Python3 检测目前文件路径的方法有多种,常见的包括使用 os 模块、pathlib 模块、inspect 模块等。
其中,使用 os
模块是最常见且简单的方法。通过 os 模块中的 os.getcwd()
可以获取当前的工作目录,使用 os.path.abspath(__file__)
可以获取当前文件的绝对路径,使用 os.path.dirname(os.path.abspath(__file__))
可以获取当前文件所在的目录。以下是详细介绍及示例代码。
一、使用 os 模块检测文件路径
1. 获取当前工作目录
通过 os.getcwd()
获取当前脚本执行时的工作目录。
import os
current_working_directory = os.getcwd()
print("当前工作目录为:", current_working_directory)
os.getcwd()
返回当前的工作目录,这个目录是脚本运行时所在的目录。
2. 获取当前文件的绝对路径
通过 os.path.abspath(__file__)
获取当前文件的绝对路径。
import os
current_file_path = os.path.abspath(__file__)
print("当前文件的绝对路径为:", current_file_path)
os.path.abspath(__file__)
返回当前文件的绝对路径,这对于需要确定脚本文件本身的位置非常有用。
3. 获取当前文件的目录
通过 os.path.dirname(os.path.abspath(__file__))
获取当前文件所在的目录。
import os
current_file_directory = os.path.dirname(os.path.abspath(__file__))
print("当前文件所在的目录为:", current_file_directory)
os.path.dirname(os.path.abspath(__file__))
返回当前文件所在的目录路径,这在需要引用同一目录下的其他文件时非常有用。
二、使用 pathlib 模块检测文件路径
Python 3.4 引入了 pathlib
模块,使得路径操作更加面向对象且易于使用。
1. 获取当前工作目录
通过 Path.cwd()
获取当前脚本执行时的工作目录。
from pathlib import Path
current_working_directory = Path.cwd()
print("当前工作目录为:", current_working_directory)
Path.cwd()
返回当前的工作目录,效果与 os.getcwd()
类似。
2. 获取当前文件的绝对路径
通过 Path(__file__).resolve()
获取当前文件的绝对路径。
from pathlib import Path
current_file_path = Path(__file__).resolve()
print("当前文件的绝对路径为:", current_file_path)
Path(__file__).resolve()
返回当前文件的绝对路径,效果与 os.path.abspath(__file__)
类似。
3. 获取当前文件的目录
通过 Path(__file__).resolve().parent
获取当前文件所在的目录。
from pathlib import Path
current_file_directory = Path(__file__).resolve().parent
print("当前文件所在的目录为:", current_file_directory)
Path(__file__).resolve().parent
返回当前文件所在的目录路径,效果与 os.path.dirname(os.path.abspath(__file__))
类似。
三、使用 inspect 模块检测文件路径
1. 获取当前文件的绝对路径
通过 inspect.getfile()
获取当前文件的绝对路径。
import inspect
import os
current_file_path = os.path.abspath(inspect.getfile(inspect.currentframe()))
print("当前文件的绝对路径为:", current_file_path)
inspect.getfile(inspect.currentframe())
返回当前文件的路径,结合 os.path.abspath()
可以得到绝对路径。
2. 获取当前文件的目录
通过 os.path.dirname()
获取当前文件所在的目录。
import inspect
import os
current_file_directory = os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe())))
print("当前文件所在的目录为:", current_file_directory)
os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe())))
返回当前文件所在的目录路径。
结论
在 Python3 中,检测当前文件路径的方法有多种,常见的包括使用 os 模块、pathlib 模块和 inspect 模块。使用 os 模块是最常见且简单的方法,可以通过 os.getcwd()
获取当前工作目录,os.path.abspath(__file__)
获取当前文件的绝对路径,os.path.dirname(os.path.abspath(__file__))
获取当前文件所在的目录。pathlib 模块提供了更面向对象的路径操作方法,可以通过 Path.cwd()
获取当前工作目录,Path(__file__).resolve()
获取当前文件的绝对路径,Path(__file__).resolve().parent
获取当前文件所在的目录。inspect 模块可以结合 os 模块获取当前文件的绝对路径和目录,通过 inspect.getfile(inspect.currentframe())
和 os.path.abspath()
获取当前文件的绝对路径,通过 os.path.dirname()
获取当前文件所在的目录。选择合适的方法可以帮助我们更方便地处理文件路径相关操作。
相关问答FAQs:
如何在Python3中获取当前工作目录?
可以使用os
模块中的getcwd()
函数来获取当前工作目录。示例如下:
import os
current_directory = os.getcwd()
print("当前工作目录是:", current_directory)
这段代码将打印出当前的工作目录路径,方便您进行后续的文件操作。
在Python3中,如何获取脚本所在的文件路径?
如果您想要获取正在执行的Python脚本的绝对路径,可以使用__file__
属性结合os.path
模块。以下是代码示例:
import os
script_path = os.path.abspath(__file__)
print("脚本所在路径是:", script_path)
此代码将返回正在执行的脚本的完整路径,便于您理解其位置。
是否可以在Python3中实时监控文件路径的变化?
是的,您可以使用watchdog
库来监控文件系统的变化,包括文件路径的变化。您需要先安装该库:
pip install watchdog
然后,可以创建一个监视器来检测特定目录的变化。示例如下:
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class MyHandler(FileSystemEventHandler):
def on_modified(self, event):
print(f"文件发生变化: {event.src_path}")
observer = Observer()
observer.schedule(MyHandler(), path='您要监控的目录路径', recursive=False)
observer.start()
这样,您就可以实时监控指定目录的文件变化。
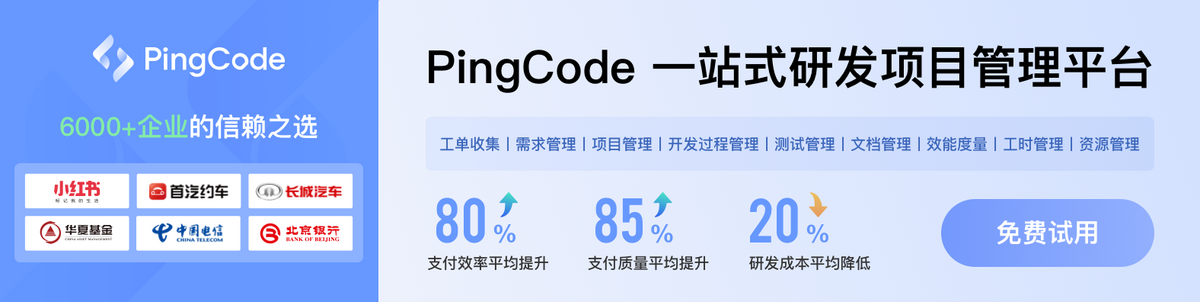