开头段落:
要查找一个文件的编码,你可以使用chardet库、使用codecs模块、使用Pandas库、手动检查文件头信息。其中,使用chardet库是最常用且方便的方法。chardet库是一个字符编码检测库,可以检测大多数文件的编码格式。你只需安装chardet库,然后读取文件内容,使用chardet的detect函数即可得到文件编码。下面详细介绍各种方法。
正文:
一、使用CHARDET库
使用chardet库是检测文件编码的简单方法。它可以识别多种编码格式,非常适合处理未知编码的文件。下面是使用chardet库检测文件编码的详细步骤:
安装chardet库
首先,你需要安装chardet库。你可以通过以下命令来安装:
pip install chardet
读取文件并检测编码
安装完成后,你可以使用以下代码读取文件并检测其编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
file_path = 'your_file.txt'
print(detect_encoding(file_path))
上面的代码中,首先以二进制模式读取文件内容,然后使用chardet.detect函数检测文件编码并返回结果。
二、使用CODECS模块
Python的codecs模块也可以用来检测文件编码。虽然它不像chardet库那样强大,但对于某些简单的场景足够用了。下面是使用codecs模块的详细步骤:
读取文件并检测编码
import codecs
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
try:
raw_data.decode('utf-8')
encoding = 'utf-8'
except UnicodeDecodeError:
try:
raw_data.decode('utf-16')
encoding = 'utf-16'
except UnicodeDecodeError:
encoding = 'unknown'
return encoding
file_path = 'your_file.txt'
print(detect_encoding(file_path))
上面的代码中,首先以二进制模式读取文件内容,然后尝试将其解码为utf-8和utf-16。如果解码失败,则返回未知编码。
三、使用PANDAS库
Pandas库是数据分析的利器,它的read_csv函数可以自动检测文件的编码。下面是使用Pandas库检测文件编码的详细步骤:
安装Pandas库
首先,你需要安装Pandas库。你可以通过以下命令来安装:
pip install pandas
读取文件并检测编码
安装完成后,你可以使用以下代码读取文件并检测其编码:
import pandas as pd
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = pd.read_csv(file_path, encoding='utf-8', error_bad_lines=False)
encoding = result.encoding
return encoding
file_path = 'your_file.txt'
print(detect_encoding(file_path))
上面的代码中,首先以二进制模式读取文件内容,然后使用Pandas库的read_csv函数读取文件并检测编码。
四、手动检查文件头信息
在某些情况下,你可以通过手动检查文件头信息来检测文件编码。某些文件格式(如XML和HTML)包含编码声明,可以直接读取这些声明来确定文件编码。下面是手动检查文件头信息的详细步骤:
读取文件并检测编码
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(100)
if raw_data.startswith(b'\xff\xfe'):
encoding = 'utf-16'
elif raw_data.startswith(b'\xfe\xff'):
encoding = 'utf-16'
elif raw_data.startswith(b'\xef\xbb\xbf'):
encoding = 'utf-8-sig'
else:
encoding = 'unknown'
return encoding
file_path = 'your_file.txt'
print(detect_encoding(file_path))
上面的代码中,首先以二进制模式读取文件的前100个字节,然后检查文件头信息以确定文件编码。
五、结合多种方法
在某些情况下,单一的方法可能不足以准确检测文件编码。你可以结合多种方法来提高检测的准确性。下面是结合多种方法的详细步骤:
读取文件并检测编码
import chardet
import pandas as pd
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
try:
result = pd.read_csv(file_path, encoding='utf-8', error_bad_lines=False)
encoding = result.encoding
except UnicodeDecodeError:
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
file_path = 'your_file.txt'
print(detect_encoding(file_path))
上面的代码中,首先尝试使用Pandas库检测文件编码,如果失败则使用chardet库检测。
六、总结
通过本文的介绍,我们了解了几种检测文件编码的方法,包括使用chardet库、codecs模块、Pandas库、手动检查文件头信息以及结合多种方法。每种方法都有其优缺点,具体选择哪种方法取决于你的需求和实际情况。希望这些方法能够帮助你准确检测文件的编码。
相关问答FAQs:
如何在Python中自动检测文件编码?
可以使用chardet
库来自动检测文件编码。首先,安装该库:pip install chardet
。然后,使用以下代码来读取文件并检测编码:
import chardet
with open('your_file.txt', 'rb') as f:
result = chardet.detect(f.read())
print(result['encoding'])
此方法会返回文件的编码格式,帮助您确认文件的字符集。
如果我不知道文件的路径,如何在Python中查找它?
可以使用os
库配合glob
模块来查找特定文件。例如,如果您想查找当前目录下的所有文本文件,可以使用以下代码:
import os
import glob
files = glob.glob(os.path.join(os.getcwd(), '*.txt'))
print(files)
这将返回当前工作目录中所有以.txt
结尾的文件路径,方便您进一步操作。
在处理不同编码文件时,Python会遇到什么问题?
如果您尝试以错误的编码打开文件,可能会遇到UnicodeDecodeError
。这种错误通常发生在读取文件时使用的编码与文件实际编码不匹配。为避免这种情况,建议在读取文件前先检测编码,确保您使用的是正确的编码格式。此外,可以考虑使用errors='ignore'
选项来忽略无法解码的字符,尽管这可能导致数据丢失。
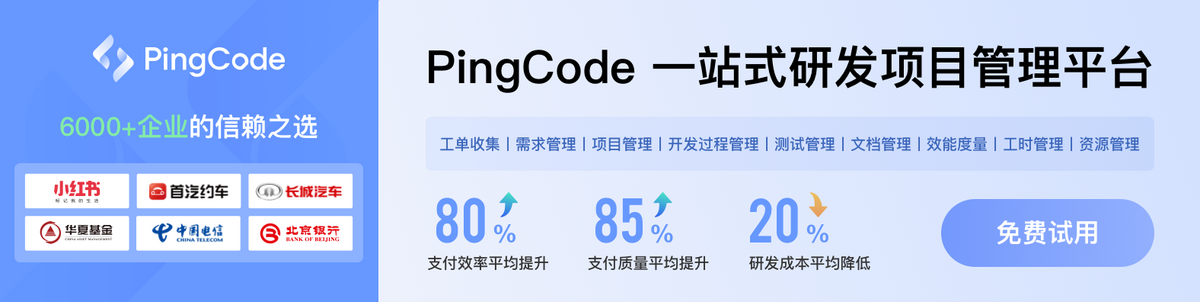