在Python中,可以使用多种方法将字典内容逐个写入txt文件,包括使用文件操作函数以及格式化输出等。主要方法有:遍历字典、使用with open
语句、格式化字符串、处理嵌套字典。
下面我们将详细描述其中一种方法。
一、遍历字典并写入txt文件
1、使用 with open
语句
Python中with open
语句用于打开文件,并确保在操作完成后关闭文件。这是一个常用的文件操作方法,安全且简洁。以下是如何使用这种方法将字典内容逐个写入txt文件的示例:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
with open('output.txt', 'w') as file:
for key, value in my_dict.items():
file.write(f'{key}: {value}\n')
在这个示例中,with open('output.txt', 'w') as file
打开一个名为output.txt
的文件,并确保在操作完成后关闭文件。for key, value in my_dict.items():
循环遍历字典中的每一个键值对,并使用file.write(f'{key}: {value}\n')
将它们逐个写入文件,每个键值对占一行。
2、处理嵌套字典
当字典包含嵌套结构时,需要递归地遍历嵌套字典,并将每一层的内容写入txt文件。以下是处理嵌套字典的示例:
def write_dict_to_file(d, file, indent=0):
for key, value in d.items():
file.write(' ' * indent + f'{key}: ')
if isinstance(value, dict):
file.write('\n')
write_dict_to_file(value, file, indent + 4)
else:
file.write(f'{value}\n')
nested_dict = {
'key1': 'value1',
'key2': {
'subkey1': 'subvalue1',
'subkey2': 'subvalue2'
},
'key3': 'value3'
}
with open('nested_output.txt', 'w') as file:
write_dict_to_file(nested_dict, file)
在这个示例中,我们定义了一个递归函数write_dict_to_file
,用于处理嵌套字典。这个函数在遇到嵌套字典时,会增加缩进并递归调用自身,将嵌套字典的内容逐个写入文件。
二、格式化输出
1、使用字符串格式化
字符串格式化是一种将变量插入字符串中的方法。在Python中,可以使用f字符串、str.format()
方法或百分号格式化。以下是使用f字符串将字典内容写入txt文件的示例:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
with open('formatted_output.txt', 'w') as file:
for key, value in my_dict.items():
file.write(f'{key}: {value}\n')
这种方法简洁且易于阅读。与之前的示例类似,with open('formatted_output.txt', 'w') as file
打开一个名为formatted_output.txt
的文件,并确保在操作完成后关闭文件。for key, value in my_dict.items():
循环遍历字典中的每一个键值对,并使用file.write(f'{key}: {value}\n')
将它们逐个写入文件,每个键值对占一行。
2、使用 str.format()
方法
str.format()
方法是另一种常用的字符串格式化方法。以下是使用 str.format()
将字典内容写入txt文件的示例:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
with open('formatted_output.txt', 'w') as file:
for key, value in my_dict.items():
file.write('{}: {}\n'.format(key, value))
这种方法与使用f字符串的方法类似,但使用'{}: {}'.format(key, value)
进行字符串格式化。
3、使用百分号格式化
百分号格式化是另一种字符串格式化方法。以下是使用百分号格式化将字典内容写入txt文件的示例:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
with open('formatted_output.txt', 'w') as file:
for key, value in my_dict.items():
file.write('%s: %s\n' % (key, value))
