如何用PHP调用Python脚本文件内容
要在PHP中调用Python脚本文件,可以通过几种不同的方法来实现:使用exec
函数、shell_exec
函数、或者使用proc_open
函数。其中最常用的方法是使用exec
函数、shell_exec
函数、传递参数和获取返回值。下面将详细介绍如何通过这些方法实现PHP调用Python脚本,并对具体实现进行详细展开。
一、使用exec
函数调用Python脚本
exec
函数是PHP中用于执行外部程序的函数。可以通过该函数来调用Python脚本,并获取脚本的输出结果。
<?php
$command = escapeshellcmd('python script.py');
$output = exec($command);
echo $output;
?>
在上面的代码中,escapeshellcmd
函数用于转义命令行参数,以防止命令注入攻击。exec
函数执行了命令并将输出结果存储在$output
变量中。最后,通过echo
函数输出结果。
二、使用shell_exec
函数调用Python脚本
shell_exec
函数是另一个可以在PHP中执行外部程序的函数。与exec
函数不同的是,shell_exec
函数返回整个命令的输出,而不是最后一行输出。
<?php
$output = shell_exec('python script.py');
echo $output;
?>
在上面的代码中,shell_exec
函数执行了命令并将输出结果存储在$output
变量中。最后,通过echo
函数输出结果。
三、使用proc_open
函数调用Python脚本
proc_open
函数提供了更高级的接口,可以在PHP中创建一个子进程并与其通信。可以通过该函数来调用Python脚本,并传递参数和获取返回值。
<?php
$descriptorspec = array(
0 => array("pipe", "r"), // stdin is a pipe that the child will read from
1 => array("pipe", "w"), // stdout is a pipe that the child will write to
2 => array("pipe", "w") // stderr is a pipe that the child will write to
);
$process = proc_open('python script.py', $descriptorspec, $pipes);
if (is_resource($process)) {
// Write to stdin
fwrite($pipes[0], 'input data');
fclose($pipes[0]);
// Read from stdout
$output = stream_get_contents($pipes[1]);
fclose($pipes[1]);
// Read from stderr
$error = stream_get_contents($pipes[2]);
fclose($pipes[2]);
// Close the process
$return_value = proc_close($process);
echo "Output: $output\n";
echo "Error: $error\n";
echo "Return value: $return_value\n";
}
?>
在上面的代码中,$descriptorspec
数组定义了子进程的三个文件描述符:标准输入、标准输出和标准错误。proc_open
函数创建了一个子进程并返回一个进程资源。通过该资源,可以与子进程进行通信。最后,通过proc_close
函数关闭子进程,并获取返回值。
四、传递参数和获取返回值
在实际应用中,可能需要向Python脚本传递参数,并获取脚本的返回值。可以通过命令行参数来实现这一功能。
<?php
$arg1 = 'value1';
$arg2 = 'value2';
$command = escapeshellcmd("python script.py $arg1 $arg2");
$output = shell_exec($command);
echo $output;
?>
在上面的代码中,通过将参数添加到命令行中,可以向Python脚本传递参数。Python脚本可以通过sys.argv
获取这些参数。
import sys
arg1 = sys.argv[1]
arg2 = sys.argv[2]
print(f'Argument 1: {arg1}')
print(f'Argument 2: {arg2}')
在Python脚本中,通过sys.argv
获取命令行参数,并进行处理。
五、使用Python脚本处理输入输出
在某些情况下,可能需要通过标准输入和标准输出与Python脚本进行交互。可以通过proc_open
函数实现这一功能。
<?php
$descriptorspec = array(
0 => array("pipe", "r"), // stdin is a pipe that the child will read from
1 => array("pipe", "w"), // stdout is a pipe that the child will write to
2 => array("pipe", "w") // stderr is a pipe that the child will write to
);
$process = proc_open('python script.py', $descriptorspec, $pipes);
if (is_resource($process)) {
// Write to stdin
fwrite($pipes[0], 'input data');
fclose($pipes[0]);
// Read from stdout
$output = stream_get_contents($pipes[1]);
fclose($pipes[1]);
// Read from stderr
$error = stream_get_contents($pipes[2]);
fclose($pipes[2]);
// Close the process
$return_value = proc_close($process);
echo "Output: $output\n";
echo "Error: $error\n";
echo "Return value: $return_value\n";
}
?>
在上面的代码中,通过向标准输入写入数据,可以将数据传递给Python脚本。Python脚本可以通过sys.stdin
读取标准输入的数据。
import sys
input_data = sys.stdin.read()
print(f'Input data: {input_data}')
在Python脚本中,通过sys.stdin.read
