Python从数据库获取密码的方法有:使用数据库驱动连接数据库、执行SQL查询语句、通过ORM框架(如SQLAlchemy)操作数据库、使用安全库进行加密和解密。在这其中,使用数据库驱动连接数据库和执行SQL查询语句是最常见和直接的方法。接下来,我将详细描述如何通过这些方法从数据库中获取密码,并提供一些代码示例和安全建议。
一、使用数据库驱动连接数据库
Python有多种数据库驱动可以连接到不同类型的数据库,如MySQL、PostgreSQL、SQLite等。通过这些驱动,可以轻松地连接数据库并执行SQL查询语句来获取密码。以下是使用MySQL数据库驱动(mysql-connector-python)获取密码的示例代码:
import mysql.connector
def get_password(username):
# 连接到数据库
connection = mysql.connector.connect(
host="your_host",
user="your_username",
password="your_password",
database="your_database"
)
cursor = connection.cursor()
# 执行SQL查询语句
query = "SELECT password FROM users WHERE username = %s"
cursor.execute(query, (username,))
# 获取查询结果
result = cursor.fetchone()
# 关闭数据库连接
cursor.close()
connection.close()
if result:
return result[0]
else:
return None
示例用法
username = "example_user"
password = get_password(username)
if password:
print(f"Password for {username}: {password}")
else:
print(f"Password for {username} not found.")
二、使用ORM框架(如SQLAlchemy)操作数据库
ORM(Object-Relational Mapping)框架可以将数据库中的表映射为Python对象,简化数据库操作。SQLAlchemy是Python中最流行的ORM框架之一。以下是使用SQLAlchemy获取密码的示例代码:
from sqlalchemy import create_engine, Column, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
定义数据库模型
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
username = Column(String, primary_key=True)
password = Column(String)
创建数据库引擎
engine = create_engine('mysql+mysqlconnector://your_username:your_password@your_host/your_database')
创建会话
Session = sessionmaker(bind=engine)
session = Session()
def get_password(username):
# 查询数据库
user = session.query(User).filter_by(username=username).first()
if user:
return user.password
else:
return None
示例用法
username = "example_user"
password = get_password(username)
if password:
print(f"Password for {username}: {password}")
else:
print(f"Password for {username} not found.")
三、通过执行SQL查询语句获取密码
直接执行SQL查询语句是获取数据库中密码的最直接方法。这种方法适用于大多数数据库驱动,包括MySQL、PostgreSQL、SQLite等。以下是使用SQLite数据库驱动(sqlite3)获取密码的示例代码:
import sqlite3
def get_password(username):
# 连接到数据库
connection = sqlite3.connect('your_database.db')
cursor = connection.cursor()
# 执行SQL查询语句
query = "SELECT password FROM users WHERE username = ?"
cursor.execute(query, (username,))
# 获取查询结果
result = cursor.fetchone()
# 关闭数据库连接
cursor.close()
connection.close()
if result:
return result[0]
else:
return None
示例用法
username = "example_user"
password = get_password(username)
if password:
print(f"Password for {username}: {password}")
else:
print(f"Password for {username} not found.")
