在Python中,取前三个数的方法有很多种,常见的方法包括使用切片、sorted函数和heapq库。 其中,使用切片 是最简单和最直观的方法。切片可以快速截取列表中的前三个元素。接下来,我将详细介绍如何使用这些方法来取列表中的前三个数。
一、使用切片
切片是Python中非常强大的功能,可以用来截取列表中的任意部分。要取列表中的前三个数,可以使用切片操作符[:]。例如:
my_list = [5, 2, 8, 1, 3]
top_three = my_list[:3]
print(top_three) # 输出: [5, 2, 8]
在这个例子中,my_list[:3]
表示从列表my_list
的开始位置到第3个元素(不包括第3个元素),因此可以得到列表的前三个数。
二、使用sorted函数
如果需要从一个无序列表中取出前三个最大值或最小值,可以使用sorted
函数。sorted
函数可以将列表按照指定的顺序进行排序,然后再取前三个数。
- 取前三个最大值:
my_list = [5, 2, 8, 1, 3]
top_three = sorted(my_list, reverse=True)[:3]
print(top_three) # 输出: [8, 5, 3]
在这个例子中,sorted(my_list, reverse=True)
会将列表按从大到小的顺序排序,然后再用切片操作符取前三个数。
- 取前三个最小值:
my_list = [5, 2, 8, 1, 3]
top_three = sorted(my_list)[:3]
print(top_three) # 输出: [1, 2, 3]
在这个例子中,sorted(my_list)
会将列表按从小到大的顺序排序,然后再用切片操作符取前三个数。
三、使用heapq库
heapq
是Python的一个内置库,专门用于堆操作。使用heapq
库可以高效地取出列表中的前三个最大值或最小值。
- 取前三个最大值:
import heapq
my_list = [5, 2, 8, 1, 3]
top_three = heapq.nlargest(3, my_list)
print(top_three) # 输出: [8, 5, 3]
在这个例子中,heapq.nlargest(3, my_list)
会返回列表中最大的三个元素。
- 取前三个最小值:
import heapq
my_list = [5, 2, 8, 1, 3]
top_three = heapq.nsmallest(3, my_list)
print(top_three) # 输出: [1, 2, 3]
在这个例子中,heapq.nsmallest(3, my_list)
会返回列表中最小的三个元素。
四、使用循环和条件语句
在某些情况下,你可能需要自定义逻辑来取出前三个数,这时可以使用循环和条件语句。例如:
my_list = [5, 2, 8, 1, 3]
top_three = []
for num in my_list:
if len(top_three) < 3:
top_three.append(num)
else:
min_top = min(top_three)
if num > min_top:
top_three.remove(min_top)
top_three.append(num)
print(top_three) # 输出: [5, 8, 3]
在这个例子中,我们通过遍历列表中的每个元素,并使用条件语句来决定是否将其加入到top_three
列表中,从而实现了取出前三个数的功能。
五、结合使用numpy和pandas库
对于数据分析和科学计算,numpy
和pandas
库提供了更为强大的功能,可以方便地操作数组和数据框。
- 使用numpy库:
import numpy as np
my_array = np.array([5, 2, 8, 1, 3])
top_three = np.sort(my_array)[-3:][::-1]
print(top_three) # 输出: [8, 5, 3]
在这个例子中,np.sort(my_array)
会对数组进行排序,然后通过切片操作符取出最后三个元素,并使用[::-1]将其逆序排列。
- 使用pandas库:
import pandas as pd
