在Python接口自动化测试中,做断言的核心方法是使用测试框架如unittest或pytest,结合requests库对接口进行请求,并对响应数据进行验证。常用的断言方式包括状态码验证、响应时间验证、响应内容验证等。下面将详细介绍如何使用这些方法进行接口自动化断言。
一、状态码验证
状态码是HTTP响应的基本组成部分,通过状态码可以判断请求是否成功。通常我们会验证状态码是否为200,表示请求成功。
import requests
import unittest
class TestAPI(unittest.TestCase):
def test_status_code(self):
url = 'http://example.com/api'
response = requests.get(url)
self.assertEqual(response.status_code, 200, "Status code is not 200")
if __name__ == '__main__':
unittest.main()
二、响应时间验证
响应时间是衡量接口性能的重要指标,通常会设置一个期望的最大响应时间来验证接口的性能。
class TestAPITime(unittest.TestCase):
def test_response_time(self):
url = 'http://example.com/api'
response = requests.get(url)
self.assertLess(response.elapsed.total_seconds(), 2, "Response time is too long")
if __name__ == '__main__':
unittest.main()
三、响应内容验证
验证响应内容是接口测试的核心,通过验证响应内容是否符合预期来确定接口是否正确实现。
1、验证响应字段的存在
class TestAPIContent(unittest.TestCase):
def test_response_content(self):
url = 'http://example.com/api'
response = requests.get(url)
data = response.json()
self.assertIn('key', data, "Key is not in response")
if __name__ == '__main__':
unittest.main()
2、验证响应字段的值
class TestAPIContentValue(unittest.TestCase):
def test_response_content_value(self):
url = 'http://example.com/api'
response = requests.get(url)
data = response.json()
self.assertEqual(data.get('key'), 'expected_value', "Value is not as expected")
if __name__ == '__main__':
unittest.main()
四、组合断言
在实际测试中,通常会组合使用多种断言方法来全面验证接口。下面是一个组合断言的示例:
class TestAPI(unittest.TestCase):
def test_api(self):
url = 'http://example.com/api'
response = requests.get(url)
# 状态码验证
self.assertEqual(response.status_code, 200, "Status code is not 200")
# 响应时间验证
self.assertLess(response.elapsed.total_seconds(), 2, "Response time is too long")
# 响应内容验证
data = response.json()
self.assertIn('key', data, "Key is not in response")
self.assertEqual(data.get('key'), 'expected_value', "Value is not as expected")
if __name__ == '__main__':
unittest.main()
五、异常处理
在接口测试中,可能会遇到各种异常情况,如请求超时、服务器错误等。为了提高测试的鲁棒性,可以使用异常处理机制。
class TestAPIException(unittest.TestCase):
def test_api_exception(self):
url = 'http://example.com/api'
try:
response = requests.get(url, timeout=5)
response.raise_for_status() # 如果响应码不是200,主动抛出异常
except requests.exceptions.Timeout:
self.fail("Request timed out")
except requests.exceptions.HTTPError as e:
self.fail(f"HTTP error occurred: {e}")
except requests.exceptions.RequestException as e:
self.fail(f"Request exception occurred: {e}")
if __name__ == '__main__':
unittest.main()
六、pytest框架的使用
除了unittest,pytest也是一个非常流行的测试框架,使用pytest进行接口测试断言会更加简洁。
import requests
def test_status_code():
url = 'http://example.com/api'
response = requests.get(url)
assert response.status_code == 200, "Status code is not 200"
def test_response_time():
url = 'http://example.com/api'
response = requests.get(url)
assert response.elapsed.total_seconds() < 2, "Response time is too long"
def test_response_content():
url = 'http://example.com/api'
response = requests.get(url)
data = response.json()
assert 'key' in data, "Key is not in response"
assert data.get('key') == 'expected_value', "Value is not as expected"
七、数据驱动测试
在实际测试中,接口测试通常需要测试多组数据,可以使用数据驱动测试来提高测试效率。pytest提供了pytest.mark.parametrize
装饰器来实现数据驱动测试。
import pytest
import requests
@pytest.mark.parametrize("url, status_code", [
('http://example.com/api1', 200),
('http://example.com/api2', 200),
('http://example.com/api3', 404),
])
def test_status_code(url, status_code):
response = requests.get(url)
assert response.status_code == status_code, f"Status code for {url} is not {status_code}"
八、结果报告
测试结果报告是测试的重要组成部分,通过报告可以清晰地展示测试结果。pytest可以与插件pytest-html结合生成HTML格式的测试报告。
pip install pytest-html
pytest --html=report.html
九、总结
Python接口自动化测试断言是确保接口功能正确性的重要手段,通过状态码验证、响应时间验证、响应内容验证等多种方式,可以全面验证接口的各项指标。在实际测试中,组合使用多种断言方法,并结合异常处理和数据驱动测试,可以提高测试的覆盖率和效率。同时,生成测试报告可以帮助更好地展示测试结果。希望这篇文章能对您在进行Python接口自动化测试时有所帮助。
相关问答FAQs:
如何在Python接口自动化中进行断言?
在Python接口自动化测试中,断言用于验证接口返回的数据是否符合预期。通常,可以使用unittest
、pytest
等测试框架中的断言功能。具体做法包括:在发送请求后,使用相应的断言方法(如assertEqual
, assertIn
等)检查返回的状态码、响应体内容或其他特定字段。例如,可以通过以下代码进行简单的断言检查:
import requests
import unittest
class TestAPI(unittest.TestCase):
def test_api_response(self):
response = requests.get('http://example.com/api')
self.assertEqual(response.status_code, 200)
self.assertIn('expected_key', response.json())
断言错误时如何进行调试?
当断言失败时,调试是非常重要的。可以通过打印响应内容、状态码以及请求参数来帮助找出问题所在。例如,使用print(response.text)
来查看实际返回的数据,结合日志信息,可以更好地定位问题。此外,使用调试工具(如PyCharm的调试功能)也能有效帮助分析代码执行情况。
在接口自动化中如何选择合适的断言方式?
选择合适的断言方式取决于接口的功能需求和测试目标。针对简单的状态码检查,可以使用基本的比较断言;而对于复杂的响应体验证,可能需要结合正则表达式或JSON Schema进行深层次的验证。确保断言的覆盖面广泛且具有针对性,可以提高测试的有效性和可靠性。
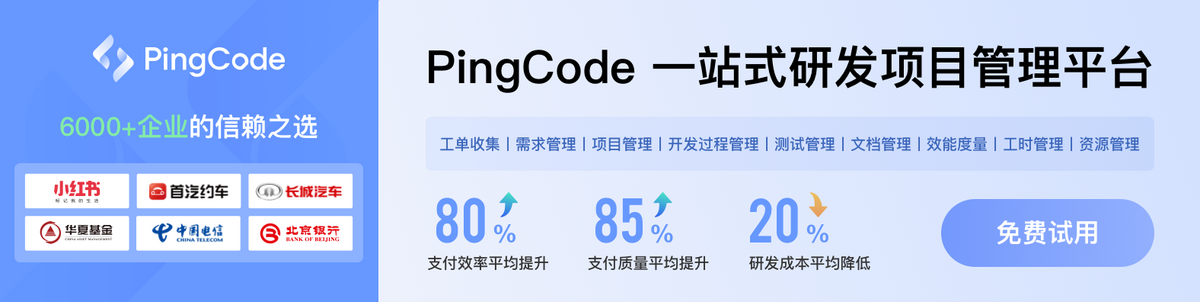