在Python中,可以通过多种方法去掉字符串后的换行符,例如使用strip()
、rstrip()
、replace()
等方法。下面将详细描述其中一种方法,并提供其他方法的简要说明。
一、使用strip()
方法去掉换行符
strip()
方法用于移除字符串开头和结尾的指定字符(默认为空格)。若要移除换行符,只需调用strip()
方法即可。
# 示例代码
text_with_newline = "Hello, World!\n"
cleaned_text = text_with_newline.strip()
print(cleaned_text) # 输出: Hello, World!
strip()
方法的优势在于它不仅能去掉字符串结尾的换行符,还能去掉字符串开头的换行符以及其他空白字符(如空格、制表符等)。这使得它非常适合处理需要清理两端空白字符的字符串。
二、使用rstrip()
方法去掉换行符
rstrip()
方法专门用于移除字符串末尾的指定字符。若要移除换行符,可以在调用rstrip()
方法时传入\n
。
# 示例代码
text_with_newline = "Hello, World!\n"
cleaned_text = text_with_newline.rstrip('\n')
print(cleaned_text) # 输出: Hello, World!
rstrip()
方法的优势在于它仅移除字符串末尾的指定字符,而不会影响字符串开头的字符。
三、使用replace()
方法去掉换行符
replace()
方法用于将字符串中的子字符串替换为另一个子字符串。可以使用它将换行符替换为空字符串,从而去掉换行符。
# 示例代码
text_with_newline = "Hello, World!\n"
cleaned_text = text_with_newline.replace('\n', '')
print(cleaned_text) # 输出: Hello, World!
replace()
方法的优势在于它可以处理字符串中的所有换行符(不仅限于末尾),并且可以指定替换的范围。
四、使用字符串切片去掉换行符
字符串切片是一种直接操作字符串的方法,可以通过切片操作去掉字符串末尾的换行符。
# 示例代码
text_with_newline = "Hello, World!\n"
cleaned_text = text_with_newline[:-1] if text_with_newline.endswith('\n') else text_with_newline
print(cleaned_text) # 输出: Hello, World!
这种方法的优势在于它不依赖于任何内置方法,直接通过索引操作字符串。
五、使用正则表达式去掉换行符
正则表达式是一种强大的字符串处理工具,可以用于查找和替换字符串中的特定模式。re
模块提供了正则表达式支持。
import re
示例代码
text_with_newline = "Hello, World!\n"
cleaned_text = re.sub(r'\n$', '', text_with_newline)
print(cleaned_text) # 输出: Hello, World!
正则表达式的优势在于它可以处理更复杂的模式匹配和替换任务,适用于需要进行复杂文本处理的场景。
六、综合比较与应用场景
不同方法各有优劣,选择合适的方法应根据具体应用场景进行。
strip()
方法:适用于需要移除两端空白字符的场景。rstrip()
方法:适用于仅需移除末尾特定字符的场景。replace()
方法:适用于需要替换字符串中所有特定字符的场景。- 字符串切片:适用于简单直接的字符串末尾字符移除。
- 正则表达式:适用于复杂模式匹配和替换任务。
七、扩展应用与示例
为了更好地理解这些方法,以下是一些扩展应用与示例。
1. 批量处理文件行
在处理文件时,通常需要去掉每行结尾的换行符。以下示例展示了如何使用strip()
方法批量处理文件行。
# 示例代码
with open('example.txt', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
print(cleaned_lines)
2. 处理多种换行符
在跨平台开发中,可能需要处理多种换行符(如\n
、\r\n
)。可以使用replace()
方法处理。
# 示例代码
text_with_newline = "Hello, World!\r\n"
cleaned_text = text_with_newline.replace('\r\n', '').replace('\n', '')
print(cleaned_text) # 输出: Hello, World!
3. 去掉多余空白字符
有时需要去掉字符串中的多余空白字符,包括换行符、空格、制表符等。可以结合strip()
或正则表达式处理。
import re
示例代码
text_with_whitespace = " Hello, \t World! \n"
cleaned_text = re.sub(r'\s+', ' ', text_with_whitespace).strip()
print(cleaned_text) # 输出: Hello, World!
八、总结
在Python中,可以通过多种方法去掉字符串后的换行符,包括strip()
、rstrip()
、replace()
、字符串切片、正则表达式等。选择合适的方法应根据具体应用场景进行。strip()
方法适用于移除两端空白字符,rstrip()
方法适用于移除末尾特定字符,replace()
方法适用于替换字符串中所有特定字符,字符串切片适用于简单直接的字符串末尾字符移除,正则表达式适用于复杂模式匹配和替换任务。通过综合比较与应用场景,可以更好地理解和应用这些方法。
相关问答FAQs:
如何在Python中去掉字符串末尾的特定字符?
在Python中,可以使用str.rstrip()
方法来去掉字符串末尾的特定字符。这个方法接受一个参数,表示要去除的字符集合。例如,如果想要去掉字符串末尾的字符'n',可以这样做:
my_string = "example stringnnn"
cleaned_string = my_string.rstrip('n')
print(cleaned_string) # 输出: example string
这个方法非常灵活,可以去掉多个不同的字符,只需将它们一起传入即可。
可以用什么方法去掉字符串中的所有特定字符?
如果需要从字符串中去掉所有的特定字符,而不仅仅是末尾的,可以使用str.replace()
方法。该方法可以用来替换字符串中的某个字符或子字符串。例如,去掉所有的'n'字符:
my_string = "example stringnnn"
cleaned_string = my_string.replace('n', '')
print(cleaned_string) # 输出: example strig
使用replace()
可以很方便地处理字符串中的字符。
在处理字符串时,如何处理多个字符的删除?
针对多个字符的删除,可以结合使用str.translate()
和str.maketrans()
方法。这样可以高效地删除多个不同的字符。例如,删除字符'n'和'e':
my_string = "example stringnnn"
translation_table = str.maketrans('', '', 'ne')
cleaned_string = my_string.translate(translation_table)
print(cleaned_string) # 输出: xampl strig
这种方法适合需要进行复杂字符删除的场景,提供了更大的灵活性。
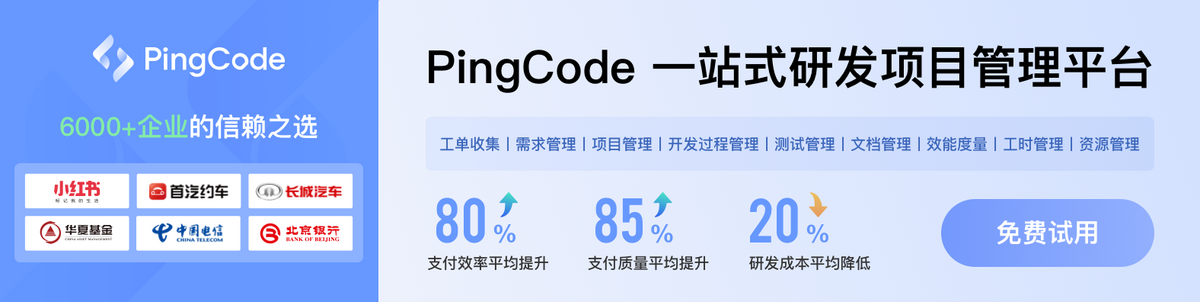