Python从字符串中提取数字的方法包括使用正则表达式、字符串操作方法、列表推导等。在这些方法中,使用正则表达式是一种非常强大且灵活的方式。 例如,可以使用 re.findall()
方法从字符串中提取所有的数字。接下来将详细介绍如何使用这些方法来提取字符串中的数字。
一、使用正则表达式提取数字
正则表达式(Regular Expressions, regex)是一种强大的工具,用于匹配字符串中的特定模式。Python的 re
模块提供了正则表达式的支持,可以非常方便地从字符串中提取数字。
安装和导入re模块
首先,需要确保已经导入 re
模块。这个模块是Python标准库的一部分,所以不需要额外安装。
import re
使用re.findall()方法
要从字符串中提取所有的数字,可以使用 re.findall()
方法。这个方法会返回一个包含所有匹配项的列表。
text = "There are 2 apples for 4 people"
numbers = re.findall(r'\d+', text)
print(numbers) # 输出: ['2', '4']
在这个例子中,正则表达式 \d+
匹配一个或多个数字字符,所以 re.findall()
会返回字符串中的所有数字。
提取数字并转换为整数
提取的数字默认是字符串形式,如果需要将其转换为整数,可以使用列表推导。
numbers = [int(num) for num in re.findall(r'\d+', text)]
print(numbers) # 输出: [2, 4]
提取浮点数
如果字符串中包含浮点数,可以使用更复杂的正则表达式来匹配。
text = "The temperature is 23.5 degrees, but it feels like 21.8"
numbers = re.findall(r'\d+\.\d+', text)
print(numbers) # 输出: ['23.5', '21.8']
同样,可以使用列表推导将这些浮点数转换为浮点类型。
numbers = [float(num) for num in re.findall(r'\d+\.\d+', text)]
print(numbers) # 输出: [23.5, 21.8]
二、使用字符串操作方法
虽然正则表达式是非常强大的工具,但有时使用简单的字符串操作方法也可以完成任务。下面介绍几种常见的方法。
使用str.isdigit()
可以迭代字符串的每一个字符,检查它是否是数字。
text = "There are 2 apples for 4 people"
numbers = [int(char) for char in text if char.isdigit()]
print(numbers) # 输出: [2, 4]
使用str.split()和列表推导
如果数字和其他字符之间有明确的分隔符,可以使用 split()
方法。
text = "There are 2 apples for 4 people"
words = text.split()
numbers = [int(word) for word in words if word.isdigit()]
print(numbers) # 输出: [2, 4]
三、使用列表推导和条件判断
列表推导和条件判断可以结合使用,以实现从字符串中提取数字的目的。
提取整数
可以使用内置函数 filter()
和 str.isdigit()
方法。
text = "There are 2 apples for 4 people"
numbers = list(filter(str.isdigit, text))
numbers = list(map(int, numbers))
print(numbers) # 输出: [2, 4]
提取浮点数
可以先使用 split()
方法将字符串分割成单词,然后检查每个单词是否是浮点数。
text = "The temperature is 23.5 degrees, but it feels like 21.8"
words = text.split()
numbers = [float(word) for word in words if re.match(r'^\d+\.\d+$', word)]
print(numbers) # 输出: [23.5, 21.8]
四、结合不同方法进行复杂提取
有时候,单一的方法可能无法满足需求,需要结合多种方法进行提取。
提取混合类型的数字
假设字符串中既有整数又有浮点数,可以结合使用正则表达式和条件判断。
text = "I have 2 apples and 3.5 oranges"
matches = re.findall(r'\d+\.\d+|\d+', text)
numbers = [float(num) if '.' in num else int(num) for num in matches]
print(numbers) # 输出: [2, 3.5]
提取负数
如果字符串中包含负数,可以使用带负号的正则表达式。
text = "The stock prices changed by -2, 3, and -4.5 points"
matches = re.findall(r'-?\d+\.?\d*', text)
numbers = [float(num) if '.' in num else int(num) for num in matches]
print(numbers) # 输出: [-2, 3, -4.5]
五、处理复杂的字符串格式
有时候字符串格式非常复杂,需要更精细的处理方式。下面是一些处理复杂字符串格式的建议。
自定义函数处理字符串
可以编写自定义函数,结合正则表达式和字符串操作方法,来处理复杂的字符串格式。
def extract_numbers(text):
matches = re.findall(r'-?\d+\.?\d*', text)
numbers = [float(num) if '.' in num else int(num) for num in matches]
return numbers
text = "The total cost is -123.45 dollars, and the discount is -10%"
numbers = extract_numbers(text)
print(numbers) # 输出: [-123.45, -10]
使用生成器处理大文本
如果需要处理非常大的文本,可以使用生成器来提高效率。
def extract_numbers_gen(text):
for match in re.finditer(r'-?\d+\.?\d*', text):
num = match.group()
yield float(num) if '.' in num else int(num)
text = "The stock prices changed by -2, 3, and -4.5 points"
numbers = list(extract_numbers_gen(text))
print(numbers) # 输出: [-2, 3, -4.5]
综上所述,Python提供了多种方法从字符串中提取数字,包括正则表达式、字符串操作方法和列表推导等。根据具体需求,可以选择适合的方法来完成任务。如果字符串格式较为复杂,可以结合多种方法,并考虑编写自定义函数或使用生成器来提高处理效率。通过掌握这些技巧,可以在实际项目中更加灵活地处理字符串中的数字提取问题。
相关问答FAQs:
如何在Python中从字符串中提取所有数字?
在Python中,可以使用正则表达式来提取字符串中的所有数字。通过re
模块,可以使用re.findall()
函数来找到所有符合条件的数字。例如,re.findall(r'\d+', my_string)
将返回字符串中所有的数字,作为一个列表输出。
是否可以从字符串中提取浮点数和整数?
是的,使用正则表达式可以同时提取整数和浮点数。可以使用模式r'\d+\.?\d*'
来匹配整数和带小数点的浮点数。这种方式确保你能获取到所有形式的数字。
在提取数字后如何将它们转换为整数或浮点数?
提取后,返回的数字通常为字符串格式。可以使用int()
函数将字符串转换为整数,或使用float()
函数将其转换为浮点数。例如,numbers = [int(num) for num in re.findall(r'\d+', my_string)]
将提取的数字转换为整数列表。
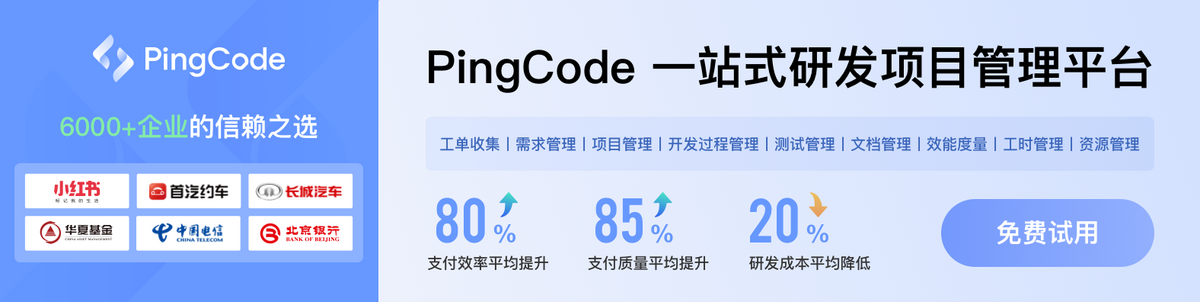