在 Python 类中调用多线程的方法有以下几种:使用 threading 模块、使用 concurrent.futures 模块、注意线程安全。 以下是对如何使用 threading 模块的详细描述。
在 Python 中,threading
模块提供了一个简单的接口来创建和管理线程。要在类中调用多线程的方法,可以通过定义类的方法,然后在这些方法中使用 threading.Thread
来启动多线程任务。下面将详细介绍如何在 Python 类中使用多线程,并提供示例代码。
一、使用 threading 模块
在 Python 中,threading
模块是最常用的多线程库。通过这个模块,可以方便地创建和管理线程。下面是一个基本示例,展示了如何在类中使用 threading
模块来调用多线程方法。
示例代码
import threading
import time
class MyClass:
def __init__(self):
self.data = []
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
self.data.append(thread_id)
def run_threads(self):
threads = []
for i in range(5):
thread = threading.Thread(target=self.worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads finished.")
print("Collected data:", self.data)
if __name__ == "__main__":
my_class = MyClass()
my_class.run_threads()
详细解释
-
创建类和初始化方法:
class MyClass:
def __init__(self):
self.data = []
在类的初始化方法中,创建一个空列表
self.data
,用于存储线程完成时的数据。 -
定义工作方法:
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
self.data.append(thread_id)
这个方法将被多个线程调用。它接收一个
thread_id
参数,用于标识线程。方法中打印线程开始和结束的消息,并将thread_id
添加到self.data
列表中。 -
运行多线程的方法:
def run_threads(self):
threads = []
for i in range(5):
thread = threading.Thread(target=self.worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads finished.")
print("Collected data:", self.data)
在这个方法中,创建多个线程并启动它们。首先,创建一个空列表
threads
来存储线程对象。然后,使用一个循环创建和启动多个线程。每个线程调用worker
方法,并传递一个唯一的thread_id
。最后,使用thread.join()
方法等待所有线程完成。
二、使用 concurrent.futures 模块
concurrent.futures
模块提供了一个更高级的接口来创建和管理线程。可以使用 ThreadPoolExecutor
来管理线程池,并异步执行任务。下面是一个示例,展示了如何在类中使用 concurrent.futures
模块来调用多线程方法。
示例代码
from concurrent.futures import ThreadPoolExecutor
import time
class MyClass:
def __init__(self):
self.data = []
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
self.data.append(thread_id)
def run_threads(self):
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(self.worker, i) for i in range(5)]
for future in futures:
future.result()
print("All threads finished.")
print("Collected data:", self.data)
if __name__ == "__main__":
my_class = MyClass()
my_class.run_threads()
详细解释
-
导入模块和定义类:
from concurrent.futures import ThreadPoolExecutor
import time
class MyClass:
def __init__(self):
self.data = []
导入
ThreadPoolExecutor
模块,并定义类和初始化方法。 -
定义工作方法:
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
self.data.append(thread_id)
这个方法与前面的示例相同,接收一个
thread_id
参数,并将其添加到self.data
列表中。 -
运行多线程的方法:
def run_threads(self):
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(self.worker, i) for i in range(5)]
for future in futures:
future.result()
print("All threads finished.")
print("Collected data:", self.data)
在这个方法中,使用
ThreadPoolExecutor
创建一个线程池,并异步执行任务。首先,使用ThreadPoolExecutor(max_workers=5)
创建一个线程池,最多允许 5 个线程同时运行。然后,使用executor.submit(self.worker, i)
提交任务,并将返回的Future
对象存储在futures
列表中。最后,使用future.result()
等待所有任务完成。
三、注意线程安全
在多线程环境中,访问和修改共享数据时需要注意线程安全问题。可以使用 threading.Lock
来确保线程安全。下面是一个示例,展示了如何在类中使用 threading.Lock
来确保线程安全。
示例代码
import threading
import time
class MyClass:
def __init__(self):
self.data = []
self.lock = threading.Lock()
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
with self.lock:
self.data.append(thread_id)
def run_threads(self):
threads = []
for i in range(5):
thread = threading.Thread(target=self.worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads finished.")
