使用Python复制文件的几种方法包括使用shutil模块、使用os模块、以及手动读取和写入文件内容,这些方法各有优缺点,推荐使用shutil模块,因为它简单且功能强大。
使用shutil模块是最常见和便捷的方法,因为它提供了高层次的文件操作功能,能够处理文件的复制、移动、删除等操作。下面我们详细描述一下如何使用shutil模块来复制文件。
一、使用shutil模块
shutil
模块是Python标准库中的一个模块,专门用于执行高级的文件操作。它提供了一些简单的函数来进行文件复制操作,例如shutil.copy
、shutil.copy2
、shutil.copyfile
等等。
1、shutil.copy
shutil.copy
函数会复制源文件到目标位置,并且保留文件的权限模式,但不会保留文件的元数据(例如文件的创建时间和修改时间)。
示例代码:
import shutil
source = 'source_file.txt'
destination = 'destination_file.txt'
shutil.copy(source, destination)
2、shutil.copy2
shutil.copy2
函数在执行文件复制的同时,还会保留文件的元数据(创建时间、修改时间等),因此它是shutil.copy
的增强版。
示例代码:
import shutil
source = 'source_file.txt'
destination = 'destination_file.txt'
shutil.copy2(source, destination)
3、shutil.copyfile
shutil.copyfile
函数只复制文件内容,不保留文件的权限模式和元数据。
示例代码:
import shutil
source = 'source_file.txt'
destination = 'destination_file.txt'
shutil.copyfile(source, destination)
二、使用os模块
虽然os
模块并不是专门用于文件复制的,但我们可以通过结合文件的读写操作来实现文件的复制。
1、手动读取和写入文件
我们可以手动打开源文件进行读取,并将读取到的内容写入目标文件,从而实现文件的复制。这种方法灵活性较高,但相对繁琐。
示例代码:
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
with open(source, 'rb') as src_file:
with open(destination, 'wb') as dest_file:
dest_file.write(src_file.read())
2、使用os.system
我们也可以通过os.system
函数调用操作系统的命令来实现文件复制,例如在Linux或MacOS上使用cp
命令,在Windows上使用copy
命令。
示例代码(Linux/MacOS):
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
os.system(f'cp {source} {destination}')
示例代码(Windows):
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
os.system(f'copy {source} {destination}')
三、手动读取和写入文件内容
这种方法是最基础的文件复制方法,适用于对文件内容需要进行特殊处理的场景。
1、逐行读取和写入
我们可以逐行读取源文件的内容,并将每一行写入目标文件。这种方法适用于文件较大,逐行处理可以节省内存。
示例代码:
source = 'source_file.txt'
destination = 'destination_file.txt'
with open(source, 'rb') as src_file:
with open(destination, 'wb') as dest_file:
for line in src_file:
dest_file.write(line)
四、总结
通过以上几种方法,我们可以灵活地选择适合自己的文件复制方法。如果只是简单地复制文件,推荐使用shutil
模块,因为它提供了高层次的接口,使用起来非常方便。如果对文件复制有特殊要求,可以考虑使用os
模块或者手动读取和写入文件内容的方法。
总之,在Python中复制文件的方法多种多样,选择合适的方法可以提高工作效率。
相关问答FAQs:
如何在Python中复制文件?
在Python中,可以使用内置的shutil
模块来轻松复制文件。具体方法是使用shutil.copy()
函数,该函数接受源文件路径和目标文件路径作为参数。以下是一个示例代码:
import shutil
shutil.copy('source_file.txt', 'destination_file.txt')
这段代码会将source_file.txt
复制到destination_file.txt
,如果目标文件已存在,则会被覆盖。
是否可以在复制文件时更改文件名?
是的,复制文件时可以随意更改目标文件的名称。在调用shutil.copy()
时,只需指定一个新的文件名作为目标路径。例如,如果您希望将文件复制到同一目录下并重命名为new_file.txt
,可以这样做:
shutil.copy('source_file.txt', 'new_file.txt')
这样,source_file.txt
的副本将被创建为new_file.txt
。
在Python中如何复制文件夹及其内容?
如果需要复制整个文件夹及其内容,可以使用shutil.copytree()
函数。此函数会将源文件夹及其内部所有文件和子文件夹复制到目标位置。例如:
import shutil
shutil.copytree('source_folder', 'destination_folder')
这段代码会将source_folder
及其所有内容复制到destination_folder
,如果目标文件夹已存在,则会引发错误,因此在复制之前请确保目标路径是新的或已删除。
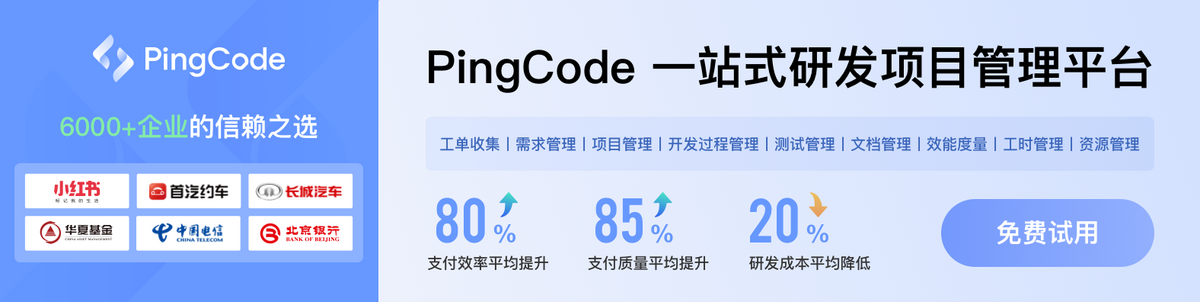