Python多线程间的通信可以通过队列、事件、锁、条件变量等方式来实现。其中,队列(Queue)是最常用的一种方法,因为它能提供线程安全的、先进先出的消息传递机制。下面将详细展开如何使用队列来实现多线程间的通信。
一、队列(Queue)
队列是Python标准库中的queue
模块提供的一个类,适用于多线程环境下的消息传递。它通过锁机制确保线程安全,可以避免多线程竞争条件。以下是如何使用队列进行多线程通信的详细说明:
1、基本使用
首先,你需要导入queue
模块并创建一个队列对象。你可以使用Queue
类来创建一个FIFO队列,使用LifoQueue
来创建一个LIFO队列,或者使用PriorityQueue
来创建一个优先级队列。
import queue
q = queue.Queue()
接下来,你可以使用put()
方法将消息放入队列,使用get()
方法从队列中取出消息。
# 向队列中放入消息
q.put('message')
从队列中取出消息
message = q.get()
2、生产者-消费者模型
队列在生产者-消费者模型中尤为有用。生产者线程负责生成数据并将其放入队列,而消费者线程从队列中取出数据进行处理。以下是一个简单的生产者-消费者模型示例:
import threading
import queue
import time
创建队列
q = queue.Queue()
def producer():
for i in range(5):
item = f'item {i}'
q.put(item)
print(f'Produced {item}')
time.sleep(1)
def consumer():
while True:
item = q.get()
if item is None:
break
print(f'Consumed {item}')
time.sleep(2)
创建并启动生产者线程
producer_thread = threading.Thread(target=producer)
producer_thread.start()
创建并启动消费者线程
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
等待生产者线程完成
producer_thread.join()
向队列发送终止信号
q.put(None)
等待消费者线程完成
consumer_thread.join()
在这个示例中,生产者线程生成5个项目并将其放入队列中,消费者线程从队列中取出项目进行处理。通过向队列发送一个None
作为终止信号,消费者线程可以知道何时终止。
二、事件(Event)
事件是一种简单的同步机制,可以用于线程间的信号传递。通过使用threading.Event
对象,一个线程可以等待另一个线程发出信号。以下是一个简单的示例:
import threading
import time
创建事件对象
event = threading.Event()
def worker():
print('Waiting for event to be set...')
event.wait()
print('Event received!')
创建并启动工作线程
worker_thread = threading.Thread(target=worker)
worker_thread.start()
模拟延迟
time.sleep(3)
设置事件
event.set()
等待工作线程完成
worker_thread.join()
在这个示例中,工作线程会等待事件被设置(即等待信号),主线程在3秒后设置事件,工作线程收到信号后继续执行。
三、锁(Lock)
锁是一种常见的同步机制,用于防止多个线程同时访问共享资源。通过使用threading.Lock
对象,你可以确保同一时间只有一个线程可以访问共享资源。以下是一个简单的示例:
import threading
创建锁对象
lock = threading.Lock()
shared_resource = 0
def worker():
global shared_resource
with lock:
# 访问共享资源
shared_resource += 1
print(f'Shared resource: {shared_resource}')
创建并启动多个工作线程
threads = []
for i in range(5):
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
在这个示例中,多个工作线程通过使用锁来确保同一时间只有一个线程可以访问和修改共享资源。
四、条件变量(Condition)
条件变量是一种更高级的同步机制,允许线程等待特定的条件被满足。通过使用threading.Condition
对象,你可以实现更复杂的线程间通信。以下是一个简单的示例:
import threading
import time
创建条件变量对象
condition = threading.Condition()
data_ready = False
def producer():
global data_ready
with condition:
print('Producing data...')
time.sleep(2)
data_ready = True
print('Data produced.')
condition.notify()
def consumer():
global data_ready
with condition:
while not data_ready:
print('Waiting for data...')
condition.wait()
print('Data received!')
创建并启动消费者线程
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
模拟延迟
time.sleep(1)
创建并启动生产者线程
producer_thread = threading.Thread(target=producer)
producer_thread.start()
等待所有线程完成
producer_thread.join()
consumer_thread.join()
在这个示例中,消费者线程会等待数据准备好,生产者线程在生产完数据后通知消费者线程。
五、信号量(Semaphore)
信号量是一种计数器,用于控制对共享资源的访问。通过使用threading.Semaphore
对象,你可以限制同时访问共享资源的线程数。以下是一个简单的示例:
import threading
import time
创建信号量对象,允许同时访问的线程数为2
semaphore = threading.Semaphore(2)
def worker(id):
with semaphore:
print(f'Thread {id} is working...')
time.sleep(2)
print(f'Thread {id} finished work.')
