要下载FTP服务器上的所有文件夹,您可以使用Python的ftplib库、os库和shutil库。使用这些库,您可以连接到FTP服务器、遍历目录结构并下载文件。具体步骤包括:连接FTP服务器、遍历目录结构、创建本地目录、下载文件。
连接FTP服务器是下载文件的第一步,它允许您访问和遍历远程目录结构。
一、连接FTP服务器
连接到FTP服务器是下载文件的第一步,下面是一个简单的示例代码:
from ftplib import FTP
ftp = FTP('ftp.example.com') # 连接FTP服务器
ftp.login(user='username', passwd='password') # 登录
这段代码展示了如何连接FTP服务器并登录,接下来我们需要遍历目录结构。
二、遍历目录结构
遍历目录结构可以使用FTP的nlst()方法,该方法列出当前目录下的所有文件和文件夹:
def list_files(ftp, dir):
file_list = []
ftp.retrlines('NLST ' + dir, file_list.append)
return file_list
此函数返回指定目录下的所有文件和文件夹的列表。我们可以递归调用该函数来遍历整个目录结构。
三、创建本地目录
下载文件之前,我们需要在本地创建相应的目录结构。可以使用os.makedirs()方法:
import os
def create_local_dir(path):
if not os.path.exists(path):
os.makedirs(path)
四、下载文件
最后一步是下载文件,可以使用FTP的retrbinary()方法:
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as f:
ftp.retrbinary('RETR ' + remote_file, f.write)
整合代码
将以上各个部分整合在一起,形成一个完整的程序:
from ftplib import FTP
import os
def list_files(ftp, dir):
file_list = []
ftp.retrlines('NLST ' + dir, file_list.append)
return file_list
def create_local_dir(path):
if not os.path.exists(path):
os.makedirs(path)
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as f:
ftp.retrbinary('RETR ' + remote_file, f.write)
def download_ftp_tree(ftp, remote_dir, local_dir):
create_local_dir(local_dir)
file_list = list_files(ftp, remote_dir)
for file in file_list:
local_path = os.path.join(local_dir, os.path.basename(file))
remote_path = remote_dir + '/' + file
if '.' not in os.path.basename(file): # 这是一个目录
download_ftp_tree(ftp, remote_path, local_path)
else: # 这是一个文件
download_file(ftp, remote_path, local_path)
使用示例
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
download_ftp_tree(ftp, '/remote/dir', 'local/dir')
通过这种方式,您可以下载FTP服务器上的所有文件和文件夹,包括嵌套的目录结构。
五、处理异常
在实际应用中,处理异常和错误是非常重要的。可以使用try-except块来处理可能发生的异常:
def download_ftp_tree(ftp, remote_dir, local_dir):
create_local_dir(local_dir)
try:
file_list = list_files(ftp, remote_dir)
except Exception as e:
print(f'Error listing files in {remote_dir}: {e}')
return
for file in file_list:
local_path = os.path.join(local_dir, os.path.basename(file))
remote_path = remote_dir + '/' + file
try:
if '.' not in os.path.basename(file): # 这是一个目录
download_ftp_tree(ftp, remote_path, local_path)
else: # 这是一个文件
download_file(ftp, remote_path, local_path)
except Exception as e:
print(f'Error downloading {remote_path}: {e}')
通过这种方式,您可以确保即使在下载过程中发生错误,程序也能继续运行并记录错误。
六、总结
通过使用Python的ftplib库、os库和shutil库,您可以轻松地连接到FTP服务器、遍历目录结构并下载文件。处理异常和错误对于确保程序的稳定性和可靠性非常重要。在实际应用中,您可以根据需要进一步优化和扩展这些代码。
总的来说,下载FTP服务器上的所有文件夹涉及连接FTP服务器、遍历目录结构、创建本地目录和下载文件等步骤。通过整合这些步骤,您可以实现一个完整的下载程序。处理异常和错误可以确保程序的稳定性和可靠性。希望这篇文章对您有所帮助。
相关问答FAQs:
如何使用Python连接到FTP服务器?
要连接到FTP服务器,可以使用Python的ftplib
库。首先,导入该库,然后创建一个FTP对象并使用connect
方法连接到服务器。接着,使用login
方法输入用户名和密码进行身份验证。成功连接后,就可以开始下载文件和文件夹。
在Python中如何递归下载FTP服务器上的所有文件夹及其内容?
可以通过编写一个递归函数来下载FTP服务器上的所有文件夹及其内容。该函数应该列出当前目录中的所有文件和子目录。如果遇到子目录,函数将调用自身以进入子目录并继续下载,确保在下载文件时使用retrbinary
方法。
有哪些库可以帮助简化FTP文件下载过程?
除了ftplib
,还有一些第三方库可以简化FTP操作。例如,pyftpdlib
是一个功能强大的库,可以用于创建和管理FTP服务器;而wget
库则能简化文件下载过程。选择适合您需求的库,可以提高代码的可读性和效率。
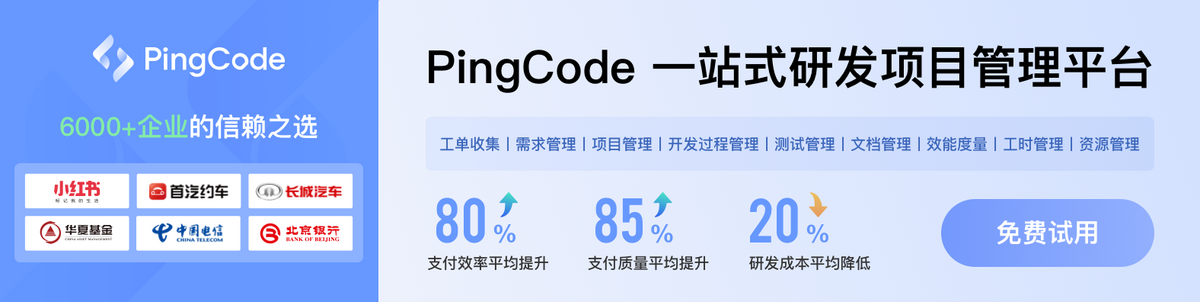