四、使用安全库进行加密和解密
在获取密码时,安全性是非常重要的。建议在存储密码时使用加密技术,并在获取密码时进行解密。以下是使用Python的cryptography库进行加密和解密的示例代码:
from cryptography.fernet import Fernet
import sqlite3
生成密钥并保存到文件
def generate_key():
key = Fernet.generate_key()
with open('secret.key', 'wb') as key_file:
key_file.write(key)
加载密钥
def load_key():
return open('secret.key', 'rb').read()
加密密码
def encrypt_password(password, key):
f = Fernet(key)
encrypted_password = f.encrypt(password.encode())
return encrypted_password
解密密码
def decrypt_password(encrypted_password, key):
f = Fernet(key)
decrypted_password = f.decrypt(encrypted_password).decode()
return decrypted_password
示例:加密密码并存储到数据库
def store_encrypted_password(username, password):
key = load_key()
encrypted_password = encrypt_password(password, key)
connection = sqlite3.connect('your_database.db')
cursor = connection.cursor()
cursor.execute("INSERT INTO users (username, password) VALUES (?, ?)", (username, encrypted_password))
connection.commit()
cursor.close()
connection.close()
示例:从数据库中获取加密的密码并解密
def get_decrypted_password(username):
key = load_key()
connection = sqlite3.connect('your_database.db')
cursor = connection.cursor()
cursor.execute("SELECT password FROM users WHERE username = ?", (username,))
result = cursor.fetchone()
cursor.close()
connection.close()
if result:
encrypted_password = result[0]
return decrypt_password(encrypted_password, key)
else:
return None
生成密钥并保存到文件(只需运行一次)
generate_key()
示例用法
username = "example_user"
password = "example_password"
加密并存储密码
store_encrypted_password(username, password)
获取并解密密码
decrypted_password = get_decrypted_password(username)
if decrypted_password:
print(f"Decrypted password for {username}: {decrypted_password}")
else:
print(f"Password for {username} not found.")
五、总结
从数据库获取密码是一项常见的任务,可以使用多种方法完成,包括数据库驱动连接数据库、执行SQL查询语句、使用ORM框架和安全库进行加密和解密。在实际应用中,建议结合使用这些方法,以确保密码的安全性。例如,可以使用ORM框架简化数据库操作,使用安全库加密和解密密码,确保密码在存储和传输过程中的安全性。无论采用哪种方法,都应遵循最佳实践,确保数据库连接和密码处理的安全性。
相关问答FAQs:
如何在Python中安全地从数据库中获取密码?
在Python中获取密码时,确保安全是至关重要的。您可以使用库如sqlite3
或SQLAlchemy
连接到数据库。使用参数化查询可以防止SQL注入,确保只取出必要的字段。请注意,不应直接存储明文密码,而应存储其哈希值。获取密码时,您应该先获取哈希值,并使用安全的哈希算法(如bcrypt)进行比对。
如何有效管理数据库中的用户密码?
有效管理数据库中的用户密码包括使用强密码策略和适当的哈希算法。密码应至少包含字母、数字及特殊字符。采用bcrypt
或argon2
等现代哈希算法可以增加密码的安全性。此外,定期更新密码和实施账户锁定策略也是重要的管理措施。
在Python中如何处理数据库连接和密码获取的异常?
处理数据库连接和密码获取的异常至关重要。使用try-except
块可以捕获连接错误和查询错误。在出现异常时,可以记录错误信息并采取适当的措施,如重试连接或通知用户。此外,确保在发生异常时及时关闭数据库连接,以防资源泄漏。
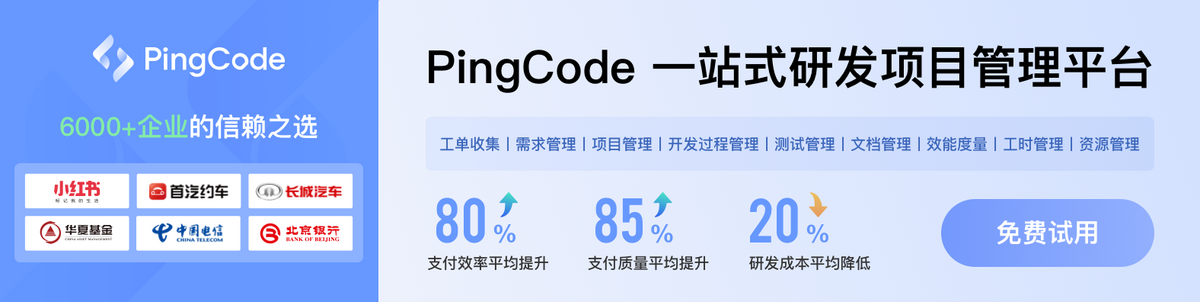