如何使用Python自动化运维
使用Python进行自动化运维的关键在于利用其强大的库和模块来简化和自动化日常任务、提高效率和减少人为错误。Python的简洁性、丰富的库、跨平台支持使其成为自动化运维的理想选择。本文将详细探讨如何通过Python实现自动化运维,包括脚本编写、常用库介绍、实战案例以及最佳实践。
一、Python自动化运维的优势
Python在自动化运维中的优势主要体现在以下几个方面:
- 简洁易学:Python的语法简单,易于学习和使用,特别适合运维人员快速上手。
- 丰富的库支持:Python拥有丰富的第三方库,如Paramiko、Fabric、Ansible等,可以帮助快速实现自动化任务。
- 跨平台支持:Python可以在Windows、Linux、macOS等多个平台上运行,具有很强的跨平台能力。
- 社区和文档:Python拥有庞大的社区和丰富的文档资源,可以方便地找到解决问题的方法和最佳实践。
二、常用Python库介绍
在自动化运维中,以下几个Python库尤为常用:
1、Paramiko
Paramiko是一个用于处理SSH连接的模块,适用于远程服务器管理。它可以用于执行远程命令、文件传输等操作。
import paramiko
def ssh_connect(hostname, port, username, password):
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, port, username, password)
return client
def execute_command(client, command):
stdin, stdout, stderr = client.exec_command(command)
return stdout.read().decode()
client = ssh_connect('your_server_ip', 22, 'your_username', 'your_password')
output = execute_command(client, 'ls -la')
print(output)
client.close()
2、Fabric
Fabric是一个高级的Python库,基于Paramiko构建,旨在简化SSH连接和命令执行。它可以方便地定义和执行部署任务。
from fabric import Connection
def deploy():
conn = Connection(host='your_server_ip', user='your_username', connect_kwargs={'password': 'your_password'})
conn.run('ls -la')
conn.put('local_file_path', 'remote_file_path')
deploy()
3、Ansible
Ansible是一个开源的自动化工具,使用YAML语言编写剧本(Playbook),适用于配置管理、应用部署、任务执行等。
- name: Install and start Apache
hosts: webservers
become: yes
tasks:
- name: Install Apache
apt: name=apache2 update_cache=yes state=latest
- name: Start Apache
service: name=apache2 state=started
三、Python自动化运维实战案例
1、自动备份数据库
自动备份是运维的重要任务之一。下面是一个使用Python实现MySQL数据库自动备份的示例:
import os
import time
def backup_mysql(user, password, db_name, backup_dir):
timestamp = time.strftime('%Y%m%d%H%M%S')
backup_file = os.path.join(backup_dir, f'{db_name}_{timestamp}.sql')
dump_cmd = f'mysqldump -u {user} -p{password} {db_name} > {backup_file}'
os.system(dump_cmd)
print(f'Backup completed: {backup_file}')
backup_mysql('your_db_user', 'your_db_password', 'your_db_name', '/path/to/backup/dir')
2、监控服务器状态
通过Python脚本可以定期监控服务器的状态,并将结果发送到指定的邮箱。
import smtplib
from email.mime.text import MIMEText
import psutil
def send_email(subject, message, from_addr, to_addr, smtp_server, smtp_port, smtp_user, smtp_password):
msg = MIMEText(message)
msg['Subject'] = subject
msg['From'] = from_addr
msg['To'] = to_addr
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(smtp_user, smtp_password)
server.sendmail(from_addr, to_addr, msg.as_string())
def monitor_server():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
disk_info = psutil.disk_usage('/')
message = f"CPU Usage: {cpu_usage}%\n"
message += f"Memory Usage: {memory_info.percent}%\n"
message += f"Disk Usage: {disk_info.percent}%\n"
send_email(
'Server Status Report', message,
'your_email@example.com', 'recipient@example.com',
'smtp.example.com', 587, 'your_email@example.com', 'your_email_password'
)
monitor_server()
四、最佳实践
在实际应用中,遵循一些最佳实践可以提高自动化运维的质量和效率:
1、模块化和可复用性
将代码模块化,使其具备良好的可复用性。避免重复代码,提高维护性和扩展性。
def connect_to_server(host, user, password):
# Connection code here
pass
def execute_task():
# Task execution code here
pass
def main():
connect_to_server('host', 'user', 'password')
execute_task()
if __name__ == '__main__':
main()
2、错误处理和日志记录
在脚本中添加错误处理和日志记录,以便在出错时能够快速定位问题。
import logging
logging.basicConfig(filename='automation.log', level=logging.INFO)
def perform_task():
try:
# Task code here
logging.info('Task completed successfully.')
