实现Python数据分析的词频统计,可以通过以下几个关键步骤来完成:数据预处理、分词处理、统计词频、结果可视化。 其中,数据预处理是基础,它决定了后续步骤的准确性与有效性。下面详细描述数据预处理的过程。
数据预处理是词频统计的第一步,涉及清洗和规范化文本数据。文本数据通常包含多余的空格、标点符号、特殊字符和大小写不一致等问题。通过对数据进行清洗,删除多余元素,统一大小写,可以提高词频统计的准确性。例如,可以使用正则表达式(Regex)来去除标点符号和特殊字符,使用Python内置的字符串操作函数来处理空格和大小写。
一、数据预处理
数据预处理是文本分析的关键步骤,直接影响后续的词频统计结果。在此步骤中,我们需要对文本数据进行清洗和规范化处理。
1、清洗数据
清洗数据是为了去除文本中的噪音数据,这些数据包括标点符号、特殊字符、多余的空格和换行符等。可以使用正则表达式(Regex)进行清洗。
例如,以下代码展示了如何使用正则表达式去除文本中的标点符号和特殊字符:
import re
def clean_text(text):
# 移除标点符号和特殊字符
text = re.sub(r'[^\w\s]', '', text)
# 移除多余的空格
text = re.sub(r'\s+', ' ', text)
return text.strip()
text = "Hello, world! This is a sample text with, punctuation."
cleaned_text = clean_text(text)
print(cleaned_text)
2、规范化处理
规范化处理主要是将文本中的字符统一为小写或大写,确保同一个词在统计时不会因为大小写不同而被分开统计。
例如,以下代码展示了如何将文本全部转换为小写:
def normalize_text(text):
return text.lower()
normalized_text = normalize_text(cleaned_text)
print(normalized_text)
二、分词处理
分词是将文本分割成一个个单词或词组的过程,是词频统计的基础。不同语言的分词方式不同,Python中常用的分词库有nltk
和jieba
。
1、使用nltk进行分词
nltk
(Natural Language Toolkit)是Python中常用的自然语言处理库,适用于英文文本的分词处理。
以下代码展示了如何使用nltk
进行英文文本的分词:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def tokenize_text(text):
return word_tokenize(text)
tokens = tokenize_text(normalized_text)
print(tokens)
2、使用jieba进行分词
jieba
是Python中常用的中文分词库,适用于中文文本的分词处理。
以下代码展示了如何使用jieba
进行中文文本的分词:
import jieba
def tokenize_text_cn(text):
return list(jieba.cut(text))
text_cn = "这是一个中文分词的示例文本。"
tokens_cn = tokenize_text_cn(text_cn)
print(tokens_cn)
三、统计词频
在完成分词之后,接下来就是统计每个词出现的频率。可以使用Python的collections.Counter
类来完成词频统计。
1、使用Counter统计词频
以下代码展示了如何使用Counter
统计词频:
from collections import Counter
def count_word_frequency(tokens):
return Counter(tokens)
word_freq = count_word_frequency(tokens)
print(word_freq)
2、统计中文词频
同样的方法也适用于中文文本的词频统计:
word_freq_cn = count_word_frequency(tokens_cn)
print(word_freq_cn)
四、结果可视化
为了更直观地展示词频统计结果,可以使用可视化工具进行展示。Python中常用的可视化库有matplotlib
和wordcloud
。
1、使用matplotlib绘制词频直方图
以下代码展示了如何使用matplotlib
绘制词频直方图:
import matplotlib.pyplot as plt
def plot_word_frequency(word_freq):
words = list(word_freq.keys())
frequencies = list(word_freq.values())
plt.figure(figsize=(10, 5))
plt.bar(words, frequencies)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Word Frequency')
plt.show()
plot_word_frequency(word_freq)
2、使用wordcloud绘制词云
词云是一种直观展示词频的图形方法,可以使用wordcloud
库生成词云。
以下代码展示了如何使用wordcloud
生成词云:
from wordcloud import WordCloud
def generate_wordcloud(word_freq):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_freq)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
generate_wordcloud(word_freq)
五、综合应用实例
最后,通过一个综合应用实例来展示如何将上述步骤结合起来,完成数据预处理、分词、词频统计和结果可视化。
1、综合代码实例
以下代码展示了一个综合应用实例,从数据预处理到结果可视化的全过程:
import re
import nltk
import jieba
import matplotlib.pyplot as plt
from collections import Counter
from wordcloud import WordCloud
def clean_text(text):
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\s+', ' ', text)
return text.strip()
def normalize_text(text):
return text.lower()
def tokenize_text(text):
return word_tokenize(text)
def tokenize_text_cn(text):
return list(jieba.cut(text))
def count_word_frequency(tokens):
return Counter(tokens)
def plot_word_frequency(word_freq):
words = list(word_freq.keys())
frequencies = list(word_freq.values())
plt.figure(figsize=(10, 5))
plt.bar(words, frequencies)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Word Frequency')
plt.show()
def generate_wordcloud(word_freq):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_freq)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
示例文本
text = "Hello, world! This is a sample text with, punctuation."
