在Python中,判断一个字符串是否包含某个字符,可以使用以下几种方法:使用'in'运算符、使用find()方法、使用index()方法、使用正则表达式。 推荐使用'in'运算符,因为它最简单且可读性最好。以下是详细的解释和示例:
一、使用'in'运算符:
'in'运算符是Python中最简单和最直接的方法来判断一个字符串是否包含某个字符。你只需要使用'in'关键字来检查字符是否存在于字符串中,返回True或False。
# 示例代码
text = "Hello, World!"
char = 'o'
if char in text:
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
二、使用find()方法:
find()方法返回字符串中指定字符的第一个出现位置的索引,如果未找到则返回-1。
# 示例代码
text = "Hello, World!"
char = 'o'
index = text.find(char)
if index != -1:
print(f"'{char}' is present in the text at index {index}.")
else:
print(f"'{char}' is not present in the text.")
三、使用index()方法:
index()方法与find()方法类似,但如果字符未找到,则会抛出ValueError异常。
# 示例代码
text = "Hello, World!"
char = 'o'
try:
index = text.index(char)
print(f"'{char}' is present in the text at index {index}.")
except ValueError:
print(f"'{char}' is not present in the text.")
四、使用正则表达式:
正则表达式(Regular Expressions)是一种强大的字符串处理工具。使用re模块可以在字符串中搜索模式。
import re
示例代码
text = "Hello, World!"
char = 'o'
if re.search(char, text):
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
一、使用'in'运算符
'in'运算符是Python中最简洁和直观的方法之一来判断一个字符串是否包含某个字符。它不仅语法简单,而且执行效率高,适合大多数情况下使用。
示例代码
text = "Hello, World!"
char = 'o'
if char in text:
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,'o' in text
操作返回True
,因为字符'o'确实存在于字符串"Hello, World!"中。这个方法的优势在于其简洁性和可读性,非常适合代码阅读和维护。
二、使用find()方法
find()方法是一种更灵活的方法,它不仅可以检查字符是否存在,还可以返回字符在字符串中的位置。如果字符未找到,find()方法返回-1。
示例代码
text = "Hello, World!"
char = 'o'
index = text.find(char)
if index != -1:
print(f"'{char}' is present in the text at index {index}.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,find()方法返回字符'o'在字符串中的第一个出现位置的索引。这个方法适用于需要知道字符具体位置的情况。
三、使用index()方法
index()方法与find()方法类似,但有一个重要区别:如果字符未找到,index()方法会抛出ValueError异常。这使得它在某些情况下更适合使用,例如当你希望程序立即中断并处理错误时。
示例代码
text = "Hello, World!"
char = 'o'
try:
index = text.index(char)
print(f"'{char}' is present in the text at index {index}.")
except ValueError:
print(f"'{char}' is not present in the text.")
在这个例子中,index()方法会返回字符'o'在字符串中的位置,如果字符不存在则会抛出异常。这种方法适用于需要严格错误处理的情况。
四、使用正则表达式
正则表达式是一种强大的工具,用于字符串模式匹配。虽然正则表达式比其他方法略显复杂,但它提供了极大的灵活性和功能。
示例代码
import re
text = "Hello, World!"
char = 'o'
if re.search(char, text):
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,re.search(char, text)
用于搜索字符'o'在字符串中的位置。如果找到匹配的字符,返回一个Match对象,否则返回None。正则表达式适用于复杂的模式匹配需求。
五、使用count()方法
count()方法返回字符在字符串中出现的次数。如果返回值大于0,则说明字符串包含该字符。
示例代码
text = "Hello, World!"
char = 'o'
count = text.count(char)
if count > 0:
print(f"'{char}' is present in the text {count} times.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,count()方法用于统计字符'o'在字符串中出现的次数。这个方法不仅可以判断字符是否存在,还可以统计其出现的次数,适用于需要统计字符频率的场景。
六、使用any()函数与生成器表达式
any()函数结合生成器表达式也是一种优雅的方法,可以在不需要显式循环的情况下检查字符是否存在。
示例代码
text = "Hello, World!"
char = 'o'
if any(c == char for c in text):
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,生成器表达式(c == char for c in text)
用于生成一个布尔值序列,any()函数用于检查序列中是否有任意一个值为True。这种方法适用于需要快速检查多个条件的场景。
七、使用列表解析
列表解析是Python中一种简洁而强大的工具,可以用于创建列表。同样,它也可以用于检查字符是否存在。
示例代码
text = "Hello, World!"