读取标准输入的数据,并进行处理。
六、处理Python脚本的输出和错误
在实际应用中,可能需要处理Python脚本的输出和错误。可以通过proc_open
函数获取标准输出和标准错误,并进行处理。
<?php
$descriptorspec = array(
0 => array("pipe", "r"), // stdin is a pipe that the child will read from
1 => array("pipe", "w"), // stdout is a pipe that the child will write to
2 => array("pipe", "w") // stderr is a pipe that the child will write to
);
$process = proc_open('python script.py', $descriptorspec, $pipes);
if (is_resource($process)) {
// Write to stdin
fwrite($pipes[0], 'input data');
fclose($pipes[0]);
// Read from stdout
$output = stream_get_contents($pipes[1]);
fclose($pipes[1]);
// Read from stderr
$error = stream_get_contents($pipes[2]);
fclose($pipes[2]);
// Close the process
$return_value = proc_close($process);
// Process the output and error
if ($return_value === 0) {
echo "Output: $output\n";
} else {
echo "Error: $error\n";
}
}
?>
在上面的代码中,通过读取标准输出和标准错误,可以获取Python脚本的输出和错误信息。根据返回值,判断脚本是否执行成功,并进行相应的处理。
七、使用JSON格式进行数据交换
在实际应用中,可能需要在PHP和Python之间传递复杂的数据结构。可以使用JSON格式进行数据交换。
<?php
$data = array('key1' => 'value1', 'key2' => 'value2');
$json_data = json_encode($data);
$descriptorspec = array(
0 => array("pipe", "r"), // stdin is a pipe that the child will read from
1 => array("pipe", "w"), // stdout is a pipe that the child will write to
2 => array("pipe", "w") // stderr is a pipe that the child will write to
);
$process = proc_open('python script.py', $descriptorspec, $pipes);
if (is_resource($process)) {
// Write to stdin
fwrite($pipes[0], $json_data);
fclose($pipes[0]);
// Read from stdout
$output = stream_get_contents($pipes[1]);
fclose($pipes[1]);
// Read from stderr
$error = stream_get_contents($pipes[2]);
fclose($pipes[2]);
// Close the process
$return_value = proc_close($process);
// Process the output and error
if ($return_value === 0) {
$response_data = json_decode($output, true);
print_r($response_data);
} else {
echo "Error: $error\n";
}
}
?>
在上面的代码中,通过json_encode
函数将数据转换为JSON格式,并通过标准输入传递给Python脚本。Python脚本可以通过sys.stdin
读取标准输入的数据,并使用json.loads
函数解析JSON数据。
import sys
import json
input_data = sys.stdin.read()
data = json.loads(input_data)
print(json.dumps(data))
在Python脚本中,通过sys.stdin.read
读取标准输入的数据,并使用json.loads
函数解析JSON数据。通过json.dumps
函数将数据转换为JSON格式,并输出结果。
八、处理不同的Python版本
在实际应用中,可能需要处理不同的Python版本。可以通过指定Python解释器的路径来确保使用正确的版本。
<?php
$python_path = '/usr/bin/python3'; // Specify the path to the Python interpreter
$command = escapeshellcmd("$python_path script.py");
$output = shell_exec($command);
echo $output;
?>
在上面的代码中,通过指定Python解释器的路径,可以确保使用正确的版本。可以通过which python
命令找到Python解释器的路径。
九、处理多线程和异步调用
在某些情况下,可能需要处理多线程和异步调用。可以使用PHP的pcntl_fork
函数来创建子进程,并使用Python的asyncio
模块来实现异步调用。
<?php
$pid = pcntl_fork();
if ($pid == -1) {
// Fork failed
die('could not fork');
} else if ($pid) {
// Parent process
echo "Parent process\n";
pcntl_wait($status); // Wait for child process to finish
} else {
// Child process
$command = escapeshellcmd('python script.py');
$output = shell_exec($command);
echo $output;
}
?>
在上面的代码中,通过pcntl_fork
函数创建子进程,并在子进程中调用Python脚本。父进程等待子进程完成。
import asyncio
async def main():
print('Hello, world!')
