Python解析网页内容的主要方法包括:使用requests库获取网页内容、使用BeautifulSoup库解析HTML、使用lxml库解析HTML、使用Selenium模拟浏览器操作。 其中,requests库和BeautifulSoup库的组合是最常见的方案,因为其简单易用且功能强大。下面将详细介绍这两者的用法。
一、使用requests库获取网页内容
1、安装requests库
首先,我们需要安装requests库。你可以使用以下命令进行安装:
pip install requests
2、使用requests库获取网页内容
使用requests库获取网页内容非常简单。只需几行代码就能实现:
import requests
url = 'https://www.example.com'
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
print(response.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
上述代码中,我们首先导入requests库,然后使用requests.get
方法获取网页内容。如果请求成功(状态码为200),则输出网页内容。
二、使用BeautifulSoup库解析HTML
1、安装BeautifulSoup库
BeautifulSoup库需要与lxml或html.parser解析器结合使用。你可以使用以下命令安装:
pip install beautifulsoup4 lxml
2、使用BeautifulSoup库解析HTML
使用BeautifulSoup库解析HTML内容也非常简单。以下是一个基本示例:
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
print(soup.prettify())
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在上述代码中,我们首先使用requests库获取网页内容,然后将内容传递给BeautifulSoup对象进行解析。最后,我们使用soup.prettify()
方法输出格式化的HTML内容。
三、使用lxml库解析HTML
1、安装lxml库
lxml库是一个非常强大的HTML解析库。你可以使用以下命令进行安装:
pip install lxml
2、使用lxml库解析HTML
以下是一个使用lxml库解析HTML的示例:
from lxml import html
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
tree = html.fromstring(response.content)
print(html.tostring(tree, pretty_print=True).decode())
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在上述代码中,我们首先使用requests库获取网页内容,然后将内容传递给lxml的html.fromstring
方法进行解析。最后,我们使用html.tostring
方法输出格式化的HTML内容。
四、使用Selenium模拟浏览器操作
1、安装Selenium和浏览器驱动
Selenium是一个强大的工具,可以用来模拟浏览器操作。你可以使用以下命令安装Selenium:
pip install selenium
此外,你还需要下载相应的浏览器驱动(如ChromeDriver或GeckoDriver),并将其路径添加到系统环境变量中。
2、使用Selenium模拟浏览器操作
以下是一个使用Selenium获取网页内容的示例:
from selenium import webdriver
url = 'https://www.example.com'
driver = webdriver.Chrome() # 或者使用webdriver.Firefox()等其他浏览器
driver.get(url)
html_content = driver.page_source
print(html_content)
driver.quit()
在上述代码中,我们首先使用Selenium创建一个浏览器实例,然后使用driver.get
方法打开指定的网页,最后获取网页内容并输出。
五、结合使用requests和BeautifulSoup库
结合使用requests和BeautifulSoup库是解析网页内容的最佳实践。以下是一个完整的示例:
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
# 查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
# 查找特定的元素
title = soup.find('title')
print(f"Title: {title.string}")
# 查找特定的类或ID的元素
specific_div = soup.find('div', {'class': 'specific-class'})
print(specific_div.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在上述代码中,我们首先使用requests库获取网页内容,然后使用BeautifulSoup库解析HTML内容。接着,我们展示了如何查找所有链接、查找特定元素以及查找特定类或ID的元素。
六、处理JavaScript生成的内容
有些网页的内容是由JavaScript生成的,使用requests和BeautifulSoup库可能无法直接获取这些内容。在这种情况下,我们可以使用Selenium或其他工具来模拟浏览器操作。
以下是一个使用Selenium处理JavaScript生成内容的示例:
from selenium import webdriver
url = 'https://www.example.com'
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'lxml')
查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
driver.quit()
在上述代码中,我们使用Selenium模拟浏览器打开网页,获取完整的HTML内容,然后使用BeautifulSoup库解析HTML内容。
七、处理动态加载的内容
对于一些动态加载的内容(如通过AJAX请求加载的数据),我们可以使用requests库直接请求这些数据的API接口,或者使用Selenium等待页面完全加载后再获取内容。
以下是一个使用requests库请求API接口的示例:
import requests
api_url = 'https://www.example.com/api/data'
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Failed to retrieve the data. Status code: {response.status_code}")
在上述代码中,我们直接请求API接口获取数据,并将数据解析为JSON格式。
八、使用正则表达式提取内容
在某些情况下,我们可能需要使用正则表达式从HTML内容中提取特定信息。以下是一个使用正则表达式提取内容的示例:
import re
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
# 提取所有链接
links = re.findall(r'href="(.*?)"', html_content)
for link in links:
print(link)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在上述代码中,我们使用正则表达式从HTML内容中提取所有链接。
九、处理编码问题
在解析网页内容时,可能会遇到编码问题。以下是一个处理编码问题的示例:
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
response.encoding = response.apparent_encoding
print(response.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在上述代码中,我们使用response.apparent_encoding
来自动检测网页的编码,并设置response.encoding
以确保正确解析网页内容。
十、处理反爬虫机制
有些网站会有反爬虫机制,限制频繁请求或检测爬虫行为。以下是一些常见的反爬虫机制及应对方法:
1、设置请求头
设置请求头可以模拟浏览器请求,减少被识别为爬虫的风险:
import requests
url = 'https://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(response.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
2、使用代理
使用代理可以隐藏真实IP地址,绕过IP限制:
import requests
url = 'https://www.example.com'
proxies = {
'http': 'http://your-proxy-ip:port',
'https': 'https://your-proxy-ip:port'
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
print(response.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
3、添加延迟
添加请求间隔,避免频繁请求触发反爬虫机制:
import time
import requests
url = 'https://www.example.com'
for i in range(5): # 示例:请求5次
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
time.sleep(2) # 每次请求间隔2秒
总结
通过上述内容,我们详细介绍了使用Python解析网页内容的几种主要方法,包括使用requests库获取网页内容、使用BeautifulSoup库解析HTML、使用lxml库解析HTML、使用Selenium模拟浏览器操作、处理JavaScript生成的内容、处理动态加载的内容、使用正则表达式提取内容、处理编码问题以及应对反爬虫机制。希望这些内容能够帮助你更好地理解和应用Python进行网页内容解析。
相关问答FAQs:
如何使用Python提取网页数据?
要提取网页数据,您可以使用Python中的库如BeautifulSoup和requests。首先,使用requests库发送HTTP请求获取网页内容,然后用BeautifulSoup解析HTML。解析后,您可以使用各种方法选择和提取所需的数据。
在解析网页时需要注意哪些事项?
解析网页时,确保遵循网站的robots.txt文件中的爬虫规则,尊重网站的使用条款。此外,处理动态加载的内容时,可能需要使用Selenium等工具,因为某些数据通过JavaScript异步加载。
解析网页内容后,如何保存提取的数据?
提取的数据可以保存为多种格式,例如CSV、JSON或直接存入数据库。使用Python的pandas库可以很方便地将数据导出为CSV文件,或者使用json模块将数据序列化为JSON格式,满足不同的需求。
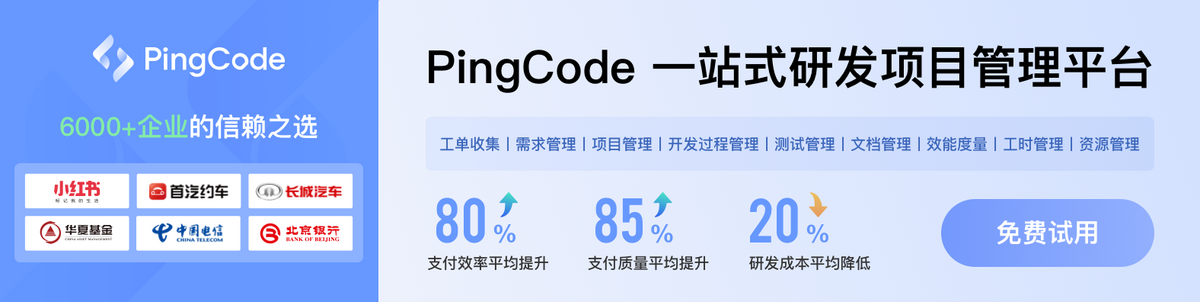