要制作一个翻译字典,您可以使用Python的字典数据结构来存储单词及其翻译。首先,创建一个包含单词和翻译的字典,然后编写函数来添加、删除和查找翻译。例如,您可以使用dict
来存储翻译对,通过各种操作(如查找和更新)来管理翻译字典。
下面我们将详细说明如何制作一个翻译字典,涵盖创建、管理和使用翻译字典的各个方面。
一、创建翻译字典
创建翻译字典的第一步是定义一个Python字典,其中键是原词,值是翻译后的词。下面是一个简单的例子:
translation_dict = {
'hello': 'hola',
'world': 'mundo',
'thank you': 'gracias',
'yes': 'sí',
'no': 'no'
}
1、初始化翻译字典
为了使翻译字典更具扩展性,我们可以使用函数来初始化字典:
def create_translation_dict():
return {
'hello': 'hola',
'world': 'mundo',
'thank you': 'gracias',
'yes': 'sí',
'no': 'no'
}
二、添加词汇到翻译字典
为了动态地添加新词汇及其翻译,我们可以定义一个函数。这个函数将接收原词和翻译词并将其添加到字典中。
def add_translation(dictionary, word, translation):
dictionary[word] = translation
1、示例用法
translation_dict = create_translation_dict()
add_translation(translation_dict, 'goodbye', 'adiós')
三、删除翻译字典中的词汇
有时我们需要从翻译字典中删除某个词汇。可以定义一个函数来执行此操作。
def remove_translation(dictionary, word):
if word in dictionary:
del dictionary[word]
else:
print(f"Word '{word}' not found in dictionary.")
1、示例用法
remove_translation(translation_dict, 'world')
四、查找翻译
为了查找某个词汇的翻译,我们可以定义一个函数。如果词汇存在,函数将返回其翻译;否则,它将返回一个提示信息。
def find_translation(dictionary, word):
return dictionary.get(word, f"Translation for '{word}' not found.")
1、示例用法
print(find_translation(translation_dict, 'hello'))
print(find_translation(translation_dict, 'unknown'))
五、批量添加翻译
在某些情况下,我们可能需要一次性添加多个翻译。我们可以定义一个函数来批量添加翻译。
def batch_add_translations(dictionary, translations):
for word, translation in translations.items():
dictionary[word] = translation
1、示例用法
new_translations = {
'cat': 'gato',
'dog': 'perro',
'bird': 'pájaro'
}
batch_add_translations(translation_dict, new_translations)
六、保存和加载翻译字典
为了持久化存储翻译字典,我们可以将其保存到文件中,并能够从文件中加载。我们可以使用json
模块来实现这一点。
1、保存翻译字典到文件
import json
def save_dictionary_to_file(dictionary, filename):
with open(filename, 'w') as file:
json.dump(dictionary, file)
2、从文件加载翻译字典
def load_dictionary_from_file(filename):
with open(filename, 'r') as file:
return json.load(file)
3、示例用法
save_dictionary_to_file(translation_dict, 'translations.json')
loaded_dict = load_dictionary_from_file('translations.json')
print(loaded_dict)
七、使用翻译字典进行翻译
最后,我们可以编写一个函数来使用翻译字典进行翻译。该函数将接收一个句子并翻译其中的每个单词。
def translate_sentence(dictionary, sentence):
words = sentence.split()
translated_words = [dictionary.get(word, word) for word in words]
return ' '.join(translated_words)
1、示例用法
sentence = 'hello world'
translated_sentence = translate_sentence(translation_dict, sentence)
print(translated_sentence)
八、增加词性和上下文信息
为了提高翻译的准确性,我们可以在字典中添加词性和上下文信息。这可以通过使用嵌套字典来实现。
def add_translation_with_context(dictionary, word, translation, part_of_speech, context):
dictionary[word] = {
'translation': translation,
'part_of_speech': part_of_speech,
'context': context
}
1、示例用法
add_translation_with_context(translation_dict, 'run', 'correr', 'verb', 'to move fast on foot')
九、支持多语言翻译
如果需要支持多语言翻译,我们可以将翻译字典扩展为多层嵌套结构,每种语言一个子字典。
def add_multilanguage_translation(dictionary, word, translations):
dictionary[word] = translations
1、示例用法
multilang_translations = {
'spanish': 'hola',
'french': 'bonjour',
'german': 'hallo'
}
add_multilanguage_translation(translation_dict, 'hello', multilang_translations)
十、总结
制作一个翻译字典需要考虑各种操作,例如添加、删除、查找和批量处理翻译。通过使用Python的字典数据结构和函数,我们可以轻松实现这些功能。进一步的扩展可以包括保存和加载字典、增加上下文信息以及支持多语言翻译。通过这些方法,我们可以创建一个功能强大且灵活的翻译字典系统。
相关问答FAQs:
如何使用Python创建一个简单的翻译字典?
要创建一个翻译字典,可以使用Python的字典(dict)数据结构。首先,定义一个字典,其中键是要翻译的单词,值是对应的翻译。比如:
translation_dict = {
'hello': '你好',
'world': '世界',
'python': '蟒蛇'
}
通过这种方式,你可以轻松地查找和获取翻译。只需通过键访问值即可。
如何将翻译字典保存到文件中?
为了持久化翻译字典,可以使用Python的json
模块将字典保存为JSON文件。使用json.dump()
方法可以将字典写入文件。示例代码如下:
import json
with open('translation_dict.json', 'w', encoding='utf-8') as f:
json.dump(translation_dict, f, ensure_ascii=False)
这样,你的翻译字典就会被保存为一个名为translation_dict.json
的文件。
如何实现翻译字典的动态更新?
为了实现动态更新,可以定义一个函数来添加新单词及其翻译到字典中。例如:
def add_translation(word, translation):
translation_dict[word] = translation
调用这个函数并传入新单词和其翻译,可以方便地扩展翻译字典。确保在更新后保存字典到文件,以免丢失更改。
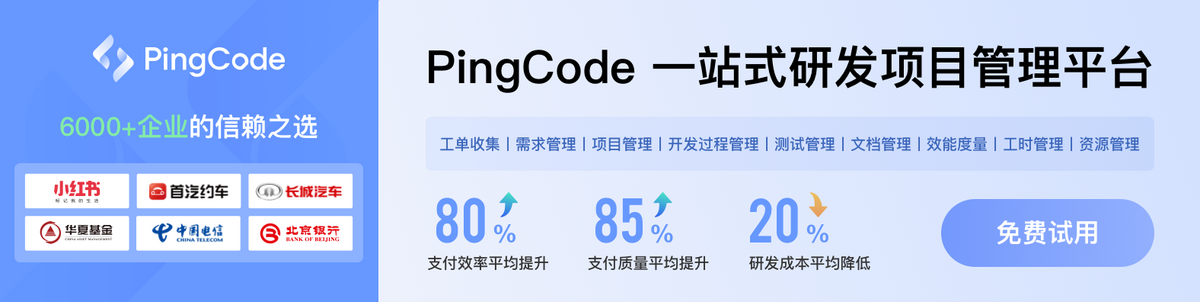