Python 如何画五角星
要在Python中画五角星,可以使用turtle库、matplotlib库、PIL库等。这些库提供了不同的工具和方法来绘制图形、实现简单易用的绘图功能。 其中,turtle库是Python标准库的一部分,特别适合初学者。matplotlib库则提供了更高级的绘图功能,适合需要更复杂图形的用户。而PIL库则可以处理图像并绘制图形。下面详细介绍如何使用turtle库绘制五角星。
一、turtle库绘制五角星
turtle库是一个非常易于使用的绘图工具,特别适合教育和初学者。它基于海龟绘图的概念,通过控制“海龟”的移动来绘制图形。
1、安装和导入turtle库
turtle库是Python标准库的一部分,不需要额外安装。可以直接导入:
import turtle
2、设置绘图窗口和海龟
首先,需要设置绘图窗口和海龟的初始状态:
# 创建一个绘图窗口
screen = turtle.Screen()
screen.title("五角星绘制")
创建一个海龟对象
star = turtle.Turtle()
star.speed(1) # 设置海龟的绘图速度
3、绘制五角星
绘制五角星的关键在于五角星的顶点之间的角度。五角星的每个角度是144度。
# 绘制五角星
for _ in range(5):
star.forward(100) # 向前移动100像素
star.right(144) # 右转144度
完成绘图
turtle.done()
4、完整代码
将以上步骤结合起来,得到完整的绘制五角星的代码如下:
import turtle
创建一个绘图窗口
screen = turtle.Screen()
screen.title("五角星绘制")
创建一个海龟对象
star = turtle.Turtle()
star.speed(1) # 设置海龟的绘图速度
绘制五角星
for _ in range(5):
star.forward(100) # 向前移动100像素
star.right(144) # 右转144度
完成绘图
turtle.done()
二、matplotlib库绘制五角星
matplotlib是一个强大的绘图库,适用于需要更复杂和专业的绘图需求。虽然它主要用于绘制数据图表,但也可以用来绘制基本图形。
1、安装和导入matplotlib库
首先,需要安装matplotlib库:
pip install matplotlib
然后导入必要的模块:
import matplotlib.pyplot as plt
import numpy as np
2、定义五角星顶点
通过数学计算,可以定义五角星的顶点坐标:
# 定义五角星顶点坐标
angles = np.linspace(0, 2 * np.pi, 6)[:-1] # 生成五角星的五个顶点角度
radius = 1 # 半径
计算顶点的坐标
x = radius * np.cos(angles)
y = radius * np.sin(angles)
交叉连接顶点
x = np.array([x[0], x[2], x[4], x[1], x[3], x[0]])
y = np.array([y[0], y[2], y[4], y[1], y[3], y[0]])
3、绘制五角星
使用matplotlib绘制五角星:
plt.plot(x, y, 'b-') # 绘制蓝色线条
plt.fill(x, y, 'cyan') # 填充颜色
plt.axis('equal') # 保持坐标轴比例
plt.title('五角星')
plt.show()
4、完整代码
将以上步骤结合起来,得到完整的绘制五角星的代码如下:
import matplotlib.pyplot as plt
import numpy as np
定义五角星顶点坐标
angles = np.linspace(0, 2 * np.pi, 6)[:-1]
radius = 1
x = radius * np.cos(angles)
y = radius * np.sin(angles)
x = np.array([x[0], x[2], x[4], x[1], x[3], x[0]])
y = np.array([y[0], y[2], y[4], y[1], y[3], y[0]])
plt.plot(x, y, 'b-')
plt.fill(x, y, 'cyan')
plt.axis('equal')
plt.title('五角星')
plt.show()
三、PIL库绘制五角星
PIL(Python Imaging Library)是一个强大的图像处理库,可以用来创建和操作图像。在PIL库中,可以使用ImageDraw模块来绘制基本图形。
1、安装和导入PIL库
首先,需要安装PIL库(Pillow是PIL的升级版):
pip install pillow
然后导入必要的模块:
from PIL import Image, ImageDraw
2、创建图像和绘图对象
创建一个空白图像,并获得绘图对象:
# 创建空白图像
image = Image.new("RGB", (200, 200), "white")
创建绘图对象
draw = ImageDraw.Draw(image)
3、定义五角星顶点
定义五角星的顶点坐标:
# 定义五角星顶点坐标
angles = np.linspace(0, 2 * np.pi, 6)[:-1]
radius = 80
center = (100, 100)
计算顶点的坐标
vertices = [(center[0] + radius * np.cos(angle), center[1] + radius * np.sin(angle)) for angle in angles]
vertices = [vertices[0], vertices[2], vertices[4], vertices[1], vertices[3], vertices[0]]
4、绘制五角星
使用ImageDraw对象绘制五角星:
draw.line(vertices, fill="blue", width=2)
5、保存和显示图像
保存和显示图像:
image.show()
image.save("five_point_star.png")
6、完整代码
将以上步骤结合起来,得到完整的绘制五角星的代码如下:
from PIL import Image, ImageDraw
import numpy as np
创建空白图像
image = Image.new("RGB", (200, 200), "white")
创建绘图对象
draw = ImageDraw.Draw(image)
定义五角星顶点坐标
angles = np.linspace(0, 2 * np.pi, 6)[:-1]
radius = 80
center = (100, 100)
vertices = [(center[0] + radius * np.cos(angle), center[1] + radius * np.sin(angle)) for angle in angles]
vertices = [vertices[0], vertices[2], vertices[4], vertices[1], vertices[3], vertices[0]]
绘制五角星
draw.line(vertices, fill="blue", width=2)
保存和显示图像
image.show()
image.save("five_point_star.png")
四、总结
通过以上介绍,我们可以使用不同的Python库来绘制五角星,包括turtle库、matplotlib库和PIL库。turtle库适合初学者,提供简单易用的绘图功能;matplotlib库适合需要更复杂图形的用户,提供更高级的绘图功能;PIL库则适合处理图像并绘制图形。 选择合适的工具,可以帮助我们更好地完成绘图任务。
相关问答FAQs:
如何在Python中绘制五角星?
