在Python中编写万能验证码是一项具有挑战性的任务,涉及生成随机字符串、图像处理和验证码识别等多个方面。使用Python生成和破解验证码、实现验证码的图像处理、使用OCR技术识别验证码,是实现万能验证码的关键步骤。下面我们将详细介绍如何使用Python编写万能验证码程序。
一、生成随机验证码
生成随机验证码是编写万能验证码的第一步。我们可以使用Python的内置库random
来生成随机字符串,然后使用图像库PIL
来创建验证码图像。
import random
import string
from PIL import Image, ImageDraw, ImageFont
def generate_random_string(length=6):
letters = string.ascii_letters + string.digits
return ''.join(random.choice(letters) for _ in range(length))
def create_captcha_image(text, font_path='arial.ttf', font_size=36):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.truetype(font_path, font_size)
text_width, text_height = draw.textsize(text, font=font)
text_x = (width - text_width) // 2
text_y = (height - text_height) // 2
draw.text((text_x, text_y), text, font=font, fill=(0, 0, 0))
return image
random_string = generate_random_string()
captcha_image = create_captcha_image(random_string)
captcha_image.show()
二、图像处理
为了提高验证码的复杂性,我们可以对图像进行一些处理,比如添加干扰线、点等。
def add_noise(draw, width, height):
for _ in range(random.randint(100, 200)):
x1 = random.randint(0, width)
y1 = random.randint(0, height)
x2 = random.randint(0, width)
y2 = random.randint(0, height)
draw.line(((x1, y1), (x2, y2)), fill=(0, 0, 0), width=1)
def add_noise_dots(draw, width, height):
for _ in range(random.randint(100, 200)):
x = random.randint(0, width)
y = random.randint(0, height)
draw.point((x, y), fill=(0, 0, 0))
def create_noisy_captcha_image(text, font_path='arial.ttf', font_size=36):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.truetype(font_path, font_size)
text_width, text_height = draw.textsize(text, font=font)
text_x = (width - text_width) // 2
text_y = (height - text_height) // 2
draw.text((text_x, text_y), text, font=font, fill=(0, 0, 0))
add_noise(draw, width, height)
add_noise_dots(draw, width, height)
return image
noisy_captcha_image = create_noisy_captcha_image(random_string)
noisy_captcha_image.show()
三、使用OCR技术识别验证码
识别验证码是编写万能验证码的最后一步。我们可以使用pytesseract
库(基于Tesseract OCR引擎)来识别验证码。
首先,确保你已经安装了Tesseract OCR引擎,并且在系统路径中可以访问。
sudo apt-get install tesseract-ocr
pip install pytesseract
pip install pillow
然后,我们可以使用以下代码识别验证码:
import pytesseract
def recognize_captcha(image):
return pytesseract.image_to_string(image)
recognized_text = recognize_captcha(noisy_captcha_image)
print(f"Recognized Captcha: {recognized_text}")
四、综合实现万能验证码
将以上所有步骤整合起来,实现一个完整的万能验证码生成和识别程序。
import random
import string
from PIL import Image, ImageDraw, ImageFont
import pytesseract
def generate_random_string(length=6):
letters = string.ascii_letters + string.digits
return ''.join(random.choice(letters) for _ in range(length))
def add_noise(draw, width, height):
for _ in range(random.randint(100, 200)):
