在Python中创建空集合,可以使用set()
函数。 这是创建空集合的唯一方法,因为使用空的大括号 {}
会创建一个空字典,而不是集合。在Python中,集合是一种无序的、可变的、且不允许重复元素的数据类型。下面我们将详细介绍如何在Python中创建空集合以及与集合相关的其他操作。
一、使用set()函数创建空集合
在Python中创建空集合的正确方法是使用 set()
函数:
empty_set = set()
print(empty_set) # 输出: set()
使用 set()
函数创建一个空集合是非常重要的,因为 {}
创建的是一个空字典,而不是集合。
二、集合的基本操作
在我们创建了一个空集合之后,可以向集合中添加元素、删除元素以及进行其他操作。下面我们将介绍一些常用的集合操作。
1、添加元素
可以使用 add()
方法向集合中添加元素:
empty_set.add(1)
empty_set.add(2)
empty_set.add(3)
print(empty_set) # 输出: {1, 2, 3}
2、删除元素
可以使用 remove()
方法从集合中删除元素,如果元素不存在则会引发 KeyError
异常:
empty_set.remove(2)
print(empty_set) # 输出: {1, 3}
为了避免 KeyError
,可以使用 discard()
方法,它在元素不存在时不会引发异常:
empty_set.discard(4) # 不会引发异常
print(empty_set) # 输出: {1, 3}
3、检查元素
可以使用 in
关键字检查元素是否存在于集合中:
print(1 in empty_set) # 输出: True
print(4 in empty_set) # 输出: False
三、集合操作的实例
为了更好地理解集合的使用,我们可以通过一些实例来展示集合的常见操作。
1、联合(Union)
联合操作可以使用 union()
方法或 |
运算符:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set) # 输出: {1, 2, 3, 4, 5}
或者使用 | 运算符
union_set = set1 | set2
print(union_set) # 输出: {1, 2, 3, 4, 5}
2、交集(Intersection)
交集操作可以使用 intersection()
方法或 &
运算符:
intersection_set = set1.intersection(set2)
print(intersection_set) # 输出: {3}
或者使用 & 运算符
intersection_set = set1 & set2
print(intersection_set) # 输出: {3}
3、差集(Difference)
差集操作可以使用 difference()
方法或 -
运算符:
difference_set = set1.difference(set2)
print(difference_set) # 输出: {1, 2}
或者使用 - 运算符
difference_set = set1 - set2
print(difference_set) # 输出: {1, 2}
4、对称差(Symmetric Difference)
对称差操作可以使用 symmetric_difference()
方法或 ^
运算符:
symmetric_difference_set = set1.symmetric_difference(set2)
print(symmetric_difference_set) # 输出: {1, 2, 4, 5}
或者使用 ^ 运算符
symmetric_difference_set = set1 ^ set2
print(symmetric_difference_set) # 输出: {1, 2, 4, 5}
四、集合的其他特性和方法
除了上述基本操作,集合还有一些其他有用的方法和特性。
1、集合的长度
可以使用 len()
函数获取集合的长度:
print(len(empty_set)) # 输出: 2
2、清空集合
可以使用 clear()
方法清空集合中的所有元素:
empty_set.clear()
print(empty_set) # 输出: set()
3、遍历集合
可以使用 for
循环遍历集合中的元素:
for element in set1:
print(element)
4、复制集合
可以使用 copy()
方法复制集合:
set_copy = set1.copy()
print(set_copy) # 输出: {1, 2, 3}
五、集合的应用场景
集合在实际应用中有很多场景,下面列举一些常见的应用场景:
1、数据去重
集合最大的特点之一是不允许重复元素,因此可以用来去除重复数据:
data = [1, 2, 2, 3, 4, 4, 5]
unique_data = list(set(data))
print(unique_data) # 输出: [1, 2, 3, 4, 5]
2、集合运算
集合运算在处理关系数据时非常有用,例如计算两个集合的交集、并集、差集等:
students_in_class1 = {"Alice", "Bob", "Charlie"}
students_in_class2 = {"Bob", "David", "Edward"}
找到两个班级的所有学生(并集)
all_students = students_in_class1 | students_in_class2
print(all_students) # 输出: {'Alice', 'Bob', 'Charlie', 'David', 'Edward'}
找到两个班级都在的学生(交集)
common_students = students_in_class1 & students_in_class2
print(common_students) # 输出: {'Bob'}
找到只在第一个班级的学生(差集)
unique_to_class1 = students_in_class1 - students_in_class2
print(unique_to_class1) # 输出: {'Alice', 'Charlie'}
3、快速查找
由于集合的实现基于哈希表,因此查找操作非常快,可以用于需要快速查找的场景:
prime_numbers = {2, 3, 5, 7, 11, 13, 17, 19, 23, 29}
检查一个数是否是质数
def is_prime(number):
return number in prime_numbers
print(is_prime(11)) # 输出: True
print(is_prime(12)) # 输出: False
六、总结
在Python中,集合是一种非常有用的数据结构,特别适合用于需要存储唯一元素的场景。通过本文的介绍,我们详细讲解了如何创建空集合、集合的基本操作、集合的应用场景等内容。希望这些内容能够帮助你更好地理解和使用集合。
集合在数据处理、去重、关系运算、快速查找等方面都有广泛的应用。掌握集合的使用,可以让你的代码更加简洁、高效。希望本文对你有所帮助,如果有任何问题或建议,欢迎交流讨论。
相关问答FAQs:
如何在Python中创建一个空集合?
在Python中,创建一个空集合可以使用 set()
函数。与其他数据类型不同,空集合不能使用花括号 {}
来创建,因为这会被解释为一个空字典。示例代码如下:
empty_set = set()
空集合与空字典有什么区别?
空集合和空字典在Python中是不同的数据结构。空集合使用 set()
创建,表示一个无序的、不重复的元素集合。而空字典使用 {}
或 dict()
创建,表示一个键值对的集合。空集合不能包含重复元素,而空字典可以有重复的值。
在Python中向空集合添加元素的方式有哪些?
可以使用 add()
方法向集合中添加单个元素,或者使用 update()
方法向集合中添加多个元素。以下是示例:
my_set = set()
my_set.add(1) # 添加单个元素
my_set.update([2, 3]) # 添加多个元素
通过这些方法,可以灵活地管理集合中的元素。
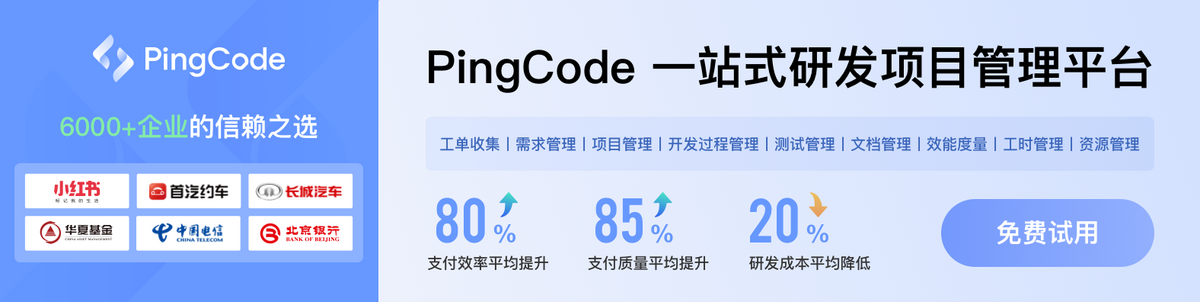