PYTHON中自定义的类如何使用
在Python中自定义的类可以通过定义类、创建类的实例、调用类的方法、访问类的属性来使用。 其中最重要的步骤是定义类和理解类的属性和方法。下面将详细介绍如何在Python中自定义类并使用。
一、定义类
定义类是创建自定义类的第一步。类是通过class
关键字定义的,类的名字通常首字母大写以便与函数名区分。类的基本结构如下:
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
def method1(self):
return self.attribute1
def method2(self):
return self.attribute2
在上面的例子中,MyClass
是类名,__init__
是初始化方法,它在创建类的实例时自动调用。self
参数是类方法的第一个参数,指向类的实例本身。
二、创建类的实例
创建类的实例是使用类的关键步骤之一。通过类名加上括号,并传递必要的参数来创建实例:
my_instance = MyClass('value1', 'value2')
这段代码创建了MyClass
的一个实例my_instance
,并传递了两个参数value1
和value2
给初始化方法__init__
。
三、调用类的方法
类的方法是定义在类中的函数,用于操作类的属性或执行某些功能。通过实例可以调用类的方法:
print(my_instance.method1()) # 输出: value1
print(my_instance.method2()) # 输出: value2
在这里,my_instance.method1()
调用了MyClass
类中的method1
方法,并返回attribute1
的值。
四、访问类的属性
类的属性是类中定义的变量,可以通过实例直接访问和修改:
print(my_instance.attribute1) # 输出: value1
my_instance.attribute1 = 'new_value1'
print(my_instance.attribute1) # 输出: new_value1
在这里,通过my_instance.attribute1
访问并修改了attribute1
的值。
深入理解与实战
一、类的继承
Python支持类的继承,可以创建一个新类,该新类继承自一个或多个现有类。继承允许我们复用代码,并创建更复杂的类结构。例如:
class ParentClass:
def __init__(self, attribute):
self.attribute = attribute
def parent_method(self):
return self.attribute
class ChildClass(ParentClass):
def child_method(self):
return 'child method'
在这个例子中,ChildClass
继承自ParentClass
,这意味着ChildClass
拥有ParentClass
的所有属性和方法。我们可以创建ChildClass
的实例并调用继承的方法:
child_instance = ChildClass('parent attribute')
print(child_instance.parent_method()) # 输出: parent attribute
print(child_instance.child_method()) # 输出: child method
二、重写方法
子类可以重写父类的方法,以提供不同的实现。例如:
class ParentClass:
def method(self):
return 'parent method'
class ChildClass(ParentClass):
def method(self):
return 'child method'
在这个例子中,ChildClass
重写了ParentClass
的method
方法:
child_instance = ChildClass()
print(child_instance.method()) # 输出: child method
三、类的多态性
多态性是面向对象编程的一个重要概念,它允许我们使用不同的类的对象调用相同的方法。例如:
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return 'Woof!'
class Cat(Animal):
def speak(self):
return 'Meow!'
在这个例子中,Dog
和Cat
类都实现了Animal
类的speak
方法。我们可以创建一个函数,它接受一个Animal
对象,并调用它的speak
方法:
def make_animal_speak(animal):
return animal.speak()
dog = Dog()
cat = Cat()
print(make_animal_speak(dog)) # 输出: Woof!
print(make_animal_speak(cat)) # 输出: Meow!