在Python中,您可以使用turtle
库来绘制五角星。首先,确保已安装turtle
库,接着可以通过简单的代码来创建五角星图形。以下是一个示例代码:
import turtle
def draw_star(size):
for i in range(5):
turtle.forward(size)
turtle.right(144)
turtle.speed(1)
draw_star(100)
turtle.done()
在这个代码中,draw_star
函数接受一个参数size
,您可以根据需要调整星星的大小。
使用其他库来绘制五角星的方式有哪些?
除了turtle
库外,您还可以使用matplotlib
库来绘制五角星。使用matplotlib
,您可以更方便地调整图形的外观和样式。以下是一个简单的示例:
import matplotlib.pyplot as plt
import numpy as np
def draw_star():
angles = np.linspace(0, 2 * np.pi, 6)
x = np.sin(angles)
y = np.cos(angles)
plt.fill(x, y, color='yellow')
plt.axis('equal')
plt.show()
draw_star()
这种方法提供了更强大的绘图功能,适合制作复杂的图形。
如何自定义五角星的颜色和大小?
在使用turtle
库时,您可以通过turtle.color()
方法设置五角星的颜色,并通过传递不同的参数给draw_star
函数来改变大小。例如:
import turtle
def draw_star(size, color):
turtle.color(color)
for i in range(5):
turtle.forward(size)
turtle.right(144)
turtle.speed(1)
draw_star(100, 'blue')
turtle.done()
使用matplotlib
时,您可以在plt.fill()
函数中更改color
参数来改变颜色,同时可以调整size
参数以改变五角星的大小。
在绘制五角星时,可以添加哪些效果来增强视觉效果?
您可以通过添加背景、改变线条粗细、或使用渐变色来增强五角星的视觉效果。在turtle
库中,可以使用turtle.pensize()
来调整线条的粗细。在matplotlib
中,您可以使用渐变填充效果来增加视觉吸引力。以下是一个简单的调整示例:
import matplotlib.pyplot as plt
import numpy as np
def draw_star_with_effects():
angles = np.linspace(0, 2 * np.pi, 6)
x = np.sin(angles)
y = np.cos(angles)
plt.fill(x, y, color='orange', alpha=0.5) # 使用透明度增强效果
plt.axis('equal')
plt.grid(True) # 添加网格
plt.show()
draw_star_with_effects()
通过这些方法,您可以让五角星的绘制变得更加生动。
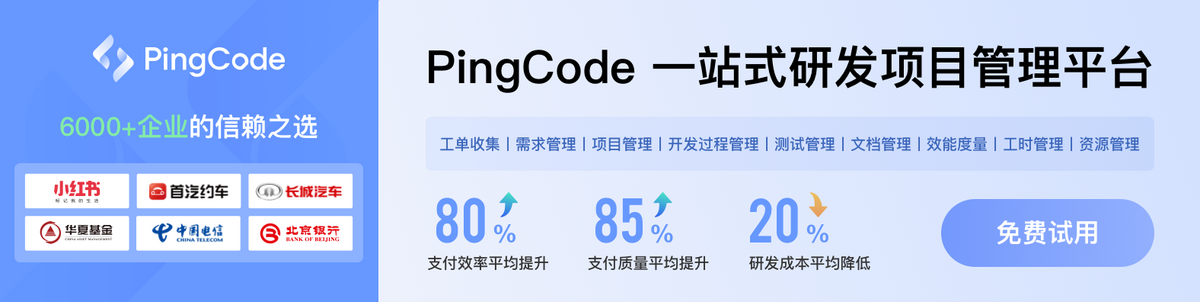