PYTHON中如何读取TXT数字进行使用
在Python中,读取TXT文件中的数字并进行使用是一个常见的需求。读取文件、处理数据、数据转换、错误处理是实现这一需求的关键步骤。以下是详细的步骤和方法。
一、读取文件
在Python中,可以使用内置的open()
函数来读取文件。此函数可以打开文件并返回文件对象。以下是一个简单的例子:
with open('filename.txt', 'r') as file:
data = file.read()
在这个例子中,filename.txt
是要读取的文件名,'r'
表示以只读模式打开文件。file.read()
将文件内容读取到字符串变量data
中。
二、处理数据
读取文件后,需要处理数据以提取数字。假设文件中的数字是按行排列的,可以使用splitlines()
方法将每一行分割成一个列表元素:
lines = data.splitlines()
lines
是一个列表,每个元素是文件中的一行。
三、数据转换
接下来,需要将提取的字符串转换为数字。可以使用int()
或float()
函数进行转换:
numbers = [float(line) for line in lines]
在这个例子中,float(line)
将每一行的字符串转换为浮点数。
四、错误处理
在处理文件和数据转换过程中,可能会遇到各种错误。可以使用try
和except
块来捕获并处理这些错误:
numbers = []
with open('filename.txt', 'r') as file:
lines = file.read().splitlines()
for line in lines:
try:
numbers.append(float(line))
except ValueError:
print(f"Skipping line: {line}")
在这个例子中,如果某一行无法转换为浮点数,将会跳过该行并打印警告信息。
一、读取文件的详细步骤
- 打开文件
使用open()
函数打开文件时,可以指定不同的模式,如只读模式'r'
、写入模式'w'
、追加模式'a'
等。通常,读取文件时使用只读模式。
file = open('filename.txt', 'r')
- 读取文件内容
可以使用file.read()
方法读取整个文件内容,也可以使用file.readline()
逐行读取,或使用file.readlines()
读取所有行并返回一个列表。
data = file.read()
- 关闭文件
读取文件内容后,应关闭文件以释放资源。
file.close()
为确保文件始终能正确关闭,建议使用with
语句,它会在代码块执行完毕后自动关闭文件。
with open('filename.txt', 'r') as file:
data = file.read()
二、处理数据的详细步骤
- 按行分割
使用splitlines()
方法将文件内容按行分割成列表。
lines = data.splitlines()
- 清理和过滤数据
在处理数据时,可能需要清理和过滤空行或无效数据。例如,可以使用列表推导式过滤掉空行:
lines = [line for line in lines if line.strip()]
三、数据转换的详细步骤
- 转换为数字
使用int()
或float()
函数将字符串转换为数字。可以使用列表推导式将所有行转换为数字:
numbers = [float(line) for line in lines]
- 处理异常
在转换过程中,可能会遇到无法转换的行。使用try
和except
块处理这些异常,并记录或跳过无效行。
numbers = []
for line in lines:
try:
numbers.append(float(line))
except ValueError:
print(f"Skipping invalid line: {line}")
四、错误处理的详细步骤
- 捕获文件读取错误
在打开和读取文件时,可能会遇到文件不存在或无法访问的错误。可以使用try
和except
块捕获这些错误:
try:
with open('filename.txt', 'r') as file:
data = file.read()
except FileNotFoundError:
print("File not found.")
except IOError:
print("Error reading file.")
- 捕获数据转换错误
在转换数据时,可能会遇到无法转换的行。使用try
和except
块捕获这些错误并处理:
numbers = []
with open('filename.txt', 'r') as file:
lines = file.read().splitlines()
for line in lines:
try:
numbers.append(float(line))
except ValueError:
print(f"Skipping invalid line: {line}")
五、综合实例
以下是一个综合实例,展示如何读取TXT文件中的数字并进行处理:
def read_numbers_from_file(filename):
numbers = []
try:
with open(filename, 'r') as file:
lines = file.read().splitlines()
for line in lines:
try:
numbers.append(float(line))
except ValueError:
print(f"Skipping invalid line: {line}")
except FileNotFoundError:
print("File not found.")
except IOError:
print("Error reading file.")