四、类的封装
封装是面向对象编程的另一个重要概念,它允许将数据和操作数据的方法封装在一起,并隐藏类的内部实现细节。通过使用双下划线前缀,我们可以将类的属性和方法设为私有:
class MyClass:
def __init__(self, attribute):
self.__attribute = attribute
def get_attribute(self):
return self.__attribute
def set_attribute(self, value):
self.__attribute = value
在这个例子中,__attribute
是一个私有属性,只能通过get_attribute
和set_attribute
方法访问和修改:
instance = MyClass('value')
print(instance.get_attribute()) # 输出: value
instance.set_attribute('new value')
print(instance.get_attribute()) # 输出: new value
五、类的组合
组合是将一个类的实例作为另一个类的属性,从而复用代码。例如:
class Engine:
def start(self):
return 'Engine started'
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
return self.engine.start()
在这个例子中,Car
类包含一个Engine
类的实例,并通过调用Engine
类的方法实现启动功能:
car = Car()
print(car.start()) # 输出: Engine started
六、类的静态方法和类方法
静态方法和类方法是类中的特殊方法,它们不需要类的实例即可调用。静态方法使用@staticmethod
装饰器定义,而类方法使用@classmethod
装饰器定义:
class MyClass:
@staticmethod
def static_method():
return 'static method'
@classmethod
def class_method(cls):
return 'class method'
在这个例子中,static_method
是一个静态方法,class_method
是一个类方法:
print(MyClass.static_method()) # 输出: static method
print(MyClass.class_method()) # 输出: class method
七、类的特殊方法
Python类可以定义一些特殊方法,以实现特定的功能。例如,__str__
方法用于定义对象的字符串表示,__eq__
方法用于定义对象的比较:
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
def __str__(self):
return f'MyClass(attribute={self.attribute})'
def __eq__(self, other):
return self.attribute == other.attribute
在这个例子中,__str__
方法返回对象的字符串表示,__eq__
方法比较两个对象的属性:
instance1 = MyClass('value')
instance2 = MyClass('value')
print(instance1) # 输出: MyClass(attribute=value)
print(instance1 == instance2) # 输出: True
通过这些步骤和概念,我们可以在Python中自定义类,并使用类来创建灵活、可复用的代码结构。理解和掌握这些概念,有助于提高代码的质量和可维护性。
相关问答FAQs:
如何创建一个自定义的Python类?
要创建一个自定义的Python类,您需要使用class
关键字。在类定义内部,您可以定义属性和方法。例如:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
return f"Hello, {self.name}!"
在这个例子中,MyClass
是一个自定义类,具有一个初始化方法和一个问候方法。
在自定义类中如何添加属性和方法?
自定义类可以包含多种属性和方法。属性通常在__init__
方法中定义,而方法则是类内的函数。可以通过self
关键字引用当前类的实例。例如:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def display_info(self):
return f"{self.brand} {self.model}"
在这个示例中,Car
类有brand
和model
属性,以及一个显示信息的方法。
如何在Python中实例化自定义类?
实例化自定义类非常简单,只需调用类名并传入所需的参数。使用__init__
方法初始化属性后,您可以使用实例访问属性和方法。例如:
my_car = Car("Toyota", "Corolla")
print(my_car.display_info()) # 输出: Toyota Corolla
这段代码创建了一个Car
类的实例,并调用display_info
方法来显示汽车信息。
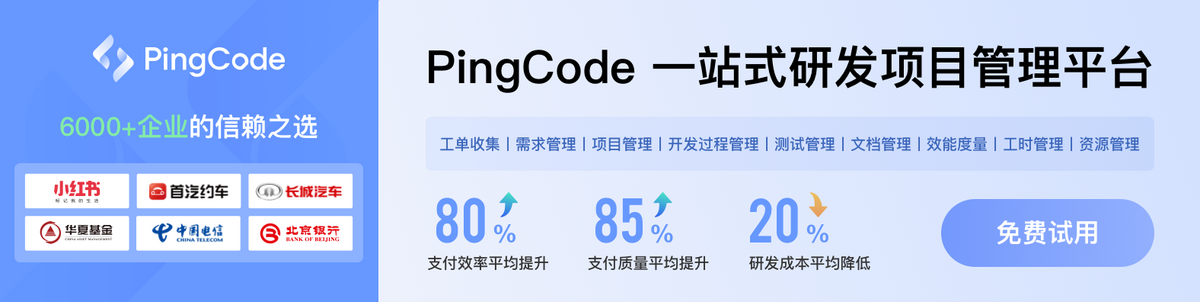