在Python中取出多线程的数据库的方法包括使用线程安全的数据库连接库、适当的数据库连接池、合理的锁机制。使用线程安全的数据库连接库,如SQLite3、PyMySQL,可以确保线程间操作不冲突,适当的数据库连接池,如使用SQLAlchemy的连接池管理,能够提高多线程环境下的数据库访问效率,合理的锁机制,如使用线程锁,可以避免多个线程同时访问数据库时引发的数据不一致问题。
多线程数据库操作在许多高性能和高并发应用中是非常重要的。下面我们将详细介绍如何在Python中实现多线程数据库操作的最佳实践。
一、线程安全的数据库连接库
在多线程环境下,使用线程安全的数据库连接库是至关重要的。Python中常用的线程安全数据库连接库有SQLite3和PyMySQL。它们可以确保在多个线程中进行数据库操作时不会发生冲突。
1. SQLite3
SQLite3是Python内置的数据库库,它是线程安全的,但默认模式下不同线程共享一个数据库连接是不安全的。因此,建议为每个线程创建单独的数据库连接。
import sqlite3
import threading
def thread_job():
conn = sqlite3.connect('example.db', check_same_thread=False)
cursor = conn.cursor()
cursor.execute('SELECT * FROM table_name')
result = cursor.fetchall()
print(result)
conn.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2. PyMySQL
对于MySQL数据库,PyMySQL库是一个很好的选择,它是线程安全的,每个线程都可以创建自己的数据库连接。
import pymysql
import threading
def thread_job():
conn = pymysql.connect(host='localhost', user='user', password='passwd', db='dbname')
cursor = conn.cursor()
cursor.execute('SELECT * FROM table_name')
result = cursor.fetchall()
print(result)
conn.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
二、适当的数据库连接池
数据库连接池是一个非常有效的工具,它可以显著提高多线程环境下的数据库访问效率。SQLAlchemy提供了一个强大的连接池管理功能,适用于多种数据库。
1. 使用SQLAlchemy连接池
SQLAlchemy是一个高级的ORM库,支持多种数据库后端,并且内置了高效的连接池管理。
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
import threading
engine = create_engine('mysql+pymysql://user:passwd@localhost/dbname', pool_size=10, max_overflow=20)
Session = sessionmaker(bind=engine)
def thread_job():
session = Session()
result = session.execute('SELECT * FROM table_name').fetchall()
print(result)
session.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
三、合理的锁机制
在多线程环境下,使用锁机制可以确保多个线程不会同时操作同一个数据库资源,从而避免数据不一致的问题。
1. 使用线程锁
Python的threading
模块提供了锁对象,可以用来确保同一时间只有一个线程访问数据库。
import sqlite3
import threading
lock = threading.Lock()
def thread_job():
with lock:
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM table_name')
result = cursor.fetchall()
print(result)
conn.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2. 使用上下文管理器
上下文管理器可以确保在离开with块时自动释放锁,这样可以避免忘记释放锁而导致的死锁问题。
import pymysql
import threading
lock = threading.Lock()
def thread_job():
with lock:
