在Python中查找对应的字符串可以使用find()方法、index()方法、正则表达式、str.contains()方法。这些方法各有特点和适用场景。本文将详细介绍这些方法的使用,帮助你在不同情况下选择合适的字符串查找方法。
一、使用find()方法
find()方法是Python字符串对象的一个方法,用于查找子字符串在字符串中的位置。它返回子字符串在字符串中第一次出现的位置,如果找不到子字符串,则返回-1。
1、基本用法
text = "Hello, welcome to the world of Python"
substring = "Python"
position = text.find(substring)
print(f"The position of '{substring}' in the text is: {position}")
2、使用start和end参数
find()方法还可以接受start和end参数,指定查找的范围。
text = "Hello, welcome to the world of Python"
substring = "o"
position = text.find(substring, 10, 20)
print(f"The position of '{substring}' in the text from index 10 to 20 is: {position}")
二、使用index()方法
index()方法与find()方法类似,但不同之处在于,如果找不到子字符串,index()方法会引发ValueError异常。
1、基本用法
text = "Hello, welcome to the world of Python"
substring = "Python"
try:
position = text.index(substring)
print(f"The position of '{substring}' in the text is: {position}")
except ValueError:
print(f"'{substring}' not found in the text")
2、使用start和end参数
index()方法同样可以接受start和end参数,指定查找的范围。
text = "Hello, welcome to the world of Python"
substring = "o"
try:
position = text.index(substring, 10, 20)
print(f"The position of '{substring}' in the text from index 10 to 20 is: {position}")
except ValueError:
print(f"'{substring}' not found in the text from index 10 to 20")
三、使用正则表达式
正则表达式(Regular Expressions)是一种强大的文本处理工具,适用于复杂的字符串查找和匹配操作。在Python中,可以使用re模块来处理正则表达式。
1、基本用法
import re
text = "Hello, welcome to the world of Python"
pattern = re.compile(r"Python")
match = pattern.search(text)
if match:
print(f"The position of 'Python' in the text is: {match.start()}")
else:
print("'Python' not found in the text")
2、查找所有匹配项
import re
text = "Hello, welcome to the world of Python. Python is great!"
pattern = re.compile(r"Python")
matches = pattern.findall(text)
print(f"Found {len(matches)} occurrences of 'Python' in the text")
四、使用str.contains()方法
在处理数据分析时,Pandas库中的str.contains()方法可以用于字符串查找。它返回一个布尔值,指示每个元素是否包含指定的子字符串。
1、基本用法
import pandas as pd
data = pd.Series(["Hello, welcome to the world of Python", "Python is great!", "Hello, world!"])
contains_python = data.str.contains("Python")
print(contains_python)
五、使用in关键字
in关键字可以直接用于检查子字符串是否存在于字符串中,返回一个布尔值。
1、基本用法
text = "Hello, welcome to the world of Python"
substring = "Python"
if substring in text:
print(f"'{substring}' is found in the text")
else:
print(f"'{substring}' is not found in the text")
六、使用字符串切片
字符串切片可以用来获取字符串的子字符串,并结合上述方法进行查找。
1、基本用法
text = "Hello, welcome to the world of Python"
substring = text[7:14]
print(f"The substring from index 7 to 14 is: '{substring}'")
七、总结
在Python中查找字符串的方法有很多,选择合适的方法取决于具体的需求和场景。find()方法、index()方法、正则表达式、str.contains()方法、in关键字、字符串切片都是常用的方法。理解这些方法的特点和使用方法,可以帮助你更高效地处理字符串查找任务。
在实际应用中,建议根据字符串的复杂度和查找需求选择合适的方法。例如,对于简单的查找操作,可以使用find()方法或in关键字;对于复杂的模式匹配,可以使用正则表达式;在数据分析中,可以使用Pandas库中的str.contains()方法。希望本文能帮助你更好地理解和应用这些方法,提高字符串处理效率。
相关问答FAQs:
如何在Python中进行字符串查找?
在Python中,可以使用多种方法来查找字符串。最常用的是使用str.find()
和str.index()
方法。find()
方法返回子字符串的最低索引,如果找不到则返回-1,而index()
方法在找不到时会引发一个ValueError
。另外,使用in
运算符也可以快速检查一个字符串是否包含另一个字符串。
有没有更高效的方法来查找大型字符串中的子字符串?
对于较大的字符串,使用正则表达式(re
模块)可以提供更灵活的查找功能。例如,re.search()
方法可以用来查找符合特定模式的字符串,从而提高查找效率和准确性。正则表达式的使用使得匹配复杂模式成为可能,如忽略大小写或匹配多个字符。
如何处理字符串查找中的大小写敏感性?
在Python中,字符串查找是区分大小写的。如果需要进行不区分大小写的查找,可以将两个字符串都转换为小写或大写,再进行比较。例如,可以使用str.lower()
或str.upper()
方法来统一大小写后再进行查找。对于正则表达式,可以使用re.IGNORECASE
标志来实现不区分大小写的匹配。
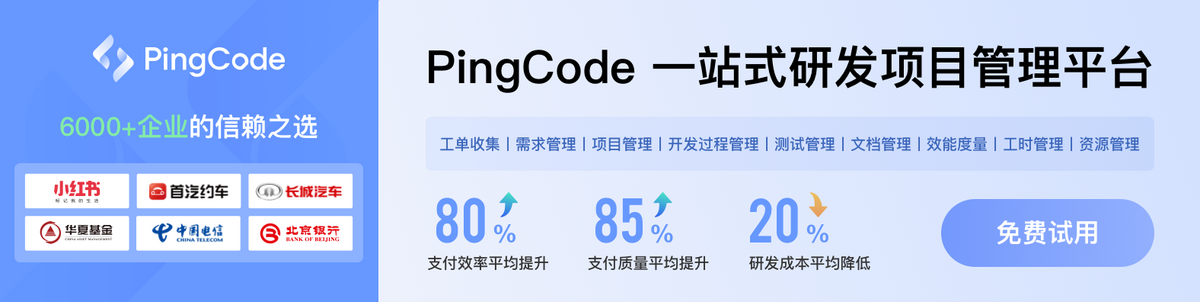