创建并启动多个工作线程
threads = []
for i in range(5):
thread = threading.Thread(target=worker, args=(i,))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
在这个示例中,信号量限制了同时访问共享资源的线程数为2,这意味着最多有两个线程可以同时执行工作。
六、总结
在多线程编程中,线程间的通信和同步是非常重要的。Python提供了多种机制来实现这些功能,包括队列、事件、锁、条件变量和信号量。每种机制都有其适用的场景和优势,选择合适的机制可以帮助你编写出高效且可靠的多线程程序。
队列适用于生产者-消费者模型,事件适用于简单的信号传递,锁适用于防止共享资源的竞争条件,条件变量适用于复杂的线程间通信,信号量适用于限制同时访问共享资源的线程数。了解并掌握这些机制,可以让你在多线程编程中游刃有余。
相关问答FAQs:
多线程通信的常用方法有哪些?
在Python中,多线程之间的通信可以通过多种方式实现。最常见的方法包括使用队列(Queue)、事件(Event)、条件(Condition)和信号量(Semaphore)。队列是线程安全的,适合用于在多个线程之间传递数据。事件和条件则适合用于线程之间的协调和状态同步,而信号量则用于控制对共享资源的访问。
如何使用队列实现线程之间的通信?
使用Python的queue
模块可以轻松实现线程间的通信。创建一个Queue
对象后,生产者线程可以将数据放入队列,而消费者线程可以从队列中取出数据。这样可以有效避免多线程环境下数据竞争的问题。示例代码如下:
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f'生产者生产: {i}')
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f'消费者消费: {item}')
q = queue.Queue()
t1 = threading.Thread(target=producer, args=(q,))
t2 = threading.Thread(target=consumer, args=(q,))
t1.start()
t2.start()
t1.join()
q.put(None) # 发送结束信号
t2.join()
在多线程环境中,如何处理数据共享问题?
在多线程环境中,数据共享可能导致竞争条件和不一致的问题。使用锁(Lock)是解决这一问题的有效方法。通过在访问共享资源时使用锁,可以确保同一时刻只有一个线程能够访问该资源,避免数据损坏。Python的threading
模块提供了简单的锁机制,可以轻松实现。
在多线程通信中,如何使用事件进行线程同步?
事件是一种用于线程间通信的同步原语。通过设置和清除事件,线程可以在特定条件下进行协调。例如,一个线程可以等待另一个线程完成某项任务后再继续执行。使用threading.Event
对象可以轻松实现这种功能,以下是一个示例:
import threading
import time
event = threading.Event()
def waiter():
print("等待事件...")
event.wait() # 等待事件被设置
print("事件被设置,继续执行。")
def setter():
time.sleep(2)
print("设置事件。")
event.set() # 设置事件
t1 = threading.Thread(target=waiter)
t2 = threading.Thread(target=setter)
t1.start()
t2.start()
t1.join()
t2.join()
通过以上示例,可以看到如何利用事件在多个线程间进行有效的通信与同步。
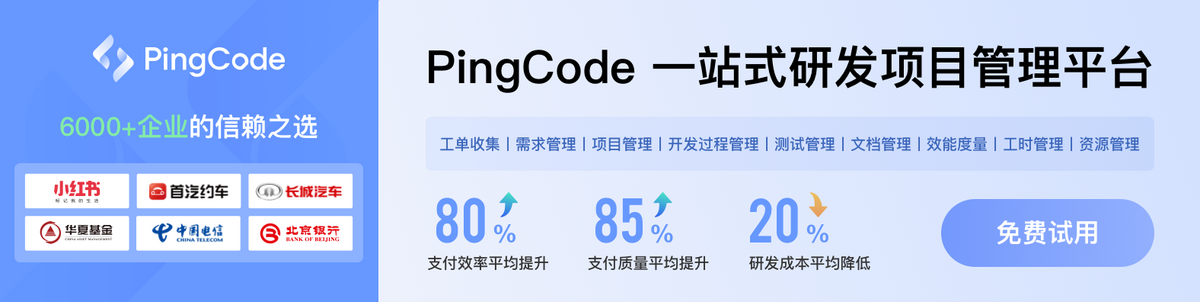