Python 控制函数执行与不执行的方法有:使用条件语句、函数装饰器、全局变量/状态标志、异常处理。 其中,使用条件语句是最常见的方法,可以根据特定条件来控制函数是否执行。以下是对条件语句控制函数执行的详细描述。
一、条件语句控制函数执行
条件语句是控制函数执行与否最直观的方法。通过if
语句,可以根据特定的条件决定是否调用某个函数。例如:
def my_function():
print("Function executed")
should_execute = True
if should_execute:
my_function()
在这个例子中,my_function
函数只有在should_execute
为True
时才会被执行。条件语句灵活且易于理解,非常适合控制函数的执行。
二、使用装饰器控制函数执行
装饰器是一种高级的Python功能,可以在不修改函数本身的情况下扩展其行为。通过装饰器,可以控制函数在特定条件下执行或不执行。例如:
def conditional_decorator(condition):
def decorator(func):
def wrapper(*args, kwargs):
if condition():
return func(*args, kwargs)
else:
print("Function not executed due to condition")
return wrapper
return decorator
def check_condition():
return True
@conditional_decorator(check_condition)
def my_function():
print("Function executed")
my_function()
在这个例子中,conditional_decorator
装饰器根据check_condition
函数的返回值决定是否执行my_function
。
三、全局变量/状态标志控制函数执行
通过使用全局变量或状态标志,可以在程序运行过程中动态控制函数的执行。例如:
execution_flag = False
def set_execution_flag(value):
global execution_flag
execution_flag = value
def my_function():
if execution_flag:
print("Function executed")
else:
print("Function not executed")
Set the flag to True to allow execution
set_execution_flag(True)
my_function()
Set the flag to False to prevent execution
set_execution_flag(False)
my_function()
在这个例子中,通过execution_flag
变量控制my_function
的执行与否。
四、异常处理控制函数执行
异常处理是一种在特定情况下控制函数执行的方法。可以在函数内部使用try...except
块来捕捉特定异常,从而控制函数是否继续执行。例如:
def my_function():
try:
# Some code that might raise an exception
result = 10 / 0
print("Function executed")
except ZeroDivisionError:
print("Function not executed due to ZeroDivisionError")
my_function()
在这个例子中,my_function
尝试执行一个可能引发异常的操作,如果捕捉到ZeroDivisionError
,则函数不会继续执行。
五、使用回调函数控制执行
回调函数是一种将函数作为参数传递并在特定条件下执行的方法。例如:
def my_function(callback):
if callback():
print("Function executed")
else:
print("Function not executed")
def check_condition():
return True
my_function(check_condition)
在这个例子中,my_function
根据check_condition
回调函数的返回值决定是否执行。
六、基于时间的控制执行
有时需要在特定时间或间隔执行函数,可以使用定时器或调度器来控制。例如,使用threading
模块的Timer
类:
import threading
def my_function():
print("Function executed")
Execute my_function after 5 seconds
timer = threading.Timer(5.0, my_function)
timer.start()
在这个例子中,my_function
将在5秒后执行。
七、基于事件的控制执行
在事件驱动的编程中,可以使用事件来控制函数的执行。例如,使用threading
模块的Event
类:
import threading
def my_function(event):
event.wait() # Wait until the event is set
print("Function executed")
event = threading.Event()
Start a thread that waits for the event
thread = threading.Thread(target=my_function, args=(event,))
thread.start()
Set the event to allow the function to execute
event.set()
在这个例子中,my_function
只有在event
被设置后才会执行。
八、使用Mock库控制执行(测试环境)
在测试环境中,可以使用unittest.mock
库来控制函数的执行。例如:
from unittest.mock import patch
def my_function():
print("Function executed")
Mock my_function to prevent it from executing
with patch('__main__.my_function', return_value=None):
my_function() # This will not print anything
在这个例子中,通过patch
函数,可以在测试期间控制my_function
的执行。
通过以上方法,您可以灵活地控制Python函数的执行和不执行,以满足不同的编程需求。不同的方法适用于不同的场景,选择最适合您当前需求的方法来实现功能。
相关问答FAQs:
1. 如何在Python中使用条件语句控制函数的执行?
在Python中,可以使用条件语句(如if
语句)来决定是否执行某个函数。例如,可以根据某个变量的值或者用户输入的条件来判断是否调用该函数。以下是一个简单的示例:
def my_function():
print("函数被执行")
execute_function = True # 设定一个条件
if execute_function:
my_function() # 条件为真时执行函数
2. 是否可以使用装饰器来控制函数的执行?
是的,装饰器是Python中一个强大的功能,可以用于控制函数的执行。通过定义一个装饰器,可以在函数调用之前或之后添加一些逻辑,以决定是否执行被装饰的函数。例如:
def control_execution(func):
def wrapper(*args, **kwargs):
if some_condition: # 根据某个条件决定是否执行
return func(*args, **kwargs)
else:
print("函数未被执行")
return wrapper
@control_execution
def my_function():
print("函数被执行")
3. Python中是否可以通过异常处理来控制函数的执行?
异常处理可以用来控制函数的执行,特别是在需要捕获特定错误时。例如,如果函数执行可能会导致某种异常,可以通过try...except
语句来决定是否继续执行该函数。如下所示:
def risky_function():
# 可能抛出异常的代码
raise ValueError("发生了一个错误")
try:
risky_function() # 尝试执行该函数
except ValueError:
print("函数未被执行,捕获到错误")
通过这些方式,可以灵活地控制Python中函数的执行与否,满足不同的业务需求。
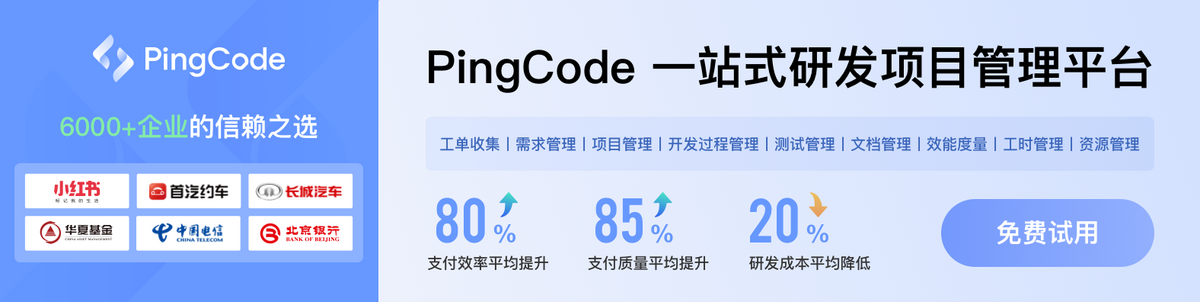