conn = pymysql.connect(host='localhost', user='user', password='passwd', db='dbname')
cursor = conn.cursor()
cursor.execute('SELECT * FROM table_name')
result = cursor.fetchall()
print(result)
conn.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
四、结合使用多种方法
在实际应用中,我们可以结合使用上述方法,以获得更好的性能和数据一致性。下面是一个综合示例,结合使用了SQLAlchemy连接池和线程锁。
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
import threading
engine = create_engine('mysql+pymysql://user:passwd@localhost/dbname', pool_size=10, max_overflow=20)
Session = sessionmaker(bind=engine)
lock = threading.Lock()
def thread_job():
with lock:
session = Session()
result = session.execute('SELECT * FROM table_name').fetchall()
print(result)
session.close()
threads = []
for _ in range(5):
thread = threading.Thread(target=thread_job)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
五、注意事项
- 避免共享数据库连接:每个线程应当使用独立的数据库连接,避免多个线程共享同一个连接。
- 合理设置连接池大小:根据实际应用的并发量,合理设置连接池的大小,以避免过多的连接导致资源浪费或连接不足。
- 使用事务:在多线程环境下,使用事务可以确保数据操作的原子性和一致性。
- 处理异常:在多线程数据库操作中,需注意捕获并处理各种可能的异常,如连接超时、SQL错误等。
六、性能优化
在多线程数据库操作中,性能优化也是一个重要的方面。以下是一些常用的优化技巧:
1. 批量操作
将多个数据库操作合并为一个批量操作,可以减少数据库连接的次数,提高效率。
import pymysql
def batch_insert(data):
conn = pymysql.connect(host='localhost', user='user', password='passwd', db='dbname')
cursor = conn.cursor()
cursor.executemany('INSERT INTO table_name (column1, column2) VALUES (%s, %s)', data)
conn.commit()
conn.close()
2. 使用索引
为常用的查询字段创建索引,可以显著提高查询性能。
CREATE INDEX index_name ON table_name (column_name);
3. 读写分离
对于读写分离的数据库架构,可以将读操作分配到从库,写操作分配到主库,以提高性能和可用性。
七、总结
在Python中取出多线程的数据库涉及到多个方面,包括选择合适的线程安全数据库连接库、使用适当的数据库连接池、合理的锁机制以及性能优化。通过结合使用这些方法,可以有效地提高多线程环境下的数据库访问效率和数据一致性。希望本文的内容对您在实际应用中有所帮助。
相关问答FAQs:
如何在Python中实现多线程数据库操作?
在Python中,可以使用threading
模块结合数据库连接库(如sqlite3
、psycopg2
或SQLAlchemy
等)来实现多线程数据库操作。首先,确保为每个线程创建一个独立的数据库连接,以避免连接冲突。可以使用线程池管理线程,提高效率。同时,要注意线程安全性问题,确保操作的原子性。
多线程访问数据库时需要注意哪些问题?
在多线程环境中,数据库连接的共享可能导致数据一致性问题。确保每个线程使用独立的连接,并考虑使用锁机制来保护对共享资源的访问。此外,确保数据库驱动支持多线程操作,避免潜在的连接问题。
如何优化多线程数据库查询性能?
优化多线程数据库查询性能可以通过多个方面实现。首先,合理设计数据库索引,减少查询时间。其次,使用连接池管理数据库连接,避免频繁创建和关闭连接带来的开销。此外,可以考虑将一些长时间运行的查询任务分配给不同的线程,并在应用层面进行结果的合并与处理,以提高整体性能。
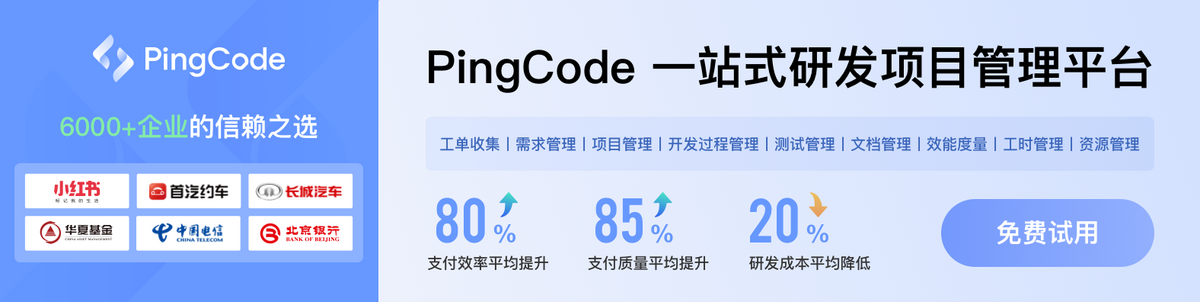