Python中打开文件夹下的文件的方法有多种,主要包括:使用os模块、使用glob模块、使用pathlib模块、使用open函数等。在本文中,我们将详细讨论这些方法并提供代码示例和注意事项。
一、使用os模块
os模块是Python内置的一个与操作系统交互的模块,其中包含了处理文件和文件夹的功能。使用os模块可以轻松地打开文件夹下的文件。
import os
获取文件夹下的所有文件
def list_files_in_directory(directory):
try:
files = os.listdir(directory)
return files
except FileNotFoundError:
print(f"The directory {directory} does not exist.")
return []
打开并读取文件内容
def read_file_content(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file {file_path} does not exist.")
except IOError:
print(f"Could not read file {file_path}.")
示例用法
directory_path = 'your_directory_path_here'
files = list_files_in_directory(directory_path)
for file_name in files:
file_path = os.path.join(directory_path, file_name)
content = read_file_content(file_path)
print(f"Content of {file_name}: {content}")
在上述代码中,我们使用了os.listdir
函数列出指定文件夹下的所有文件,并使用os.path.join
构建文件路径,然后使用open
函数打开并读取文件内容。
二、使用glob模块
glob模块提供了更灵活的文件路径匹配功能,适用于需要使用通配符匹配文件名的场景。
import glob
获取文件夹下的所有txt文件
def list_txt_files_in_directory(directory):
files = glob.glob(f"{directory}/*.txt")
return files
打开并读取文件内容
def read_file_content(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file {file_path} does not exist.")
except IOError:
print(f"Could not read file {file_path}.")
示例用法
directory_path = 'your_directory_path_here'
txt_files = list_txt_files_in_directory(directory_path)
for file_path in txt_files:
content = read_file_content(file_path)
print(f"Content of {file_path}: {content}")
在上述代码中,我们使用了glob.glob
函数匹配指定文件夹下的所有.txt
文件,并使用open
函数打开并读取文件内容。
三、使用pathlib模块
pathlib模块是Python 3.4引入的一个面向对象的路径处理模块,相较于os模块和glob模块,pathlib模块提供了更简洁和直观的文件操作方式。
from pathlib import Path
获取文件夹下的所有文件
def list_files_in_directory(directory):
try:
path = Path(directory)
files = list(path.iterdir())
return files
except FileNotFoundError:
print(f"The directory {directory} does not exist.")
return []
打开并读取文件内容
def read_file_content(file_path):
try:
with file_path.open('r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file {file_path} does not exist.")
except IOError:
print(f"Could not read file {file_path}.")
示例用法
directory_path = 'your_directory_path_here'
files = list_files_in_directory(directory_path)
for file_path in files:
content = read_file_content(file_path)
print(f"Content of {file_path}: {content}")
在上述代码中,我们使用了Path.iterdir
方法列出指定文件夹下的所有文件,并使用Path.open
方法打开并读取文件内容。
四、使用open函数
open函数是Python内置的一个打开文件的函数,虽然在上述方法中已经使用过,但这里单独列出是为了强调其重要性和灵活性。
# 打开并读取文件内容
def read_file_content(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file {file_path} does not exist.")
except IOError:
print(f"Could not read file {file_path}.")
示例用法
file_path = 'your_file_path_here'
content = read_file_content(file_path)
print(f"Content of {file_path}: {content}")
在上述代码中,我们直接使用open
函数打开并读取文件内容。
五、总结
在Python中打开文件夹下的文件,可以选择使用os模块、glob模块、pathlib模块或直接使用open函数。每种方法都有其优缺点,具体选择哪种方法取决于具体需求。os模块适合基本的文件操作,glob模块适合需要使用通配符匹配文件名的场景,pathlib模块提供了更简洁和直观的文件操作方式,而open函数则是最基础的文件操作函数。
无论使用哪种方法,都需要注意处理文件不存在或读取失败的情况,以确保代码的健壮性。在实际开发中,根据具体需求选择合适的方法,并结合异常处理,能够更好地实现文件操作功能。
相关问答FAQs:
如何在Python中列出文件夹中的所有文件?
在Python中,可以使用os
模块中的os.listdir()
方法来列出指定文件夹下的所有文件和子文件夹。例如,使用os.listdir('文件夹路径')
可以获取该目录下所有文件的名称,返回的结果是一个列表,您可以遍历这个列表来处理每个文件。
如何在Python中打开特定类型的文件?
如果您希望在Python中只打开特定类型的文件(如文本文件或CSV文件),可以结合使用os
模块和fnmatch
模块来筛选文件。例如,可以使用fnmatch.filter()
方法来匹配特定扩展名的文件。这样,您可以轻松处理文件夹中所需类型的文件。
如何使用Python读取文件内容?
在打开文件后,您可以使用open()
函数来读取文件内容。通过指定文件路径和模式(如'r'表示只读),可以打开文件。使用read()
方法可以一次性读取整个文件内容,而readlines()
则可以将文件的每一行作为一个列表元素返回。这样,您便能够对文件内容进行进一步的处理和分析。
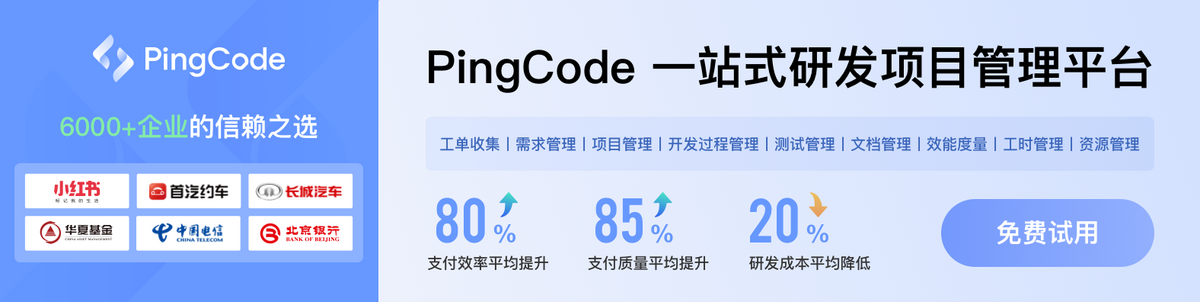