如何将Python和MySQL数据库连接
Python和MySQL数据库连接的主要步骤包括安装MySQL数据库、安装Python的MySQL连接器、配置数据库连接参数、使用SQL语句进行数据操作,这些步骤使得Python程序能够与MySQL数据库进行交互。 其中,安装MySQL连接器是关键,因为它是Python与MySQL通信的桥梁。
在详细描述如何安装MySQL连接器之前,我们需要先了解整个过程的大致步骤。以下是Python和MySQL数据库连接的详细指南:
一、安装MySQL数据库
在开始之前,首先需要在你的系统上安装MySQL数据库。无论你使用的是Windows、Mac还是Linux,MySQL的安装步骤大致相同。
- 下载MySQL:前往MySQL官方网站(https://dev.mysql.com/downloads/)下载适用于你操作系统的MySQL安装包。
- 安装MySQL:按照下载页面上的说明进行安装,并记住你的MySQL root用户的密码。
- 启动MySQL服务:安装完成后,确保MySQL服务正在运行。你可以通过命令行或系统服务管理器来启动MySQL服务。
二、安装Python的MySQL连接器
Python有多个库可以与MySQL数据库交互,其中最常用的是mysql-connector-python
和PyMySQL
。这里我们使用mysql-connector-python
进行示范。
- 安装mysql-connector-python:打开命令行工具,输入以下命令来安装连接器:
pip install mysql-connector-python
该命令将从Python包索引(PyPI)中下载并安装mysql-connector-python库。
三、配置数据库连接参数
在安装好MySQL数据库和连接器之后,你需要配置数据库连接参数。这些参数包括数据库主机地址、用户名、密码和数据库名称。
import mysql.connector
config = {
'user': 'root',
'password': 'yourpassword',
'host': '127.0.0.1',
'database': 'yourdatabase',
'raise_on_warnings': True
}
四、连接到MySQL数据库
使用上述配置参数,你可以在Python脚本中连接到MySQL数据库:
import mysql.connector
from mysql.connector import errorcode
try:
cnx = mysql.connector.connect(config)
print("Connection established.")
except mysql.connector.Error as err:
if err.errno == errorcode.ER_ACCESS_DENIED_ERROR:
print("Something is wrong with your user name or password")
elif err.errno == errorcode.ER_BAD_DB_ERROR:
print("Database does not exist")
else:
print(err)
else:
cnx.close()
在上面的代码中,我们尝试连接到MySQL数据库,并在连接成功或失败时打印相应的消息。
五、使用SQL语句进行数据操作
连接到数据库之后,你可以执行各种SQL语句来操作数据。以下是一些常见的操作示例。
插入数据
cursor = cnx.cursor()
add_employee = ("INSERT INTO employees "
"(first_name, last_name, hire_date, gender, birth_date) "
"VALUES (%s, %s, %s, %s, %s)")
data_employee = ('John', 'Doe', '2023-01-01', 'M', '1990-01-01')
cursor.execute(add_employee, data_employee)
cnx.commit()
cursor.close()
查询数据
cursor = cnx.cursor()
query = ("SELECT first_name, last_name, hire_date FROM employees "
"WHERE hire_date BETWEEN %s AND %s")
hire_start = '2023-01-01'
hire_end = '2023-12-31'
cursor.execute(query, (hire_start, hire_end))
for (first_name, last_name, hire_date) in cursor:
print(f"{first_name} {last_name} was hired on {hire_date}")
cursor.close()
更新数据
cursor = cnx.cursor()
update_employee = ("UPDATE employees SET last_name = %s "
"WHERE first_name = %s")
data_employee = ('Smith', 'John')
cursor.execute(update_employee, data_employee)
cnx.commit()
cursor.close()
删除数据
cursor = cnx.cursor()
delete_employee = ("DELETE FROM employees WHERE first_name = %s")
data_employee = ('John',)
