Python 使用三角函数的方法有:使用math模块、numpy模块、scipy模块。其中最常用的是math模块。以下是详细描述:
在Python中,三角函数的实现主要依赖于math模块。math模块提供了丰富的数学函数,包括正弦函数(sin)、余弦函数(cos)、正切函数(tan)等。math模块是Python的标准库,不需要额外安装,只需在代码中导入即可。举例来说,可以通过调用math.sin(x)来计算x的正弦值,其中x是以弧度为单位的角度值。
一、MATH模块的使用
math模块是Python中最常用的数学模块之一,它提供了一系列的三角函数,包括sin、cos、tan、asin、acos、atan等。以下是具体的使用方法:
1、导入math模块
在使用math模块中的三角函数之前,首先需要导入该模块:
import math
2、计算正弦值
使用math.sin()函数可以计算给定角度的正弦值。角度需要以弧度为单位:
# 计算45度的正弦值
angle_in_degrees = 45
angle_in_radians = math.radians(angle_in_degrees)
sine_value = math.sin(angle_in_radians)
print(f"The sine of {angle_in_degrees} degrees is {sine_value}")
3、计算余弦值
使用math.cos()函数可以计算给定角度的余弦值:
# 计算45度的余弦值
cosine_value = math.cos(angle_in_radians)
print(f"The cosine of {angle_in_degrees} degrees is {cosine_value}")
4、计算正切值
使用math.tan()函数可以计算给定角度的正切值:
# 计算45度的正切值
tangent_value = math.tan(angle_in_radians)
print(f"The tangent of {angle_in_degrees} degrees is {tangent_value}")
5、反三角函数
math模块还提供了反三角函数,用于计算弧度值。例如,math.asin()、math.acos()和math.atan()分别用于计算反正弦、反余弦和反正切值:
# 计算0.707的反正弦值
arc_sine_value = math.asin(0.707)
print(f"The arc sine of 0.707 is {math.degrees(arc_sine_value)} degrees")
二、NUMPY模块的使用
numpy模块是Python中用于科学计算的库,它也提供了丰富的三角函数。与math模块不同,numpy模块可以处理数组,因此在需要对大量数据进行三角运算时,numpy模块更加高效。
1、导入numpy模块
在使用numpy模块中的三角函数之前,首先需要导入该模块:
import numpy as np
2、计算数组的正弦值
使用numpy.sin()函数可以计算数组中每个元素的正弦值:
# 计算数组中每个元素的正弦值
angles_in_degrees = np.array([0, 30, 45, 60, 90])
angles_in_radians = np.radians(angles_in_degrees)
sine_values = np.sin(angles_in_radians)
print(f"Sine values: {sine_values}")
3、计算数组的余弦值
使用numpy.cos()函数可以计算数组中每个元素的余弦值:
# 计算数组中每个元素的余弦值
cosine_values = np.cos(angles_in_radians)
print(f"Cosine values: {cosine_values}")
4、计算数组的正切值
使用numpy.tan()函数可以计算数组中每个元素的正切值:
# 计算数组中每个元素的正切值
tangent_values = np.tan(angles_in_radians)
print(f"Tangent values: {tangent_values}")
三、SCIPY模块的使用
scipy模块是一个用于科学和技术计算的Python库,提供了比numpy更高级的函数。scipy.special模块中包含了许多特殊函数,包括三角函数。
1、导入scipy模块
在使用scipy模块中的三角函数之前,首先需要导入该模块:
import scipy.special as sp
2、计算特殊三角函数