数据预处理
cleaned_text = clean_text(text)
normalized_text = normalize_text(cleaned_text)
分词处理
tokens = tokenize_text(normalized_text)
词频统计
word_freq = count_word_frequency(tokens)
结果可视化
plot_word_frequency(word_freq)
generate_wordcloud(word_freq)
中文文本示例
text_cn = "这是一个中文分词的示例文本。"
数据预处理
cleaned_text_cn = clean_text(text_cn)
分词处理
tokens_cn = tokenize_text_cn(cleaned_text_cn)
词频统计
word_freq_cn = count_word_frequency(tokens_cn)
结果可视化
plot_word_frequency(word_freq_cn)
generate_wordcloud(word_freq_cn)
通过以上步骤和代码实例,我们可以完成从数据预处理到词频统计,再到结果可视化的全过程。这些步骤在实际的文本分析和自然语言处理任务中是非常重要的,能够帮助我们更好地理解和分析文本数据。
相关问答FAQs:
如何使用Python进行词频统计?
进行词频统计的基本步骤包括文本预处理、分词、统计词频和可视化。首先,使用Python的文本处理库(如NLTK或spaCy)进行文本清洗,然后利用Counter类或pandas库来统计每个词出现的次数。最后,可以使用matplotlib或seaborn库来可视化词频分布。
在Python中有哪些库可以帮助进行词频统计?
Python提供了多个强大的库来实现词频统计。常用的有NLTK(自然语言工具包)、spaCy(用于高级自然语言处理)、collections中的Counter类(用于简单的计数),以及pandas(用于数据分析)。这些库的功能各有侧重,选择合适的工具可以大大简化词频统计的过程。
如何处理文本数据中的停用词以提高词频统计的准确性?
停用词是指在文本分析中频繁出现但对分析没有实际意义的词汇,如“的”、“是”等。为了提高词频统计的准确性,可以使用NLTK或spaCy提供的停用词列表,过滤掉这些词汇。这样可以确保统计结果更具代表性,突出重要词汇的频率。
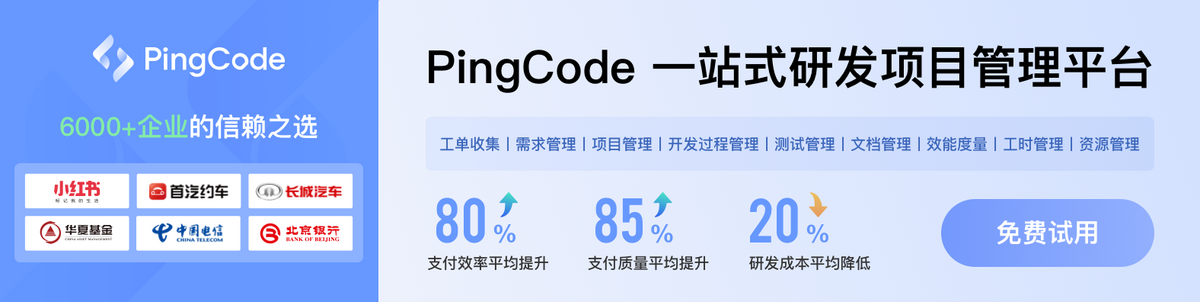