Python 处理文件夹数据的方法包括:os 模块、shutil 模块、pathlib 模块。
os 模块是 Python 标准库中的一个模块,主要用于操作文件和目录。通过 os 模块,你可以读取目录内容、创建和删除目录等。shutil 模块提供了更高级的文件操作,包括复制、移动和删除文件或目录。pathlib 模块提供了一个面向对象的路径操作方法,简化了路径管理和文件处理。
一、os 模块
os 模块是 Python 中用于与操作系统进行交互的标准模块。它提供了一些用于处理文件和目录的函数。
1、读取目录内容
使用 os.listdir()
函数可以获取指定目录中的所有文件和目录的列表。
import os
获取当前目录
current_directory = os.getcwd()
print("当前目录:", current_directory)
获取指定目录中的所有文件和目录
directory_content = os.listdir(current_directory)
print("目录内容:", directory_content)
2、创建和删除目录
使用 os.mkdir()
和 os.rmdir()
函数可以创建和删除目录。
import os
创建目录
new_directory = "new_folder"
if not os.path.exists(new_directory):
os.mkdir(new_directory)
print(f"目录 '{new_directory}' 创建成功")
else:
print(f"目录 '{new_directory}' 已经存在")
删除目录
if os.path.exists(new_directory):
os.rmdir(new_directory)
print(f"目录 '{new_directory}' 删除成功")
else:
print(f"目录 '{new_directory}' 不存在")
3、文件路径操作
使用 os.path
模块可以进行文件路径的操作,如拼接路径、检查路径是否存在等。
import os
拼接路径
directory = "example_folder"
filename = "example_file.txt"
file_path = os.path.join(directory, filename)
print("文件路径:", file_path)
检查路径是否存在
if os.path.exists(file_path):
print(f"路径 '{file_path}' 存在")
else:
print(f"路径 '{file_path}' 不存在")
二、shutil 模块
shutil 模块提供了一些高级的文件操作函数,如复制、移动和删除文件或目录。
1、复制文件和目录
使用 shutil.copy()
和 shutil.copytree()
函数可以复制文件和目录。
import shutil
复制文件
source_file = "source_file.txt"
destination_file = "destination_file.txt"
shutil.copy(source_file, destination_file)
print(f"文件 '{source_file}' 复制到 '{destination_file}'")
复制目录
source_directory = "source_folder"
destination_directory = "destination_folder"
shutil.copytree(source_directory, destination_directory)
print(f"目录 '{source_directory}' 复制到 '{destination_directory}'")
2、移动文件和目录
使用 shutil.move()
函数可以移动文件和目录。
import shutil
移动文件
source_file = "source_file.txt"
destination_directory = "destination_folder"
shutil.move(source_file, destination_directory)
print(f"文件 '{source_file}' 移动到 '{destination_directory}'")
移动目录
source_directory = "source_folder"
destination_directory = "destination_folder"
shutil.move(source_directory, destination_directory)
print(f"目录 '{source_directory}' 移动到 '{destination_directory}'")
3、删除文件和目录
使用 shutil.rmtree()
函数可以删除目录及其内容。
import shutil
删除目录及其内容
directory_to_delete = "directory_to_delete"
shutil.rmtree(directory_to_delete)
print(f"目录 '{directory_to_delete}' 及其内容已删除")
三、pathlib 模块
pathlib 模块提供了一个面向对象的路径操作方法,简化了路径管理和文件处理。
1、创建 Path 对象
使用 Path
类可以创建路径对象,并进行各种路径操作。
from pathlib import Path
创建 Path 对象
current_directory = Path.cwd()
print("当前目录:", current_directory)
创建相对路径对象
relative_path = Path("example_folder/example_file.txt")
print("相对路径:", relative_path)
创建绝对路径对象
absolute_path = current_directory / "example_folder" / "example_file.txt"
print("绝对路径:", absolute_path)
2、读取目录内容
使用 Path.iterdir()
方法可以遍历目录内容。
from pathlib import Path
获取当前目录
current_directory = Path.cwd()
遍历目录内容
for item in current_directory.iterdir():
print(item)
3、创建和删除目录
使用 Path.mkdir()
和 Path.rmdir()
方法可以创建和删除目录。
from pathlib import Path
创建目录
new_directory = Path("new_folder")
if not new_directory.exists():
new_directory.mkdir()
print(f"目录 '{new_directory}' 创建成功")
else:
print(f"目录 '{new_directory}' 已经存在")
删除目录
if new_directory.exists():
