要统计Python列表中的指定字典,可以使用内置函数和循环、条件判断、计数器等工具。其中,使用 collections.Counter
类进行统计是一个常见且高效的方法。下面将详细介绍如何在Python中统计列表中的指定字典。
一、导入相关模块
在开始之前,导入必要的模块,比如 collections
模块。该模块提供了许多有用的集合类,可以帮助简化统计操作。
from collections import Counter
二、定义列表和字典
首先,定义一个包含多个字典的列表。每个字典可以包含不同的键和值。下面是一个示例列表:
data = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 30},
{"name": "Alice", "age": 25},
{"name": "Charlie", "age": 35},
{"name": "Alice", "age": 25},
]
三、使用计数器统计字典
使用 collections.Counter
类来统计列表中出现的字典。
counter = Counter(tuple(d.items()) for d in data)
在这段代码中,tuple(d.items())
将每个字典转换为元组,这样它们可以被计数器识别和统计。
四、显示统计结果
接下来,输出统计结果。可以使用一个简单的循环来显示每个字典及其出现的次数。
for item, count in counter.items():
print(dict(item), count)
五、按指定条件统计
有时,我们可能只想统计满足特定条件的字典。例如,统计名称为 "Alice" 的字典。
alice_count = sum(1 for d in data if d.get("name") == "Alice")
print(f"Number of dictionaries with name 'Alice': {alice_count}")
六、使用函数封装
为了提高代码的可读性和复用性,可以将统计操作封装到函数中。
def count_dicts(data, key, value):
return sum(1 for d in data if d.get(key) == value)
alice_count = count_dicts(data, "name", "Alice")
print(f"Number of dictionaries with name 'Alice': {alice_count}")
七、统计字典的其他方法
除了使用 collections.Counter
,还可以使用其他方法来统计字典。例如,使用循环和条件判断。
def count_dicts_loop(data, key, value):
count = 0
for d in data:
if d.get(key) == value:
count += 1
return count
alice_count = count_dicts_loop(data, "name", "Alice")
print(f"Number of dictionaries with name 'Alice': {alice_count}")
八、基于多个键值对统计
有时,我们可能需要基于多个键值对进行统计。例如,统计名称为 "Alice" 且年龄为 25 的字典。
def count_dicts_multi(data, conditions):
return sum(1 for d in data if all(d.get(k) == v for k, v in conditions.items()))
conditions = {"name": "Alice", "age": 25}
alice_count = count_dicts_multi(data, conditions)
print(f"Number of dictionaries with name 'Alice' and age 25: {alice_count}")
九、使用 pandas 进行统计
如果数据量较大或需要进行复杂的统计,可以考虑使用 pandas
库。pandas
提供了强大的数据处理和统计功能。
import pandas as pd
df = pd.DataFrame(data)
alice_count = df[(df['name'] == 'Alice') & (df['age'] == 25)].shape[0]
print(f"Number of dictionaries with name 'Alice' and age 25 using pandas: {alice_count}")
十、总结
通过以上方法,我们可以在Python中高效地统计列表中的指定字典。无论是使用内置函数、循环和条件判断,还是使用第三方库 pandas
,都可以灵活地处理各种统计需求。选择合适的方法可以提高代码的可读性和效率。
相关问答FAQs:
如何在Python中统计列表中指定字典的数量?
要统计列表中指定字典的数量,可以使用count()
方法或列表推导式结合sum()
函数。使用count()
方法可以快速获取特定字典的出现次数,而列表推导式则可以实现更复杂的条件统计。例如,假设有一个列表包含多个字典,可以通过以下方法实现统计:
my_list = [{'name': 'Alice'}, {'name': 'Bob'}, {'name': 'Alice'}]
target_dict = {'name': 'Alice'}
count = my_list.count(target_dict)
print(count) # 输出 2
如何在Python中根据条件统计字典的属性值?
如果需要根据字典的特定属性进行统计,可以使用列表推导式或filter()
函数。例如,要统计列表中所有字典中某个属性的值,可以这样做:
my_list = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Alice', 'age': 22}]
age_count = sum(1 for d in my_list if d['name'] == 'Alice')
print(age_count) # 输出 2
如何在Python中统计列表中多个字典的不同属性?
如果需要统计多个字典的不同属性,可以使用collections.Counter
模块,它可以帮助统计每个属性的出现次数。例如,统计不同名字的出现次数可以这样实现:
from collections import Counter
my_list = [{'name': 'Alice'}, {'name': 'Bob'}, {'name': 'Alice'}, {'name': 'Charlie'}]
name_counts = Counter(d['name'] for d in my_list)
print(name_counts) # 输出 Counter({'Alice': 2, 'Bob': 1, 'Charlie': 1})
使用Counter可以快速得到每个名字的出现次数,方便进行后续分析。
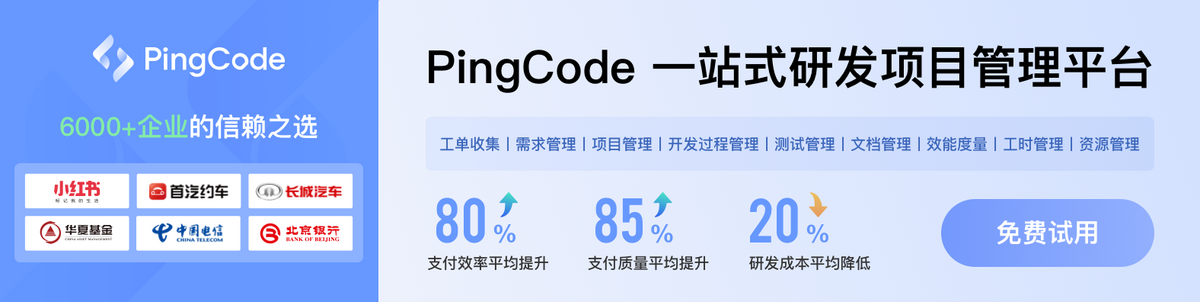