cursor.execute(delete_employee, data_employee)
cnx.commit()
cursor.close()
六、关闭数据库连接
在完成所有数据库操作后,确保关闭数据库连接:
cnx.close()
七、错误处理和调试
在实际开发中,处理错误和调试代码是非常重要的。使用mysql.connector
库时,可以通过捕获异常来处理错误,例如:
try:
cnx = mysql.connector.connect(config)
except mysql.connector.Error as err:
print(f"Error: {err}")
cnx.close()
此外,调试代码时可以使用print
语句来输出调试信息,或者使用Python的内置调试器(如pdb
)。
八、使用ORM(对象关系映射)
如果你习惯使用ORM来操作数据库,可以尝试使用SQLAlchemy,它提供了更加高级和便捷的方式来与数据库交互。
安装SQLAlchemy
pip install SQLAlchemy
使用SQLAlchemy连接MySQL
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
DATABASE_URL = "mysql+mysqlconnector://root:yourpassword@127.0.0.1/yourdatabase"
engine = create_engine(DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
定义模型和操作数据
from sqlalchemy import Column, Integer, String, Date
class Employee(Base):
__tablename__ = 'employees'
id = Column(Integer, primary_key=True, index=True)
first_name = Column(String(50))
last_name = Column(String(50))
hire_date = Column(Date)
gender = Column(String(1))
birth_date = Column(Date)
创建表
Base.metadata.create_all(bind=engine)
插入数据
session = SessionLocal()
new_employee = Employee(first_name='Jane', last_name='Doe', hire_date='2023-01-01', gender='F', birth_date='1990-01-01')
session.add(new_employee)
session.commit()
查询数据
employees = session.query(Employee).filter(Employee.hire_date.between('2023-01-01', '2023-12-31')).all()
for employee in employees:
print(f"{employee.first_name} {employee.last_name} was hired on {employee.hire_date}")
session.close()
通过以上步骤,你可以使用Python和MySQL数据库进行各种数据操作。无论是使用原生的mysql-connector-python
库,还是使用更高级的SQLAlchemy ORM,Python都提供了灵活和强大的数据库操作能力。希望这篇文章能帮助你顺利实现Python与MySQL数据库的连接和操作。
相关问答FAQs:
如何在Python中连接MySQL数据库?
要在Python中连接MySQL数据库,可以使用mysql-connector-python
或PyMySQL
等库。首先,确保已经安装所需的库,可以使用pip install mysql-connector-python
或pip install PyMySQL
进行安装。接下来,导入库并使用相应的连接函数,提供数据库的主机名、用户名、密码和数据库名。例如:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
成功连接后,您可以使用cursor
对象执行SQL查询。
在Python中如何执行MySQL查询?
一旦与数据库建立了连接,就可以使用cursor
对象来执行SQL查询。创建一个cursor
对象后,使用execute
方法执行查询,并可以通过fetchall()
或fetchone()
方法获取结果。例如:
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
for row in results:
print(row)
执行完查询后,记得关闭游标和连接以释放资源。
如何处理Python与MySQL交互中的异常?
在Python与MySQL交互时,处理异常是非常重要的。可以使用try-except
语句来捕获可能出现的错误,如连接失败、查询语法错误等。在except
块中,可以打印错误信息或进行其他处理。示例代码如下:
try:
connection = mysql.connector.connect(...)
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
cursor.close()
connection.close()
通过这种方式,可以确保即使发生错误,资源也会被妥善管理。
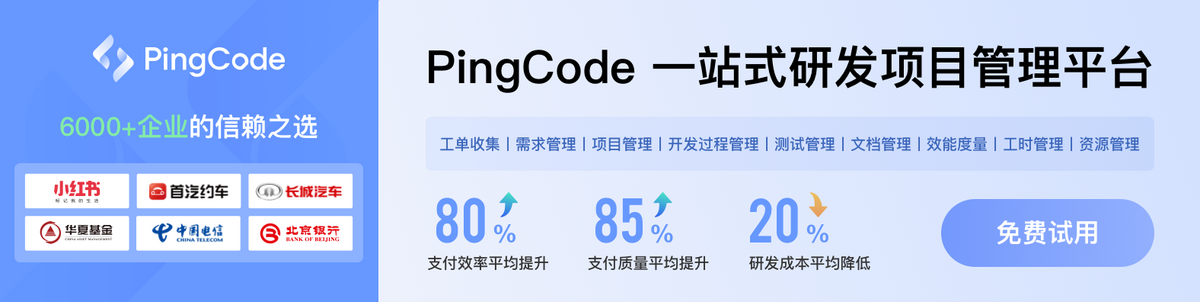