new_directory.rmdir()
print(f"目录 '{new_directory}' 删除成功")
else:
print(f"目录 '{new_directory}' 不存在")
4、文件路径操作
使用 Path
对象可以进行各种文件路径操作,如拼接路径、检查路径是否存在等。
from pathlib import Path
拼接路径
directory = Path("example_folder")
filename = "example_file.txt"
file_path = directory / filename
print("文件路径:", file_path)
检查路径是否存在
if file_path.exists():
print(f"路径 '{file_path}' 存在")
else:
print(f"路径 '{file_path}' 不存在")
四、综合应用
通过结合 os 模块、shutil 模块和 pathlib 模块,可以实现更复杂的文件夹数据处理操作。
1、递归读取目录内容
递归读取目录内容可以遍历所有子目录及其文件。
import os
def list_directory_contents(directory):
for root, dirs, files in os.walk(directory):
for name in dirs:
print("目录:", os.path.join(root, name))
for name in files:
print("文件:", os.path.join(root, name))
递归读取当前目录内容
current_directory = os.getcwd()
list_directory_contents(current_directory)
2、文件批量操作
通过读取目录内容,可以对目录中的所有文件进行批量操作。
import os
import shutil
def batch_rename_files(directory, old_extension, new_extension):
for root, dirs, files in os.walk(directory):
for filename in files:
if filename.endswith(old_extension):
old_file_path = os.path.join(root, filename)
new_file_path = os.path.join(root, filename.replace(old_extension, new_extension))
os.rename(old_file_path, new_file_path)
print(f"文件 '{old_file_path}' 重命名为 '{new_file_path}'")
批量重命名当前目录中的所有 .txt 文件为 .md 文件
current_directory = os.getcwd()
batch_rename_files(current_directory, ".txt", ".md")
3、文件夹备份与恢复
通过复制目录,可以实现文件夹的备份与恢复。
import shutil
from pathlib import Path
def backup_directory(source_directory, backup_directory):
source_path = Path(source_directory)
backup_path = Path(backup_directory)
if source_path.exists() and source_path.is_dir():
if backup_path.exists():
shutil.rmtree(backup_path)
shutil.copytree(source_path, backup_path)
print(f"目录 '{source_directory}' 备份到 '{backup_directory}'")
else:
print(f"目录 '{source_directory}' 不存在")
def restore_directory(backup_directory, restore_directory):
backup_path = Path(backup_directory)
restore_path = Path(restore_directory)
if backup_path.exists() and backup_path.is_dir():
if restore_path.exists():
shutil.rmtree(restore_path)
shutil.copytree(backup_path, restore_path)
print(f"目录 '{backup_directory}' 恢复到 '{restore_directory}'")
else:
print(f"目录 '{backup_directory}' 不存在")
备份和恢复目录
source_directory = "example_folder"
backup_directory = "backup_folder"
restore_directory = "restored_folder"
backup_directory(source_directory, backup_directory)
restore_directory(backup_directory, restore_directory)
通过上述的介绍和代码示例,我们可以看到 Python 提供了多种模块和方法用于处理文件夹数据。os 模块适用于基本的文件和目录操作,shutil 模块提供了高级的文件操作功能,pathlib 模块提供了面向对象的路径操作方法。结合这些模块,可以实现更复杂的文件夹数据处理任务,如递归读取目录内容、文件批量操作、文件夹备份与恢复等。希望这些内容对你处理文件夹数据有所帮助。
相关问答FAQs:
如何使用Python遍历文件夹中的所有文件?
使用Python的os
模块可以轻松遍历文件夹。可以使用os.listdir()
函数列出目录中的所有文件和子文件夹,结合os.path.isfile()
和os.path.isdir()
函数可以判断这些项目是文件还是文件夹。这样,您可以实现对文件夹的递归遍历,处理所需的文件。
在Python中如何读取文本文件的内容?
Python提供了简单的文件操作方法来读取文本文件的内容。使用内置的open()
函数打开文件,可以指定读取模式(如'r'
表示读取)。可以使用read()
方法读取整个文件内容,或者使用readline()
和readlines()
方法逐行读取。读取完成后,记得关闭文件,以释放系统资源。
如何在Python中写入和更新文件?
写入和更新文件同样简单。通过open()
函数以写入模式(如'w'
或'a'
)打开文件,您可以使用write()
方法将字符串写入文件。选择'w'
模式会覆盖文件内容,而'a'
模式会将新内容追加到文件末尾。在写入完成后,确保使用close()
方法关闭文件,以确保数据正确保存。
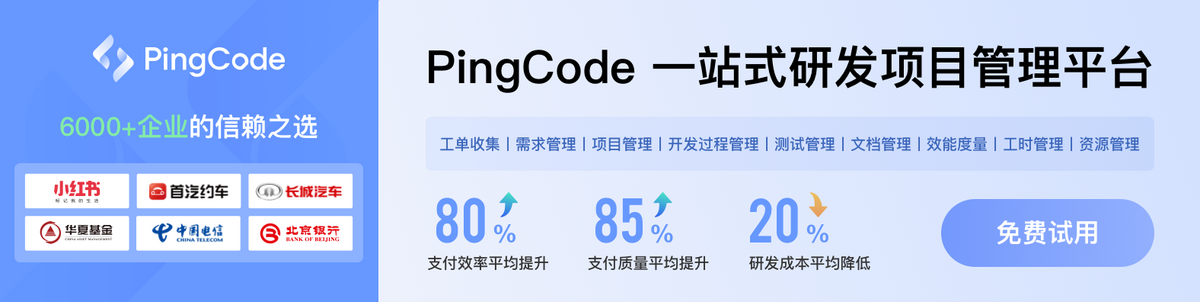