使用Python去掉文本中的标点符号可以通过多种方法来实现,常用的方法有正则表达式、字符串的translate方法、以及逐字符遍历。其中,使用正则表达式是最为灵活和高效的方法之一。下面将详细介绍这些方法,并给出具体的代码示例。
一、正则表达式方法
正则表达式是一种强大的文本处理工具,能够方便地匹配和替换特定的字符或模式。Python的re
模块提供了对正则表达式的支持。
import re
def remove_punctuation(text):
# 使用正则表达式去除标点符号
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text)
在上述代码中,re.sub(r'[^\w\s]', '', text)
使用了一个正则表达式模式[^\w\s]
,其中^
表示取反,\w
匹配任何字母数字字符,\s
匹配任何空白字符。所以这个模式匹配的是所有非字母数字和非空白字符的标点符号,并将其替换为空字符串,从而实现去除标点符号的效果。
二、translate方法
Python的字符串方法translate
配合str.maketrans
也可以用于去除标点符号。str.maketrans
可以创建一个字符映射表,用于将特定字符替换或删除。
import string
def remove_punctuation(text):
# 创建一个映射表,将所有标点符号映射为空字符
translator = str.maketrans('', '', string.punctuation)
return text.translate(translator)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text)
在上述代码中,string.punctuation
包含了所有的标点符号,str.maketrans('', '', string.punctuation)
创建了一个将所有标点符号映射为空字符的字符映射表,并通过text.translate(translator)
将文本中的标点符号删除。
三、逐字符遍历
逐字符遍历的方法通过遍历文本中的每个字符,并检查该字符是否是标点符号。如果不是标点符号,则将其添加到结果字符串中。
import string
def remove_punctuation(text):
# 定义标点符号集合
punctuation_set = set(string.punctuation)
# 逐字符遍历,去除标点符号
return ''.join(char for char in text if char not in punctuation_set)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text)
在上述代码中,set(string.punctuation)
创建了一个包含所有标点符号的集合,通过逐字符遍历文本,并将非标点符号的字符添加到结果字符串中,从而实现去除标点符号的效果。
四、使用自定义函数
有时候我们可能需要自定义标点符号的定义和处理方式,例如只去除特定的标点符号或者保留某些特殊符号。可以通过编写自定义函数来实现。
def remove_custom_punctuation(text, punctuation):
# 创建一个映射表,将自定义标点符号映射为空字符
translator = str.maketrans('', '', punctuation)
return text.translate(translator)
text = "Hello, world! This is a test."
custom_punctuation = ",!."
clean_text = remove_custom_punctuation(text, custom_punctuation)
print(clean_text)
在上述代码中,remove_custom_punctuation
函数接受两个参数:text
是要处理的文本,punctuation
是要去除的自定义标点符号字符串。通过创建一个映射表并调用text.translate(translator)
实现自定义标点符号的去除。
五、结合多种方法
在实际应用中,可以结合多种方法来实现更加复杂和灵活的标点符号去除。例如,可以先使用正则表达式去除大部分标点符号,然后使用自定义函数去除剩余的特殊符号。
import re
def remove_punctuation(text, custom_punctuation=''):
# 使用正则表达式去除大部分标点符号
text = re.sub(r'[^\w\s]', '', text)
# 使用自定义函数去除剩余的特殊符号
translator = str.maketrans('', '', custom_punctuation)
return text.translate(translator)
text = "Hello, world! This is a test."
custom_punctuation = "!"
