Python 替换含斜杠的字符串可以使用 str.replace()
方法、re.sub()
方法、字符串转义等方法。 其中,最常用的方法是 str.replace()
,因为它简单、直观。通过使用 str.replace()
方法,可以将字符串中的斜杠替换为任意字符或字符串。下面将对 str.replace()
方法进行详细描述。
一、str.replace()
方法
str.replace()
方法是 Python 中字符串对象的一个方法,用于将字符串中的指定子字符串替换为另一个子字符串。其基本语法如下:
str.replace(old, new, count)
old
:需要被替换的子字符串。new
:替换后的子字符串。count
:可选参数,指定最大替换次数。默认为替换所有匹配项。
示例代码:
# 示例字符串
original_string = "This/is/a/test/string"
使用 str.replace() 方法将斜杠替换为横杠
replaced_string = original_string.replace("/", "-")
print(replaced_string)
上述代码将输出:
This-is-a-test-string
二、正则表达式 re.sub()
方法
对于更复杂的替换需求,可以使用 re
模块中的 re.sub()
方法。re.sub()
方法可以使用正则表达式来匹配和替换字符串。其基本语法如下:
re.sub(pattern, repl, string, count=0, flags=0)
pattern
:匹配的正则表达式。repl
:替换后的字符串。string
:要处理的原字符串。count
:可选参数,指定最大替换次数。默认为替换所有匹配项。flags
:可选参数,指定匹配模式。
示例代码:
import re
示例字符串
original_string = "This/is/a/test/string"
使用 re.sub() 方法将斜杠替换为横杠
replaced_string = re.sub(r'/', '-', original_string)
print(replaced_string)
上述代码将输出:
This-is-a-test-string
三、字符串转义
在处理含有特殊字符(如斜杠)的字符串时,使用字符串转义可以避免误解。例如,反斜杠()在字符串中作为转义字符,需要使用双反斜杠(
\\
)表示一个反斜杠。
示例代码:
# 示例字符串
original_string = "This\\is\\a\\test\\string"
使用 str.replace() 方法将反斜杠替换为横杠
replaced_string = original_string.replace("\\", "-")
print(replaced_string)
上述代码将输出:
This-is-a-test-string
四、综合应用示例
下面是一个综合示例,演示如何使用不同的方法替换字符串中的斜杠:
import re
示例字符串
original_string = "Path/to/some/file/with\\mixed/slashes"
使用 str.replace() 方法将正斜杠替换为横杠
replaced_string_forward_slash = original_string.replace("/", "-")
print(f"Replaced forward slashes: {replaced_string_forward_slash}")
使用 str.replace() 方法将反斜杠替换为横杠
replaced_string_backward_slash = original_string.replace("\\", "-")
print(f"Replaced backward slashes: {replaced_string_backward_slash}")
使用 re.sub() 方法将正斜杠替换为横杠
replaced_string_regex_forward = re.sub(r'/', '-', original_string)
print(f"Replaced forward slashes with regex: {replaced_string_regex_forward}")
使用 re.sub() 方法将反斜杠替换为横杠
replaced_string_regex_backward = re.sub(r'\\', '-', original_string)
print(f"Replaced backward slashes with regex: {replaced_string_regex_backward}")
将所有斜杠替换为横杠
replaced_string_all_slashes = original_string.replace("/", "-").replace("\\", "-")
print(f"Replaced all slashes: {replaced_string_all_slashes}")
上述代码将输出:
Replaced forward slashes: Path-to-some-file-with\mixed-slashes
Replaced backward slashes: Path/to/some/file/with-mixed-slashes
Replaced forward slashes with regex: Path-to-some-file-with\mixed-slashes
Replaced backward slashes with regex: Path/to/some/file/with-mixed-slashes
Replaced all slashes: Path-to-some-file-with-mixed-slashes
通过以上方法,我们可以灵活、准确地替换字符串中的斜杠。根据具体需求选择合适的方法,可以提高代码的可读性和效率。
相关问答FAQs:
如何在Python中处理含有斜杠的字符串替换?
在Python中,处理包含斜杠的字符串时,可以使用str.replace()
方法来替换特定的子字符串。需要注意的是,在字符串中使用反斜杠(\
)时,要使用双反斜杠(\\
)来表示。例如,my_string.replace("\\", "/")
会将所有的反斜杠替换为正斜杠。
在替换含斜杠的字符串时,有哪些常用的库或方法可以使用?
除了基本的字符串替换方法,Python的re
模块提供了更灵活的正则表达式功能,可以用于复杂的替换操作。例如,使用re.sub()
函数可以匹配含有斜杠的模式并进行替换,允许使用更复杂的匹配规则,如re.sub(r'\\', '/', my_string)
。
在进行字符串替换时,如何避免不必要的错误或问题?
在处理包含斜杠的字符串替换时,确保对字符串进行适当的转义是非常重要的。如果输入字符串来自用户输入或文件,最好先验证或清洗输入数据,以避免因意外的斜杠而导致的错误。此外,使用try-except
语句来捕捉可能出现的异常也是一个良好的实践。
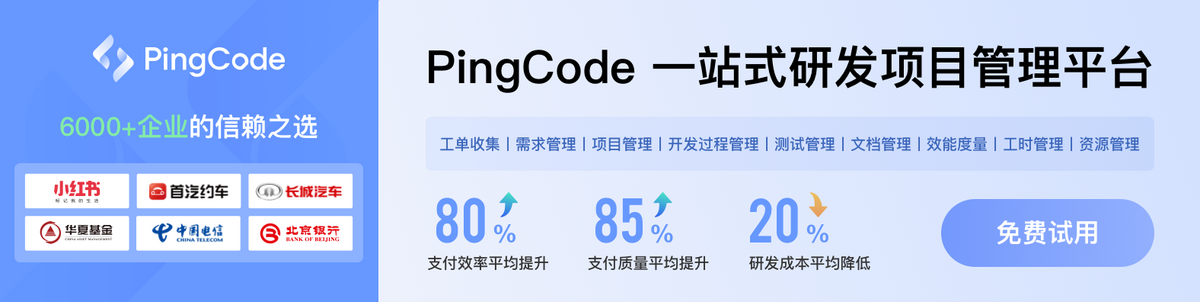