return numbers
使用示例
filename = 'numbers.txt'
numbers = read_numbers_from_file(filename)
print(numbers)
这个实例展示了如何读取文件、处理数据、进行数据转换和处理错误。read_numbers_from_file
函数接受一个文件名作为参数,读取文件中的数字并返回一个数字列表。
六、常见问题和解决方案
- 文件编码问题
有时文件可能使用不同的编码格式,如UTF-8或ISO-8859-1。如果读取文件时遇到编码错误,可以指定文件编码:
with open('filename.txt', 'r', encoding='utf-8') as file:
data = file.read()
- 大文件处理
如果文件非常大,读取整个文件内容可能会导致内存不足。可以逐行读取文件并处理每一行:
numbers = []
with open('filename.txt', 'r') as file:
for line in file:
try:
numbers.append(float(line.strip()))
except ValueError:
print(f"Skipping invalid line: {line}")
- 数据格式问题
有时文件中的数字可能包含逗号或其他分隔符。可以使用正则表达式或字符串操作方法清理数据:
import re
numbers = []
with open('filename.txt', 'r') as file:
for line in file:
line = re.sub(r'[^\d.]', '', line) # 移除非数字和小数点的字符
try:
numbers.append(float(line))
except ValueError:
print(f"Skipping invalid line: {line}")
七、应用实例
- 计算统计量
读取数字后,可以进行各种统计计算,如求和、平均值、最大值和最小值:
numbers = read_numbers_from_file('numbers.txt')
total = sum(numbers)
average = total / len(numbers)
maximum = max(numbers)
minimum = min(numbers)
print(f"Total: {total}")
print(f"Average: {average}")
print(f"Maximum: {maximum}")
print(f"Minimum: {minimum}")
- 绘制图表
可以使用matplotlib
库绘制数字的图表,如折线图或直方图:
import matplotlib.pyplot as plt
numbers = read_numbers_from_file('numbers.txt')
plt.plot(numbers)
plt.title('Numbers from File')
plt.xlabel('Index')
plt.ylabel('Value')
plt.show()
- 数据分析
可以使用pandas
库进行更复杂的数据分析和处理:
import pandas as pd
numbers = read_numbers_from_file('numbers.txt')
df = pd.DataFrame(numbers, columns=['Value'])
summary = df.describe()
print(summary)
八、总结
通过以上步骤和方法,可以轻松地在Python中读取TXT文件中的数字并进行各种处理和分析。关键步骤包括读取文件、处理数据、数据转换和错误处理。掌握这些技巧后,可以高效地处理文件中的数字数据,并应用于各种实际场景中。
无论是简单的统计计算还是复杂的数据分析,Python提供了强大的工具和库,使这一过程变得简单而高效。希望本文能为您提供有价值的指导,帮助您在Python中更好地读取和处理TXT文件中的数字。
相关问答FAQs:
如何在Python中读取txt文件中的数字?
在Python中,可以使用内置的open()
函数来打开txt文件,并利用readlines()
或read()
方法读取文件内容。读取后,可以使用split()
方法将字符串分割成数字,并使用int()
或float()
函数将其转换为相应的数字类型。以下是一个简单的示例代码:
with open('numbers.txt', 'r') as file:
numbers = [float(line.strip()) for line in file.readlines()]
读取的数字数据是否可以进行数学运算?
是的,读取的数字数据可以进行各种数学运算。将数据存储为列表或其他数据结构后,可以使用Python的标准运算符和数学函数进行处理。例如,可以计算总和、平均值或进行其他统计分析,代码示例如下:
total = sum(numbers)
average = total / len(numbers) if numbers else 0
如何处理txt文件中可能存在的非数字内容?
在读取txt文件时,可能会遇到非数字内容。为了避免错误,可以在转换时添加异常处理,例如使用try-except
语句。这样可以确保程序不会因为单个无效数据而崩溃。示例代码如下:
numbers = []
with open('numbers.txt', 'r') as file:
for line in file:
try:
numbers.append(float(line.strip()))
except ValueError:
print(f"忽略无效数据: {line.strip()}")
通过以上方式,可以有效读取和处理txt文件中的数字数据。
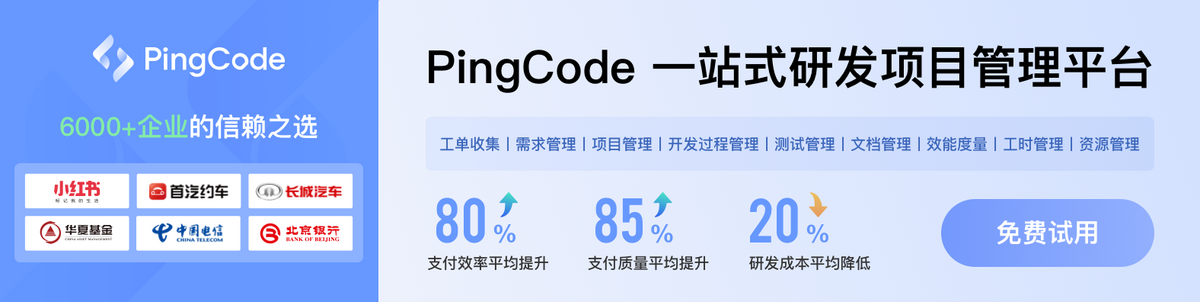