使用Python读取文件中多个图片数量的方法有多种,例如使用os
模块、glob
模块或第三方库如Pillow
或OpenCV
。最常用的方法包括使用os模块列出目录内容、使用glob模块进行模式匹配、使用Pillow库读取图像文件、使用OpenCV库读取图像文件。下面将详细介绍这些方法中的一种。
使用os模块列出目录内容。os模块是Python标准库的一部分,提供了与操作系统进行交互的功能。在读取文件夹中的图片数量时,os模块可以用于列出文件夹中的所有文件,并通过文件扩展名来筛选出图像文件。
一、os模块读取图片数量
os模块提供了一系列函数来与操作系统进行交互。我们可以使用os.listdir()
函数列出指定目录中的所有文件和目录,然后通过文件扩展名来筛选出图片文件。
示例代码:
import os
def count_images_in_directory(directory):
# 定义图片扩展名
image_extensions = ('.jpg', '.jpeg', '.png', '.gif', '.bmp', '.tiff')
# 获取目录中的所有文件
files = os.listdir(directory)
# 筛选出图片文件
image_files = [file for file in files if file.lower().endswith(image_extensions)]
# 返回图片数量
return len(image_files)
示例目录
directory_path = '/path/to/your/image/directory'
image_count = count_images_in_directory(directory_path)
print(f'There are {image_count} images in the directory.')
在上面的代码中,我们首先定义了常见的图片文件扩展名,然后使用os.listdir()
函数列出指定目录中的所有文件,通过列表推导式筛选出图片文件,最后返回图片数量。
二、glob模块读取图片数量
glob模块提供了一个函数来根据模式匹配找到所有路径名。它可以用于查找符合特定模式的文件。
示例代码:
import glob
def count_images_in_directory(directory):
# 定义图片扩展名模式
image_patterns = ['*.jpg', '*.jpeg', '*.png', '*.gif', '*.bmp', '*.tiff']
# 初始化图片数量
image_count = 0
# 遍历每种图片模式
for pattern in image_patterns:
# 使用glob.glob()匹配模式
image_files = glob.glob(os.path.join(directory, pattern))
# 累加图片数量
image_count += len(image_files)
return image_count
示例目录
directory_path = '/path/to/your/image/directory'
image_count = count_images_in_directory(directory_path)
print(f'There are {image_count} images in the directory.')
在上面的代码中,我们定义了常见的图片文件扩展名模式,然后使用glob.glob()
函数匹配目录中的文件,通过累加匹配到的文件数量来计算图片数量。
三、Pillow库读取图片数量
Pillow(PIL)库是Python中用于图像处理的第三方库。虽然Pillow库主要用于图像处理,但也可以用于读取目录中的图片文件。
示例代码:
from PIL import Image
import os
def is_image(file_path):
try:
# 尝试打开文件
with Image.open(file_path) as img:
return True
except IOError:
return False
def count_images_in_directory(directory):
# 获取目录中的所有文件
files = os.listdir(directory)
# 初始化图片数量
image_count = 0
# 遍历文件
for file in files:
file_path = os.path.join(directory, file)
# 判断文件是否为图片
if is_image(file_path):
image_count += 1
return image_count
示例目录
directory_path = '/path/to/your/image/directory'
image_count = count_images_in_directory(directory_path)
print(f'There are {image_count} images in the directory.')
在上面的代码中,我们定义了一个is_image()
函数来判断文件是否为图片,然后通过遍历目录中的所有文件来计算图片数量。
四、OpenCV库读取图片数量
OpenCV是一个强大的计算机视觉库,提供了丰富的图像处理功能。我们可以使用OpenCV库来读取目录中的图片文件。
示例代码:
import cv2
import os
def is_image(file_path):
try:
# 尝试读取图像文件
img = cv2.imread(file_path)
return img is not None
except Exception:
return False
def count_images_in_directory(directory):
# 获取目录中的所有文件
files = os.listdir(directory)
# 初始化图片数量
image_count = 0
# 遍历文件
for file in files:
file_path = os.path.join(directory, file)
# 判断文件是否为图片
if is_image(file_path):
image_count += 1
return image_count
示例目录
directory_path = '/path/to/your/image/directory'
image_count = count_images_in_directory(directory_path)
print(f'There are {image_count} images in the directory.')
在上面的代码中,我们定义了一个is_image()
函数来判断文件是否为图片,然后通过遍历目录中的所有文件来计算图片数量。
总结
通过上述方法,我们可以方便地使用Python读取文件夹中的图片数量。每种方法都有其独特的优势,具体选择哪种方法可以根据实际需求和个人偏好来决定。希望本文对你在Python中处理图片文件有所帮助。
相关问答FAQs:
如何在Python中读取文件夹中的所有图片?
要读取文件夹中的所有图片,可以使用Python的os库来获取文件夹内的所有文件名,并结合PIL(Pillow)库来处理图像。您可以通过以下示例代码实现:
import os
from PIL import Image
folder_path = 'your_folder_path'
image_files = [f for f in os.listdir(folder_path) if f.endswith(('jpg', 'jpeg', 'png', 'gif'))]
for image_file in image_files:
img = Image.open(os.path.join(folder_path, image_file))
# 处理图像的代码
这样就可以轻松读取指定文件夹中的所有图片。
如何判断文件是否为图片格式?
在读取文件时,确保文件是图片格式非常重要。可以通过检查文件扩展名来判断。常见的图片格式包括jpg、jpeg、png、gif等。使用os库的endswith
方法,可以有效地过滤出图片文件。例如:
if file.endswith(('jpg', 'jpeg', 'png', 'gif')):
# 处理图片
这样可以确保只处理有效的图像文件,避免出现错误。
读取图片数量时有哪些常用的方法?
在Python中,可以使用os库来获取文件夹内的文件数量。结合上面的代码示例,可以通过len()
函数来计算图片数量:
image_count = len(image_files)
print(f'图片数量: {image_count}')
这种方法简单有效,适用于大多数场景。如果需要更复杂的处理,可以考虑使用glob库,它支持更灵活的文件匹配模式。
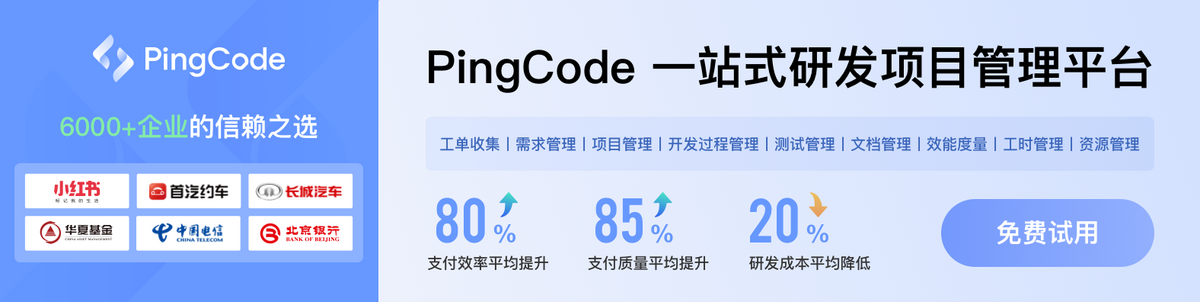