x1 = random.randint(0, width)
y1 = random.randint(0, height)
x2 = random.randint(0, width)
y2 = random.randint(0, height)
draw.line(((x1, y1), (x2, y2)), fill=(0, 0, 0), width=1)
def add_noise_dots(draw, width, height):
for _ in range(random.randint(100, 200)):
x = random.randint(0, width)
y = random.randint(0, height)
draw.point((x, y), fill=(0, 0, 0))
def create_noisy_captcha_image(text, font_path='arial.ttf', font_size=36):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.truetype(font_path, font_size)
text_width, text_height = draw.textsize(text, font=font)
text_x = (width - text_width) // 2
text_y = (height - text_height) // 2
draw.text((text_x, text_y), text, font=font, fill=(0, 0, 0))
add_noise(draw, width, height)
add_noise_dots(draw, width, height)
return image
def recognize_captcha(image):
return pytesseract.image_to_string(image)
random_string = generate_random_string()
noisy_captcha_image = create_noisy_captcha_image(random_string)
noisy_captcha_image.show()
recognized_text = recognize_captcha(noisy_captcha_image)
print(f"Original Captcha: {random_string}")
print(f"Recognized Captcha: {recognized_text}")
五、提高验证码的复杂性和识别率
为了提高验证码的复杂性,我们可以在生成图像时加入更多干扰元素,如旋转文字、变形等。同时,我们可以通过训练自定义OCR模型来提高识别率。
旋转文字:
def create_rotated_captcha_image(text, font_path='arial.ttf', font_size=36):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.truetype(font_path, font_size)
text_width, text_height = draw.textsize(text, font=font)
text_x = (width - text_width) // 2
text_y = (height - text_height) // 2
text_image = Image.new('RGBA', (text_width, text_height))
text_draw = ImageDraw.Draw(text_image)
text_draw.text((0, 0), text, font=font, fill=(0, 0, 0))
rotated_text_image = text_image.rotate(random.randint(-30, 30), expand=1)
image.paste(rotated_text_image, (text_x, text_y), rotated_text_image)
add_noise(draw, width, height)
add_noise_dots(draw, width, height)
return image
rotated_captcha_image = create_rotated_captcha_image(random_string)
rotated_captcha_image.show()
通过上述方法,我们可以生成更加复杂的验证码,从而提高识别难度。同时,通过定期更新验证码生成算法,可以进一步增强验证码的安全性。
六、总结
编写万能验证码涉及多个步骤:生成随机验证码、图像处理、使用OCR技术识别验证码,以及提高验证码的复杂性和识别率。通过综合运用这些技术,可以实现一个功能强大的万能验证码系统。希望本文能够帮助您理解并实现Python中的万能验证码。
相关问答FAQs:
在编写万能验证码时,Python有哪些常用的库和工具?
在Python中,有几个常用的库可以帮助生成验证码,包括Pillow(用于图像处理)、random(用于生成随机数)和string(用于生成随机字符)。Pillow库尤其适合创建和处理图像,可以方便地生成文本验证码或图形验证码。
如何确保生成的验证码具有足够的安全性?
为了增强验证码的安全性,可以考虑增加复杂性,比如使用混合字符(字母、数字和符号)、增加干扰线条和噪点、使用不同的字体和颜色组合。还可以设置验证码的有效时间,确保用户在短时间内完成验证。
如何在Web应用中实现和验证生成的验证码?
在Web应用中,可以使用Flask或Django等框架来实现验证码功能。生成验证码后,可以将其存储在用户会话中,并在用户提交表单时进行验证。确保在服务器端进行比对,避免验证码被轻易猜测或篡改。
如何处理用户输入错误的验证码?
对于用户输入错误的验证码,可以提供友好的提示信息,告知用户重新输入。同时,可以考虑限制输入次数,防止恶意攻击。若连续多次输入错误,可以暂时锁定验证码功能,要求用户稍后重试,以提高安全性。
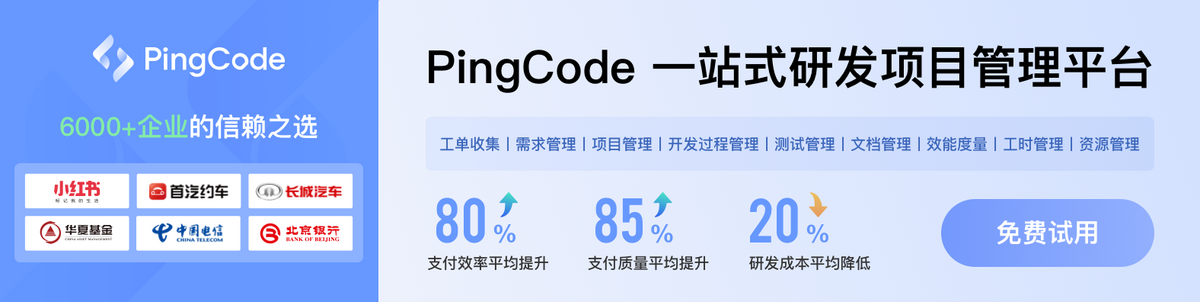