这种方法使用'%s: %s\n' % (key, value)
进行字符串格式化。
三、处理特殊情况
1、处理字典中的列表
当字典中的值是列表时,需要遍历列表并将每个元素写入txt文件。以下是处理字典中包含列表的示例:
my_dict = {'key1': 'value1', 'key2': ['list_item1', 'list_item2'], 'key3': 'value3'}
with open('list_output.txt', 'w') as file:
for key, value in my_dict.items():
if isinstance(value, list):
file.write(f'{key}:\n')
for item in value:
file.write(f' - {item}\n')
else:
file.write(f'{key}: {value}\n')
在这个示例中,我们在循环中检查字典值的类型。如果值是列表,我们先写入键,然后遍历列表并将每个元素写入文件,并增加缩进以区分列表项。
2、处理字典中的复杂数据类型
当字典中的值是复杂数据类型(如元组、集合等)时,需要根据具体情况处理。以下是处理字典中包含元组和集合的示例:
my_dict = {'key1': 'value1', 'key2': ('tuple_item1', 'tuple_item2'), 'key3': {'set_item1', 'set_item2'}}
with open('complex_output.txt', 'w') as file:
for key, value in my_dict.items():
if isinstance(value, tuple):
file.write(f'{key} (tuple):\n')
for item in value:
file.write(f' - {item}\n')
elif isinstance(value, set):
file.write(f'{key} (set):\n')
for item in value:
file.write(f' - {item}\n')
else:
file.write(f'{key}: {value}\n')
在这个示例中,我们在循环中检查字典值的类型。如果值是元组或集合,我们分别处理它们并写入文件。
四、总结
将字典内容逐个写入txt文件是一个常见的任务,可以通过遍历字典、使用with open
语句、格式化字符串等方法实现。对于嵌套字典、包含列表或复杂数据类型的字典,需要根据具体情况进行处理。通过这些方法,可以轻松地将字典内容写入txt文件并满足各种需求。
相关问答FAQs:
如何将Python字典中的内容逐个写入txt文件?
要将字典中的内容逐个写入txt文件,可以使用Python的内置文件操作功能。首先,打开文件并使用循环遍历字典,逐行写入每个键值对。示例代码如下:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
with open('output.txt', 'w') as file:
for key, value in my_dict.items():
file.write(f'{key}: {value}\n')
这样,每个键值对都会以“键: 值”的格式写入文件中。
使用with语句写入文件有什么好处?
使用with语句可以确保在文件操作完成后自动关闭文件。这不仅简化了代码,还减少了文件未关闭可能导致的错误。即使在写入过程中发生异常,with语句也能确保文件被正确关闭,提高了程序的安全性和稳定性。
如果字典中的值是列表或其他字典,该如何处理?
当字典的值是列表或其他字典时,可以使用递归或循环来处理这些嵌套结构。对于列表,可以遍历列表中的每个元素并逐个写入;对于嵌套字典,可以调用相同的写入逻辑。以下是一个示例:
nested_dict = {'name': 'Alice', 'hobbies': ['reading', 'traveling'], 'address': {'city': 'New York', 'zip': '10001'}}
def write_dict(d, file):
for key, value in d.items():
if isinstance(value, list):
file.write(f'{key}: {", ".join(value)}\n')
elif isinstance(value, dict):
file.write(f'{key}:\n')
write_dict(value, file)
else:
file.write(f'{key}: {value}\n')
with open('output.txt', 'w') as file:
write_dict(nested_dict, file)
这种方式能够确保嵌套的数据结构被正确处理并写入文件。
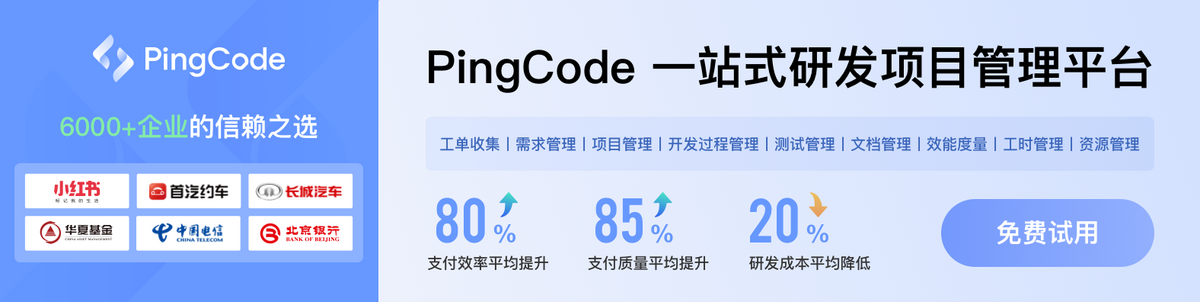