scipy.special模块提供了一些特殊的三角函数,例如scipy.special.sindg()和scipy.special.cosdg()分别用于计算角度为度的正弦和余弦值:
# 计算45度的正弦值和余弦值
sine_value_deg = sp.sindg(45)
cosine_value_deg = sp.cosdg(45)
print(f"The sine of 45 degrees is {sine_value_deg}")
print(f"The cosine of 45 degrees is {cosine_value_deg}")
四、三角函数的实际应用
三角函数在工程、物理、计算机图形学等领域有着广泛的应用。以下是一些具体的应用实例:
1、计算两点之间的距离
在二维平面上,可以使用三角函数计算两点之间的距离:
import math
def calculate_distance(x1, y1, x2, y2):
delta_x = x2 - x1
delta_y = y2 - y1
distance = math.sqrt(delta_x<strong>2 + delta_y</strong>2)
return distance
x1, y1 = 0, 0
x2, y2 = 3, 4
distance = calculate_distance(x1, y1, x2, y2)
print(f"The distance between the points is {distance}")
2、计算角度和方向
在机器人和无人驾驶领域,可以使用三角函数计算方向和角度:
import math
def calculate_angle(x1, y1, x2, y2):
delta_x = x2 - x1
delta_y = y2 - y1
angle = math.atan2(delta_y, delta_x)
return math.degrees(angle)
x1, y1 = 0, 0
x2, y2 = 3, 4
angle = calculate_angle(x1, y1, x2, y2)
print(f"The angle between the points is {angle} degrees")
3、模拟波形信号
在信号处理和通信领域,可以使用三角函数生成和模拟波形信号:
import numpy as np
import matplotlib.pyplot as plt
生成正弦波信号
t = np.linspace(0, 1, 500)
frequency = 5 # 频率为5Hz
amplitude = 1 # 振幅为1
sine_wave = amplitude * np.sin(2 * np.pi * frequency * t)
绘制正弦波信号
plt.plot(t, sine_wave)
plt.title("Sine Wave")
plt.xlabel("Time (s)")
plt.ylabel("Amplitude")
plt.grid(True)
plt.show()
通过以上的描述,我们可以了解到Python中使用三角函数的方法,包括使用math模块、numpy模块和scipy模块。每种方法都有其独特的优势,选择适合自己的方法可以提高编程效率和代码的可读性。三角函数在实际应用中有着广泛的用途,例如计算两点之间的距离、计算角度和方向以及模拟波形信号等。在编写代码时,合理利用这些三角函数可以解决许多实际问题。
相关问答FAQs:
如何在Python中导入三角函数库?
在Python中,可以使用内置的math
库来访问三角函数。导入库时,只需使用import math
。这样,你就可以使用如math.sin()
、math.cos()
和math.tan()
等函数来计算三角函数值。
Python中的三角函数的输入单位是什么?
Python的math
库中的三角函数接受的输入单位为弧度。如果你需要使用角度进行计算,可以使用math.radians()
函数将角度转换为弧度。例如,math.sin(math.radians(30))
将计算30度的正弦值。
如何在Python中处理三角函数的反函数?
Python的math
库同样提供了三角函数的反函数,如math.asin()
、math.acos()
和math.atan()
。这些函数的返回值也是弧度,如果需要角度,可以使用math.degrees()
函数进行转换。例如,math.degrees(math.asin(0.5))
将返回30度。
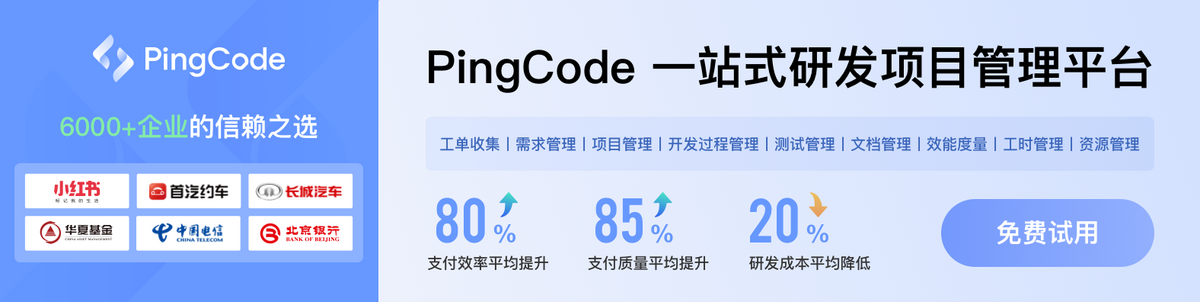