clean_text = remove_punctuation(text, custom_punctuation)
print(clean_text)
在上述代码中,remove_punctuation
函数先使用正则表达式去除大部分标点符号,然后再使用自定义函数去除剩余的特殊符号。这样可以实现更加灵活和全面的标点符号去除效果。
六、应用场景和注意事项
去除文本中的标点符号在很多应用场景中非常有用,例如:
- 文本预处理:在自然语言处理和文本分析中,去除标点符号是常见的预处理步骤之一,可以帮助简化文本和提高处理效率。
- 数据清洗:在数据清洗过程中,去除标点符号可以帮助去除噪音数据,使数据更加整洁和规范。
- 文本搜索和匹配:在进行文本搜索和匹配时,去除标点符号可以提高匹配的准确性和灵活性。
在使用上述方法时,需要注意以下几点:
- 字符集和编码:确保文本的字符集和编码正确,避免由于编码问题导致的处理错误。
- 标点符号的定义:根据具体需求确定标点符号的定义和范围,有时可能需要保留某些特殊符号。
- 性能和效率:对于大规模文本数据,选择高效的方法和算法,避免性能瓶颈。
总结
本文详细介绍了使用Python去掉文本中的标点符号的多种方法,包括正则表达式、translate方法、逐字符遍历、自定义函数以及结合多种方法。每种方法都有其优缺点和适用场景,读者可以根据具体需求选择合适的方法进行实现。希望本文能够帮助读者更好地理解和应用Python去除标点符号的技术。
相关问答FAQs:
如何在Python中去除特定类型的标点符号?
在Python中,可以使用字符串的replace()
方法或正则表达式库re
来去除特定类型的标点符号。例如,如果只想去掉句号和逗号,可以这样做:
text = "Hello, world. How are you?"
text = text.replace(",", "").replace(".", "")
print(text) # 输出: Hello world How are you?
使用正则表达式时,可以通过以下方式实现:
import re
text = "Hello, world. How are you?"
text = re.sub(r"[,.]", "", text)
print(text) # 输出: Hello world How are you?
使用Python去掉所有标点符号的最佳方法是什么?
如果需要去掉所有的标点符号,可以利用string
模块中的punctuation
属性,结合str.translate()
方法:
import string
text = "Hello, world! How's it going?"
translator = str.maketrans("", "", string.punctuation)
cleaned_text = text.translate(translator)
print(cleaned_text) # 输出: Hello world Hows it going
这种方法高效且简洁,适用于去除文本中的所有标点符号。
在去掉标点符号后,如何确保文本的完整性和可读性?
去掉标点符号可能会影响文本的可读性。为了保持文本的完整性,可以考虑在去掉标点的同时保留必要的空格,以确保单词之间的分隔。例如,可以在去掉标点后,使用str.split()
和str.join()
来重新构建字符串:
import string
text = "Hello, world! How's it going?"
translator = str.maketrans("", "", string.punctuation)
cleaned_text = text.translate(translator)
cleaned_text = ' '.join(cleaned_text.split())
print(cleaned_text) # 输出: Hello world Hows it going
这种方法不仅去掉了标点,也确保了单词之间的适当间隔,提升了文本的可读性。
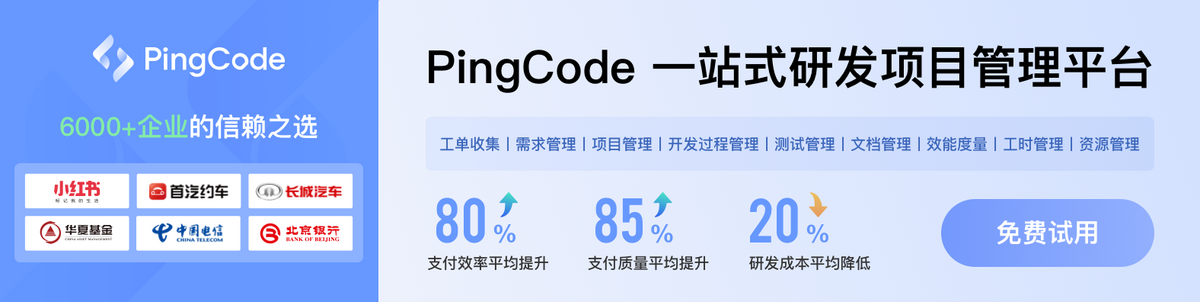