开头段落: 在Python中,可以通过字符串的内建方法、格式化字符串和使用正则表达式等方式来在字符串的后面添加空格。 最简单的方法是使用字符串拼接(concatenation),但是对于更多样化的需求,可以使用字符串的内建方法比如ljust()
,或者使用格式化字符串的方法。特别是ljust()
方法,它可以确保字符串达到指定的长度,并在必要时用空格填充。
例如,ljust()
方法的使用如下:
my_string = "Hello"
new_string = my_string.ljust(10)
print(f"'{new_string}'") # Output: 'Hello '
接下来,让我们深入探讨在Python中如何在字符串后面加空格的各种方法。
一、字符串拼接
字符串拼接是最直接的方式。使用加号+
将空格连接到字符串的末尾。
my_string = "Hello"
new_string = my_string + " "
print(f"'{new_string}'") # Output: 'Hello '
这种方法适用于简单情况,但如果需要添加更多空格或者需要确保字符串达到某个长度,可能会显得笨拙。
二、ljust() 方法
ljust()
方法是一个非常实用的内建字符串方法。它将字符串左对齐,并用指定的字符(默认为空格)填充右侧,直到达到指定的宽度。
my_string = "Hello"
new_string = my_string.ljust(10)
print(f"'{new_string}'") # Output: 'Hello '
这个方法非常方便,因为你只需要指定最终的长度,Python会自动填充空格。
使用示例
my_string = "Data"
new_string = my_string.ljust(8, '-')
print(f"'{new_string}'") # Output: 'Data----'
这种方式不仅限于空格,还可以使用其他字符来填充。
三、格式化字符串
格式化字符串提供了一种更灵活的方法来控制字符串的格式。Python有多种字符串格式化的方法,包括百分号%
格式化、str.format()
方法和f-strings(格式化字符串字面值)。
百分号格式化
my_string = "Hello"
new_string = "%-10s" % my_string
print(f"'{new_string}'") # Output: 'Hello '
str.format() 方法
my_string = "Hello"
new_string = "{:<10}".format(my_string)
print(f"'{new_string}'") # Output: 'Hello '
f-strings
my_string = "Hello"
new_string = f"{my_string:<10}"
print(f"'{new_string}'") # Output: 'Hello '
这些方法都可以非常方便地控制字符串的宽度和填充字符。
四、使用正则表达式
虽然正则表达式并不是最常用的方法,但它在处理复杂的字符串模式时非常有用。你可以使用re
模块来实现这一点。
import re
my_string = "Hello"
new_string = re.sub(r'$', ' ', my_string)
print(f"'{new_string}'") # Output: 'Hello '
在这个例子中,re.sub
函数用空格替换字符串末尾的位置。
五、循环添加空格
有时候你可能需要动态地添加空格。例如,基于某些条件来决定添加多少空格。这时可以使用循环。
my_string = "Hello"
spaces_to_add = 5
new_string = my_string + " " * spaces_to_add
print(f"'{new_string}'") # Output: 'Hello '
这种方法虽然不如ljust()
或格式化字符串简洁,但在动态情况下非常有用。
六、使用列表和join()
另一种灵活的方法是使用列表和join()
方法来生成所需的字符串。
my_string = "Hello"
spaces_to_add = 5
new_string = ''.join([my_string] + [' ' * spaces_to_add])
print(f"'{new_string}'") # Output: 'Hello '
这种方法虽然有点复杂,但提供了另一种处理字符串的思路。
七、使用字符串模板
Python的string
模块提供了一个Template
类,可以用来替换占位符。这在处理更加复杂的字符串格式时非常有用。
from string import Template
t = Template('$s ')
new_string = t.substitute(s="Hello")
print(f"'{new_string}'") # Output: 'Hello '
这种方法对于需要动态替换多个占位符的情况非常有用。
八、使用外部库
在一些高级应用场景下,你可能需要使用外部库来处理字符串。例如,textwrap
模块可以帮助你处理更加复杂的字符串格式。
import textwrap
my_string = "Hello"
width = 10
new_string = textwrap.fill(my_string, width=width)
print(f"'{new_string}'") # Output: 'Hello '
这种方法对于格式化段落和处理多行字符串非常有用。
结论
在Python中,有许多方法可以在字符串的后面添加空格,从简单的字符串拼接到使用内建方法ljust()
,再到格式化字符串和正则表达式。每种方法都有其适用的场景和优缺点。对于大多数情况,使用ljust()
方法是最方便和高效的。但是,根据具体需求,其他方法也有其独特的优势。通过掌握这些技巧,你可以更加灵活和高效地处理字符串。
相关问答FAQs:
如何在Python字符串末尾添加空格?
在Python中,可以使用字符串的拼接功能来在字符串末尾添加空格。例如,您可以通过简单的加法运算符将空格字符串与现有字符串连接起来:my_string = "Hello" + " "
。此外,使用str.ljust()
方法也可以达到相似效果,这个方法可以将字符串扩展到指定的宽度,并在右侧填充空格。
在Python中添加多个空格的最佳方法是什么?
如果您希望在字符串末尾添加多个空格,可以使用乘法运算符。例如,my_string = "Hello" + " " * 5
将会在“Hello”后添加5个空格。您也可以使用str.ljust()
方法来指定最终字符串的总长度,从而添加所需的空格。
如何在打印输出时在字符串后添加空格?
在打印字符串时,可以直接在字符串后添加空格。例如,print("Hello", end=" ")
将“Hello”打印后,保持行不换行,并在末尾添加五个空格。这样可以使输出更易于阅读,特别是在需要格式化多行输出时。
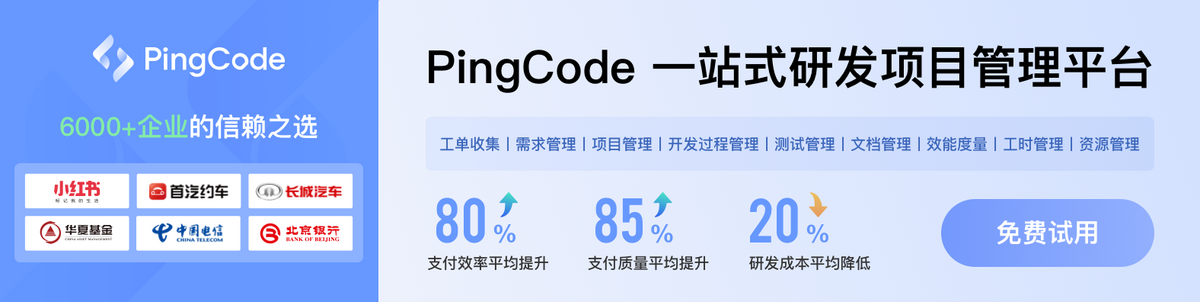