asyncio.run(main())
在Python脚本中,使用asyncio
模块实现异步调用。
十、总结
通过本文的介绍,详细介绍了在PHP中调用Python脚本的几种常用方法,包括使用exec
函数、shell_exec
函数、proc_open
函数,传递参数和获取返回值,处理输入输出,处理输出和错误,使用JSON格式进行数据交换,处理不同的Python版本,处理多线程和异步调用。希望通过这些方法的介绍,能够帮助开发者在实际应用中更好地实现PHP调用Python脚本的功能。
总之,在PHP中调用Python脚本是一种常见的需求,可以通过多种方法实现,包括使用exec
函数、shell_exec
函数、proc_open
函数,传递参数和获取返回值,处理输入输出,处理输出和错误,使用JSON格式进行数据交换,处理不同的Python版本,处理多线程和异步调用。通过这些方法,可以在实际应用中更好地实现PHP与Python的集成,提高开发效率和代码质量。
相关问答FAQs:
如何在PHP中执行Python脚本并获取输出?
在PHP中调用Python脚本可以通过使用exec()
、shell_exec()
或system()
函数来实现。这些函数允许你执行外部命令并捕获输出。你需要确保Python脚本的路径正确,并且有执行权限。以下是一个简单的示例:
$output = shell_exec('python3 /path/to/your_script.py');
echo $output;
确保将/path/to/your_script.py
替换为你的Python脚本实际路径。
PHP和Python交互时需要注意哪些安全问题?
在调用Python脚本时,务必注意输入的安全性。确保传递给Python的任何参数都经过验证和清理,以防止命令注入等安全漏洞。此外,考虑使用escapeshellarg()
函数来安全处理用户输入,保护你的系统不受潜在攻击。
在PHP中如何传递参数给Python脚本?
你可以通过命令行参数的方式将数据传递给Python脚本。在PHP中,将参数附加到执行命令的字符串中。例如:
$arg1 = 'value1';
$arg2 = 'value2';
$output = shell_exec("python3 /path/to/your_script.py $arg1 $arg2");
echo $output;
在Python脚本中,可以使用sys.argv
来获取这些参数:
import sys
arg1 = sys.argv[1]
arg2 = sys.argv[2]
这样可以实现两种语言间的数据传递。
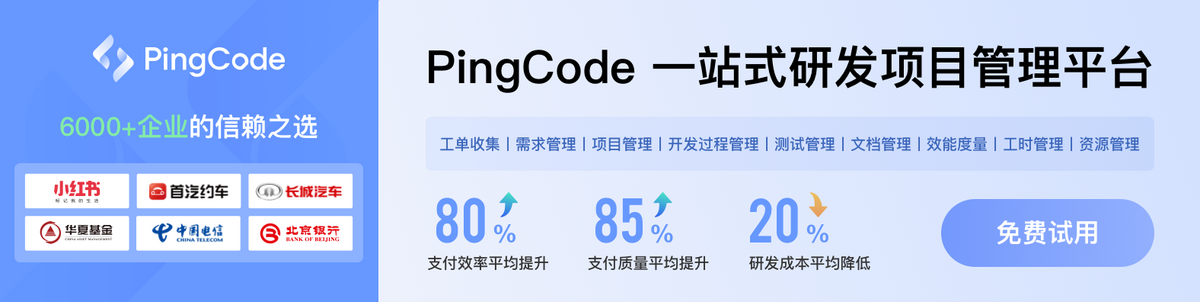