char = 'o'
exists = [c for c in text if c == char]
if exists:
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,列表解析[c for c in text if c == char]
用于生成一个包含所有匹配字符的列表。如果列表非空,则说明字符存在。这种方法适用于需要将匹配字符收集起来的场景。
八、使用set()集合操作
集合(set)是Python中的一种数据结构,支持高效的成员测试。将字符串转换为集合可以快速判断字符是否存在。
示例代码
text = "Hello, World!"
char = 'o'
if char in set(text):
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,将字符串转换为集合set(text)
,然后使用'in'运算符检查字符是否存在。这种方法适用于需要进行大量成员测试的场景。
九、使用filter()函数
filter()函数是Python中的内置函数,用于过滤序列中的元素。结合lambda表达式,它可以用于检查字符是否存在。
示例代码
text = "Hello, World!"
char = 'o'
filtered = list(filter(lambda x: x == char, text))
if filtered:
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,filter()函数用于过滤出所有匹配的字符,返回一个迭代器。将其转换为列表后,如果列表非空,则说明字符存在。这种方法适用于需要过滤和处理字符的场景。
十、使用自定义函数
最后,您还可以编写一个自定义函数来封装上述逻辑,以便在项目中复用。
示例代码
def contains_char(text, char):
return char in text
text = "Hello, World!"
char = 'o'
if contains_char(text, char):
print(f"'{char}' is present in the text.")
else:
print(f"'{char}' is not present in the text.")
在这个例子中,自定义函数contains_char()
封装了'in'运算符的逻辑,使得代码更具模块化和可重用性。这种方法适用于需要在多个地方判断字符存在的场景。
总结:
在Python中有多种方法判断字符串是否包含某个字符,包括in
运算符、find()
方法、index()
方法、正则表达式、count()
方法、any()
函数与生成器表达式、列表解析、集合操作、filter()
函数和自定义函数。每种方法都有其适用场景和优势,选择适合自己需求的方法,可以使代码更加简洁、可读和高效。
相关问答FAQs:
如何在Python中检查字符串是否包含特定字符?
在Python中,可以使用in
关键字来判断一个字符串是否包含某个特定的字符。例如,if 'a' in 'banana':
将返回True,因为字符串"banana"中包含字符"a"。此外,str.find()
和str.index()
方法也可以用来查找字符的位置,如果字符存在,它们会返回字符的索引,否则返回-1或引发异常。
是否可以在Python中忽略大小写来判断字符的包含?
当然可以。如果希望在判断字符时忽略大小写,可以将字符串转换为统一的大小写形式,比如使用str.lower()
或str.upper()
。例如,if 'a' in 'Banana'.lower():
将返回True,因为在转换后,"banana"中的"a"与"A"相同。
有没有内置函数可以更简洁地判断字符是否存在于字符串中?
Python提供了一些非常方便的字符串方法来简化字符判断。例如,使用str.count()
方法可以返回指定字符在字符串中出现的次数,如果返回值大于0,则表示该字符存在于字符串中。这种方法也可以有效地判断字符的重复情况。
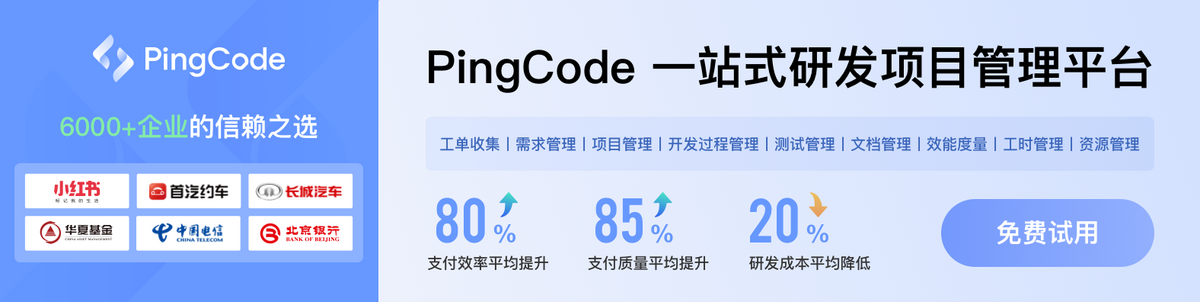