
要用Python实现邮件客户端,核心步骤包括:设置SMTP服务器、编写发送邮件代码、处理邮件附件、实现邮件接收功能、处理邮件格式。其中,SMTP服务器的设置是关键,确保能通过适当的服务器发送邮件。
一、设置SMTP服务器
1.1、选择SMTP服务器
要发送电子邮件,首先需要选择并设置SMTP服务器。常用的SMTP服务器包括Gmail、Yahoo、Outlook等。每个服务器都有其特定的端口和安全设置。例如,Gmail的SMTP服务器为smtp.gmail.com
,端口为587(TLS)或465(SSL)。
1.2、SMTP服务器设置示例
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
smtp_server = "smtp.gmail.com"
smtp_port = 587
username = "your_email@gmail.com"
password = "your_password"
在设置SMTP服务器时,需要确保启用了安全访问,并且可能需要生成应用专用密码,特别是对于Gmail等提供双重身份验证的服务。
二、编写发送邮件代码
2.1、构建邮件内容
使用email
库可以很方便地创建邮件内容,包括主题、正文以及收件人信息。以下是一个简单的示例:
def create_email(sender, recipient, subject, body):
msg = MIMEMultipart()
msg['From'] = sender
msg['To'] = recipient
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
return msg
2.2、发送邮件
在构建好邮件内容后,可以使用SMTP
对象来发送邮件:
def send_email(smtp_server, smtp_port, username, password, msg):
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(username, password)
server.sendmail(msg['From'], msg['To'], msg.as_string())
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
2.3、综合示例
if __name__ == "__main__":
sender = "your_email@gmail.com"
recipient = "recipient_email@gmail.com"
subject = "Test Email"
body = "This is a test email sent from Python."
msg = create_email(sender, recipient, subject, body)
send_email(smtp_server, smtp_port, username, password, msg)
三、处理邮件附件
3.1、添加附件
添加附件需要使用MIMEBase
类,并对文件进行编码和打包。以下是如何添加附件的示例:
from email.mime.base import MIMEBase
from email import encoders
def attach_file(msg, file_path):
with open(file_path, 'rb') as f:
part = MIMEBase('application', 'octet-stream')
part.set_payload(f.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={file_path}')
msg.attach(part)
3.2、综合示例
将附件功能整合到之前的发送邮件代码中:
if __name__ == "__main__":
sender = "your_email@gmail.com"
recipient = "recipient_email@gmail.com"
subject = "Test Email with Attachment"
body = "This is a test email with an attachment sent from Python."
file_path = "path/to/your/file.txt"
msg = create_email(sender, recipient, subject, body)
attach_file(msg, file_path)
send_email(smtp_server, smtp_port, username, password, msg)
四、实现邮件接收功能
4.1、使用IMAP协议
接收邮件通常使用IMAP协议。可以使用imaplib
库来连接IMAP服务器并获取邮件。
4.2、连接IMAP服务器
import imaplib
import email
imap_server = "imap.gmail.com"
imap_port = 993
def connect_imap(username, password):
try:
mail = imaplib.IMAP4_SSL(imap_server, imap_port)
mail.login(username, password)
return mail
except Exception as e:
print(f"Failed to connect to IMAP server: {e}")
return None
4.3、获取邮件
def fetch_emails(mail):
mail.select("inbox")
status, messages = mail.search(None, 'ALL')
email_ids = messages[0].split()
for email_id in email_ids:
status, msg_data = mail.fetch(email_id, '(RFC822)')
raw_email = msg_data[0][1]
msg = email.message_from_bytes(raw_email)
print(f"Subject: {msg['subject']}, From: {msg['from']}")
4.4、综合示例
if __name__ == "__main__":
mail = connect_imap(username, password)
if mail:
fetch_emails(mail)
mail.logout()
五、处理邮件格式
5.1、解析邮件内容
邮件内容可能包含文本、HTML、附件等。需要递归解析邮件对象来处理不同部分。
5.2、示例代码
def parse_email(msg):
if msg.is_multipart():
for part in msg.walk():
content_type = part.get_content_type()
content_disposition = str(part.get("Content-Disposition"))
if "attachment" in content_disposition:
filename = part.get_filename()
print(f"Attachment: {filename}")
with open(filename, 'wb') as f:
f.write(part.get_payload(decode=True))
elif content_type == "text/plain":
print(f"Text: {part.get_payload(decode=True).decode('utf-8')}")
elif content_type == "text/html":
print(f"HTML: {part.get_payload(decode=True).decode('utf-8')}")
else:
print(f"Text: {msg.get_payload(decode=True).decode('utf-8')}")
5.3、综合示例
if __name__ == "__main__":
mail = connect_imap(username, password)
if mail:
mail.select("inbox")
status, messages = mail.search(None, 'ALL')
email_ids = messages[0].split()
for email_id in email_ids:
status, msg_data = mail.fetch(email_id, '(RFC822)')
raw_email = msg_data[0][1]
msg = email.message_from_bytes(raw_email)
parse_email(msg)
mail.logout()
通过以上步骤,你可以用Python实现一个功能齐全的邮件客户端,支持发送和接收邮件,并处理各种邮件格式和附件。希望这些内容对你有所帮助!
相关问答FAQs:
如何用Python发送电子邮件?
使用Python发送电子邮件可以通过内置的smtplib
库来实现。您需要设置邮件服务器的SMTP地址和端口号,创建邮件内容并使用smtplib.SMTP
类来发送邮件。例如,您可以使用Gmail的SMTP服务器,通过SSL连接进行安全传输。确保您开启了“允许不够安全的应用程序”选项,以便正常发送邮件。
实现邮件客户端需要哪些Python库?
创建一个邮件客户端通常需要几个关键的库。smtplib
用于发送邮件,email
库用于构建邮件内容(如主题、发件人、收件人和正文)。如果您还希望处理附件,可以使用MIME
类来帮助构建复杂的邮件格式。此外,imaplib
库可以用于接收和管理邮件。
如何处理邮件的附件功能?
在Python中处理邮件附件时,可以使用email.mime
模块中的MIMEBase
和MIMEText
类。首先创建一个MIMEBase
对象,设置附件的内容类型和编码方式,然后使用attach
方法将其添加到邮件中。确保在发送前正确设置邮件头,以便接收者能够正确识别附件。
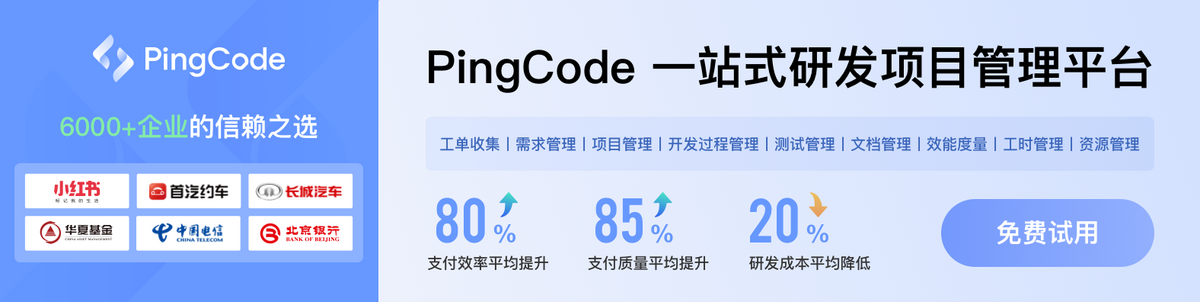