print("Collected data:", self.data)
if __name__ == "__main__":
my_class = MyClass()
my_class.run_threads()
详细解释
-
创建锁对象:
class MyClass:
def __init__(self):
self.data = []
self.lock = threading.Lock()
在类的初始化方法中,创建一个
threading.Lock
对象self.lock
。 -
使用锁对象保护共享数据:
def worker(self, thread_id):
print(f"Thread {thread_id} starting")
time.sleep(2)
print(f"Thread {thread_id} finishing")
with self.lock:
self.data.append(thread_id)
在工作方法中,使用
with self.lock
上下文管理器来保护对self.data
列表的访问。这样可以确保只有一个线程可以在同一时间访问和修改self.data
列表。 -
运行多线程的方法:
def run_threads(self):
threads = []
for i in range(5):
thread = threading.Thread(target=self.worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads finished.")
print("Collected data:", self.data)
这个方法与前面的示例相同,创建和启动多个线程,并等待所有线程完成。
通过以上示例,我们可以看到在 Python 类中使用多线程的方法。根据需求,可以选择使用 threading
模块或 concurrent.futures
模块来创建和管理线程。同时,注意线程安全问题,使用 threading.Lock
来保护共享数据。希望这些示例和详细解释能够帮助你更好地理解和使用 Python 中的多线程技术。
相关问答FAQs:
在Python类中如何定义一个多线程方法?
在Python类中定义多线程方法时,可以使用threading
模块。首先,创建一个类并在其中定义一个需要执行多线程的实例方法。在方法内,可以创建一个或多个线程实例,并使用start()
方法启动它们。例如:
import threading
class MyClass:
def my_method(self):
thread1 = threading.Thread(target=self.thread_task)
thread2 = threading.Thread(target=self.thread_task)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
def thread_task(self):
# 执行一些任务
print("Thread is running")
在多线程中如何共享数据?
共享数据可以通过类的实例变量实现。然而,需注意线程安全问题。为了避免竞争条件,建议使用threading.Lock()
来锁定共享资源。例如:
class MyClass:
def __init__(self):
self.lock = threading.Lock()
self.shared_data = 0
def thread_task(self):
with self.lock:
# 安全地访问和修改共享数据
self.shared_data += 1
如何处理多线程中的异常?
在多线程环境中,异常处理非常重要。可以在每个线程的目标函数中使用try...except
块来捕获异常。这能确保即使一个线程发生错误,其他线程也能正常运行。例如:
def thread_task(self):
try:
# 执行可能引发异常的代码
except Exception as e:
print(f"An error occurred: {e}")
通过这种方式,您可以确保多线程操作的稳定性和可靠性。
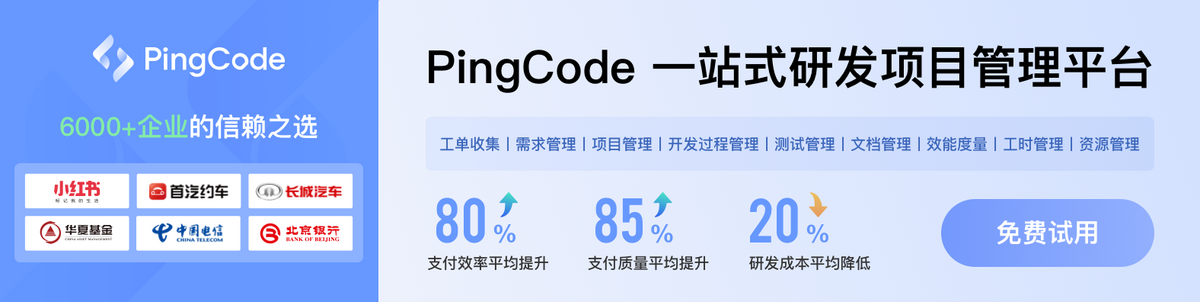