except Exception as e:
logging.error(f'Error occurred: {e}')
perform_task()
3、安全性
在涉及敏感信息(如密码)的场景中,避免在代码中明文存储这些信息。可以使用环境变量或加密的方式存储和读取这些信息。
import os
def get_db_password():
return os.getenv('DB_PASSWORD')
db_password = get_db_password()
五、案例分析
1、自动化部署Web应用
使用Python脚本自动化部署一个Django Web应用,包括从代码库拉取代码、安装依赖、迁移数据库以及重启服务。
import os
from fabric import Connection
def deploy():
conn = Connection(host='your_server_ip', user='your_username', connect_kwargs={'password': 'your_password'})
with conn.cd('/path/to/your/app'):
conn.run('git pull origin main')
conn.run('pip install -r requirements.txt')
conn.run('python manage.py migrate')
conn.run('systemctl restart your_app_service')
deploy()
2、自动化日志分析
使用Python定期分析服务器日志,提取关键数据,并生成报告。
import re
import os
def analyze_logs(log_file):
error_pattern = re.compile(r'ERROR')
error_count = 0
with open(log_file, 'r') as file:
for line in file:
if error_pattern.search(line):
error_count += 1
return error_count
def generate_report(error_count, report_file):
with open(report_file, 'w') as file:
file.write(f'Total Errors: {error_count}\n')
log_file = '/path/to/log/file.log'
report_file = '/path/to/report/file.txt'
error_count = analyze_logs(log_file)
generate_report(error_count, report_file)
六、总结
通过本文的介绍,我们了解了如何使用Python进行自动化运维,包括常用的Python库、实战案例以及最佳实践。Python的简洁性、丰富的库支持、跨平台能力使其成为自动化运维的理想工具。在实际应用中,遵循模块化、错误处理和安全性的最佳实践,可以提高自动化运维的质量和效率。希望本文能够对您在实际工作中使用Python进行自动化运维提供帮助。
Python自动化运维不仅仅是编写脚本,更是解决实际问题的一种思维方式。通过不断学习和实践,您可以更好地掌握Python自动化运维的技能,为日常工作带来更多便利和效率。
相关问答FAQs:
如何使用Python进行自动化运维的入门步骤是什么?
要开始使用Python进行自动化运维,首先需要安装Python环境及相应的库,例如paramiko
(用于SSH连接)和requests
(用于API调用)。了解基本的Python语法和数据结构是非常重要的。接下来,可以尝试编写一些简单的脚本,例如自动化服务器的监控和日志收集。逐步增加复杂性,探索更多的库和工具,例如Ansible等,以提升自动化的效率。
Python自动化运维能解决哪些具体问题?
Python可以帮助解决许多运维中的常见问题,比如自动化部署、配置管理、监控、日志分析等。通过编写脚本,运维人员能够快速完成重复性任务,减少人为错误,并提高工作效率。此外,利用Python,可以集成各种API,实时获取系统状态或进行故障排除,极大地提升了运维的灵活性和响应速度。
是否有推荐的Python库或工具来帮助进行运维自动化?
有许多优秀的Python库和工具可以帮助进行运维自动化。比如,Fabric
可以用于简化SSH操作,SaltStack
和Ansible
都是流行的配置管理工具。此外,Pandas
和NumPy
可以帮助处理和分析日志数据,Flask
可以用于构建简单的API接口。这些工具的结合使用,可以大大提升运维的自动化水平和效率。
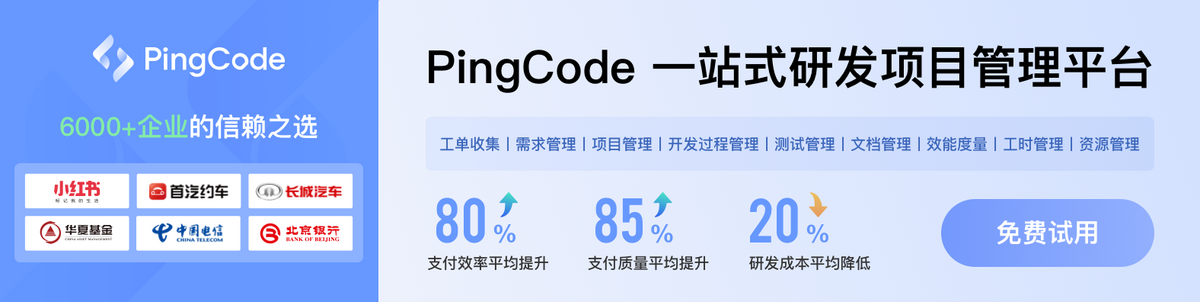