在Python中,改变输出内容的颜色主要通过使用第三方库来实现。常用的方法包括使用colorama
库、termcolor
库、以及ANSI转义序列。其中,colorama
库最为常见,它能够方便地跨平台实现终端文本着色。接下来,我将详细介绍如何使用colorama
库来改变输出内容的颜色。
一、安装与导入colorama
库
首先,需要安装colorama
库,可以通过以下命令进行安装:
pip install colorama
安装完成后,在Python脚本中导入colorama
库,并进行初始化:
import colorama
from colorama import Fore, Back, Style
colorama.init()
二、使用colorama
库设置前景色
colorama
库中的Fore
类可以用来设置文本的前景色。以下是几个常用颜色的示例:
print(Fore.RED + 'This is red text')
print(Fore.GREEN + 'This is green text')
print(Fore.BLUE + 'This is blue text')
print(Fore.YELLOW + 'This is yellow text')
三、使用colorama
库设置背景色
colorama
库中的Back
类可以用来设置文本的背景色。以下是几个常用背景色的示例:
print(Back.RED + 'This is text with red background')
print(Back.GREEN + 'This is text with green background')
print(Back.BLUE + 'This is text with blue background')
print(Back.YELLOW + 'This is text with yellow background')
四、使用colorama
库设置文本样式
colorama
库中的Style
类可以用来设置文本的样式,如加粗、变暗等。以下是几个常用样式的示例:
print(Style.BRIGHT + 'This is bright text')
print(Style.DIM + 'This is dim text')
print(Style.NORMAL + 'This is normal text')
五、重置颜色和样式
在设置了颜色和样式之后,可以使用colorama
库中的Style.RESET_ALL
来重置颜色和样式:
print(Fore.RED + Back.GREEN + 'This is red text with green background' + Style.RESET_ALL)
print('This is normal text')
六、综合示例
下面是一个综合示例,展示了如何使用colorama
库设置不同的前景色、背景色和样式:
import colorama
from colorama import Fore, Back, Style
colorama.init()
print(Fore.RED + 'This is red text')
print(Back.GREEN + 'This is text with green background')
print(Style.BRIGHT + 'This is bright text')
print(Fore.YELLOW + Back.BLUE + 'This is yellow text with blue background' + Style.RESET_ALL)
print('This is normal text')
七、使用termcolor
库
除了colorama
库,termcolor
库也是一个常用来改变输出内容颜色的库。以下是使用termcolor
库的示例:
安装termcolor
库
pip install termcolor
使用termcolor
库设置颜色
from termcolor import colored
print(colored('This is red text', 'red'))
print(colored('This is green text', 'green'))
print(colored('This is blue text', 'blue', 'on_white'))
print(colored('This is yellow text with red background', 'yellow', 'on_red'))
八、使用ANSI转义序列
在不使用第三方库的情况下,可以使用ANSI转义序列来改变输出内容的颜色。以下是几个示例:
print('\033[91m' + 'This is red text' + '\033[0m')
print('\033[92m' + 'This is green text' + '\033[0m')
print('\033[93m' + 'This is yellow text' + '\033[0m')
print('\033[94m' + 'This is blue text' + '\033[0m')
九、总结
通过以上介绍,我们了解了在Python中改变输出内容颜色的几种方法。colorama
库是最为推荐的选择,因为它支持跨平台,使用简单方便。此外,termcolor
库和ANSI转义序列也是实现文本着色的有效手段。根据实际需求选择合适的方法,可以帮助我们更好地实现终端文本的着色效果。
希望这篇文章能帮助你在Python编程中更好地使用颜色来增强终端输出的可读性和美观性。如果有任何问题,欢迎在评论区讨论。
相关问答FAQs:
如何在Python中使用ANSI转义码改变输出内容的颜色?
在Python中,可以使用ANSI转义码来改变终端输出文本的颜色。这些转义码以“\033”开头,后面跟随颜色代码。例如,红色文本可以通过“\033[31m”实现,重置颜色则使用“\033[0m”。示例代码如下:
print("\033[31m这是红色文本\033[0m")
是否可以使用第三方库来简化颜色输出?
是的,Python有多个第三方库可以简化颜色输出,比如colorama
和termcolor
。这些库提供了易于使用的接口,让你可以轻松设置文本颜色。例如,使用termcolor
库,可以通过以下方式实现:
from termcolor import colored
print(colored('这是蓝色文本', 'blue'))
在不同操作系统中,输出颜色是否会有所不同?
确实如此,ANSI转义码在类Unix系统(如Linux和macOS)中普遍支持,但在Windows命令提示符中可能需要额外配置。使用colorama
库可以解决这个问题,它会自动处理ANSI转义码在Windows上的兼容性。只需在程序开始时调用colorama.init()
即可。
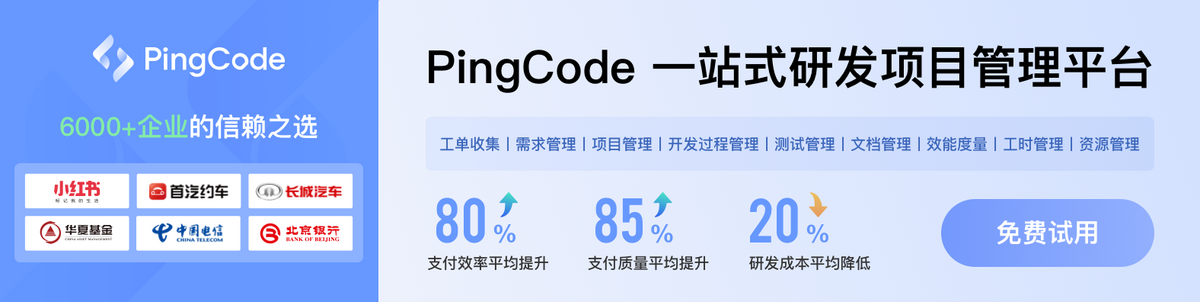