my_series = pd.Series([5, 2, 8, 1, 3])
top_three = my_series.nlargest(3)
print(top_three) # 输出:
2 8
0 5
4 3
dtype: int64
在这个例子中,my_series.nlargest(3)
会返回pandas
系列中最大的三个元素及其索引。
六、使用自定义函数
有时,您可能需要创建一个自定义函数来处理特定的逻辑。以下是一个示例,演示了如何编写一个函数来取出列表中的前三个数:
def get_top_three(numbers):
if len(numbers) < 3:
return sorted(numbers, reverse=True)
top_three = []
for num in numbers:
if len(top_three) < 3:
top_three.append(num)
else:
min_top = min(top_three)
if num > min_top:
top_three.remove(min_top)
top_three.append(num)
return sorted(top_three, reverse=True)
my_list = [5, 2, 8, 1, 3]
top_three = get_top_three(my_list)
print(top_three) # 输出: [8, 5, 3]
在这个例子中,我们定义了一个名为get_top_three
的函数,该函数接受一个数字列表作为输入,并返回最大的三个数。函数首先检查列表的长度是否小于3,如果是,则直接返回排序后的列表。否则,通过遍历列表并使用条件语句来决定是否将元素加入到top_three
列表中。
七、处理包含重复值的列表
在某些情况下,列表中可能包含重复值。如果希望取出的前三个数中不包含重复值,可以使用set
数据结构。例如:
my_list = [5, 2, 8, 1, 3, 2, 8]
unique_list = list(set(my_list))
top_three = sorted(unique_list, reverse=True)[:3]
print(top_three) # 输出: [8, 5, 3]
在这个例子中,我们首先使用set
将列表中的重复值去除,然后再使用排序和切片操作符取出前三个数。
八、处理空列表和异常情况
在实际使用中,还需要考虑列表为空或其他异常情况。例如:
def get_top_three(numbers):
if not numbers:
return []
unique_list = list(set(numbers))
return sorted(unique_list, reverse=True)[:3]
my_list = []
top_three = get_top_three(my_list)
print(top_three) # 输出: []
在这个例子中,我们添加了一个检查列表是否为空的条件,如果为空,则返回一个空列表。
通过以上几种方法,我们可以灵活地在Python中取出列表中的前三个数。选择哪种方法取决于具体的需求和场景。在实际应用中,可以根据数据的特点和操作的复杂程度,选择最适合的方法来完成任务。
相关问答FAQs:
在Python中,如何从列表中提取前三个元素?
要从一个列表中提取前三个元素,可以使用切片方法。比如,假设有一个列表numbers = [1, 2, 3, 4, 5]
,你可以通过numbers[:3]
获得前三个元素,结果将是[1, 2, 3]
。切片操作非常直观且简洁。
如何确保提取前三个数时不超过列表的长度?
在提取前三个数时,列表的长度可能小于三。为了安全提取,切片操作会自动处理这种情况。例如,若列表为numbers = [1]
,使用numbers[:3]
仍然会返回[1]
,而不会引发错误。因此,直接使用切片是一个安全的选择。
在处理字符串时,如何获取前三个字符?
对于字符串同样可以使用切片。例如,若有字符串text = "Hello, World!"
,可以通过text[:3]
获得前三个字符,结果为'Hel'
。这种方法对于字符串的处理非常方便,适用于各种场景。
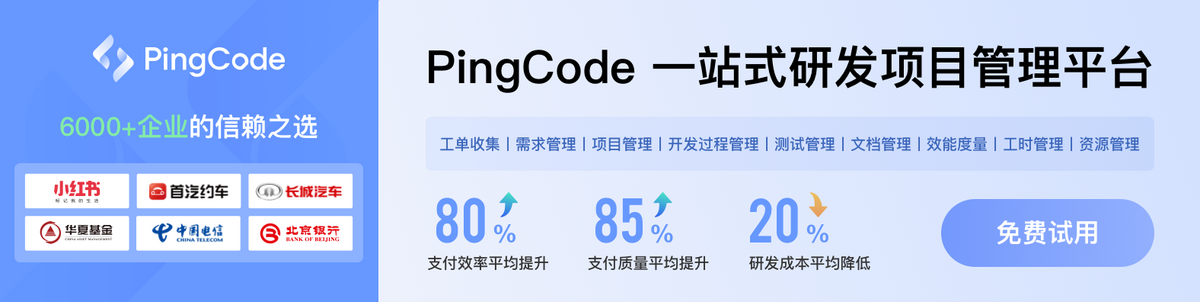