PYTHON如何使用百度地图API接口
Python使用百度地图API接口的核心步骤包括:获取API密钥、调用HTTP请求、处理返回数据、进行数据解析。其中,获取API密钥是第一步,它是调用百度地图API服务的凭证。下面我们将详细展开如何在Python中使用百度地图API接口。
一、获取API密钥
在使用百度地图API之前,首先需要在百度地图开发者平台上注册并获取API密钥。以下是详细步骤:
- 注册百度地图开发者账号:访问百度地图API官网(http://lbsyun.baidu.com/),注册一个开发者账号。
- 创建应用并获取密钥:登录后,进入“控制台”,创建一个新的应用,并获取API密钥(AK)。记下这个密钥,它将在所有API请求中使用。
二、调用HTTP请求
要使用百度地图API,需要通过HTTP请求来获取数据。Python中常用的HTTP请求库是requests
。以下是一个简单示例,演示如何调用百度地图API:
import requests
def get_location_info(address, api_key):
base_url = "http://api.map.baidu.com/geocoding/v3/"
params = {
"address": address,
"output": "json",
"ak": api_key
}
response = requests.get(base_url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
address = "北京市海淀区中关村"
location_info = get_location_info(address, api_key)
print(location_info)
三、处理返回数据
百度地图API返回的数据通常是JSON格式。需要对这些数据进行解析,以提取有用的信息。Python的json
库可以轻松完成这一任务。
import json
def parse_location_info(json_data):
if json_data['status'] == 0:
result = json_data['result']
location = result['location']
lat = location['lat']
lng = location['lng']
return lat, lng
else:
return None, None
使用示例
lat, lng = parse_location_info(location_info)
print(f"Latitude: {lat}, Longitude: {lng}")
四、进行数据解析
不同的API接口返回的数据格式可能不同,因此解析方法会有所不同。下面我们将详细介绍几种常用的百度地图API接口及其数据解析方法。
1、地理编码API
地理编码API用于将地址转换为经纬度坐标。其返回数据中包含详细的地理位置信息。
import requests
def geocode(address, api_key):
url = f"http://api.map.baidu.com/geocoding/v3/"
params = {
'address': address,
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
address = "北京市海淀区中关村"
result = geocode(address, api_key)
if result['status'] == 0:
location = result['result']['location']
print(f"Latitude: {location['lat']}, Longitude: {location['lng']}")
else:
print("Error:", result['msg'])
2、逆地理编码API
逆地理编码API用于将经纬度坐标转换为地址。其返回数据中包含详细的地址信息。
import requests
def reverse_geocode(lat, lng, api_key):
url = f"http://api.map.baidu.com/reverse_geocoding/v3/"
params = {
'location': f"{lat},{lng}",
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
lat, lng = 39.983424, 116.322987
result = reverse_geocode(lat, lng, api_key)
if result['status'] == 0:
address = result['result']['formatted_address']
print(f"Address: {address}")
else:
print("Error:", result['msg'])
3、路线规划API
路线规划API用于计算两个地点之间的路线,并返回详细的路线信息。
import requests
def direction(origin, destination, api_key):
url = f"http://api.map.baidu.com/direction/v2/driving"
params = {
'origin': origin,
'destination': destination,
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
origin = "39.983424,116.322987"
destination = "39.90816,116.397477"
result = direction(origin, destination, api_key)
if result['status'] == 0:
routes = result['result']['routes']
for route in routes:
print(f"Distance: {route['distance']} meters, Duration: {route['duration']} seconds")
else:
print("Error:", result['msg'])
4、地点搜索API
地点搜索API用于根据关键词搜索地点,并返回相关地点的信息。
import requests
def place_search(query, region, api_key):
url = f"http://api.map.baidu.com/place/v2/search"
params = {
'query': query,
'region': region,
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
query = "餐厅"
region = "北京"
result = place_search(query, region, api_key)
if result['status'] == 0:
places = result['results']
for place in places:
print(f"Name: {place['name']}, Address: {place['address']}")
else:
print("Error:", result['msg'])
五、错误处理与优化
在实际使用过程中,需要进行错误处理和优化,以提高程序的健壮性和性能。
1、错误处理
对于每个API请求,应该检查返回的状态码,以确定请求是否成功。如果请求失败,应该输出错误信息,并进行相应的处理。
def handle_api_response(response):
if response.status_code == 200:
json_data = response.json()
if json_data['status'] == 0:
return json_data
else:
print("API Error:", json_data['msg'])
return None
else:
print("HTTP Error:", response.status_code)
return None
2、优化请求
对于频繁调用的API请求,可以进行优化,例如使用缓存技术,以减少对API的调用次数,提高程序的性能。
import requests_cache
启用requests_cache
requests_cache.install_cache('baidu_cache', expire_after=1800)
def cached_geocode(address, api_key):
url = f"http://api.map.baidu.com/geocoding/v3/"
params = {
'address': address,
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
使用示例
api_key = "your_api_key_here"
address = "北京市海淀区中关村"
result = cached_geocode(address, api_key)
if result['status'] == 0:
location = result['result']['location']
print(f"Latitude: {location['lat']}, Longitude: {location['lng']}")
else:
print("Error:", result['msg'])
六、综合示例
以下是一个综合示例,演示如何结合上述API来实现一个完整的应用程序。
import requests
import requests_cache
启用requests_cache
requests_cache.install_cache('baidu_cache', expire_after=1800)
def get_location_info(address, api_key):
base_url = "http://api.map.baidu.com/geocoding/v3/"
params = {
"address": address,
"output": "json",
"ak": api_key
}
response = requests.get(base_url, params=params)
return response.json()
def parse_location_info(json_data):
if json_data['status'] == 0:
result = json_data['result']
location = result['location']
lat = location['lat']
lng = location['lng']
return lat, lng
else:
return None, None
def reverse_geocode(lat, lng, api_key):
url = f"http://api.map.baidu.com/reverse_geocoding/v3/"
params = {
'location': f"{lat},{lng}",
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
def direction(origin, destination, api_key):
url = f"http://api.map.baidu.com/direction/v2/driving"
params = {
'origin': origin,
'destination': destination,
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
def place_search(query, region, api_key):
url = f"http://api.map.baidu.com/place/v2/search"
params = {
'query': query,
'region': region,
'output': 'json',
'ak': api_key
}
response = requests.get(url, params=params)
return response.json()
主程序
api_key = "your_api_key_here"
address = "北京市海淀区中关村"
获取经纬度
location_info = get_location_info(address, api_key)
lat, lng = parse_location_info(location_info)
if lat and lng:
print(f"Latitude: {lat}, Longitude: {lng}")
# 逆地理编码
reverse_info = reverse_geocode(lat, lng, api_key)
if reverse_info['status'] == 0:
address = reverse_info['result']['formatted_address']
print(f"Address: {address}")
# 路线规划
origin = f"{lat},{lng}"
destination = "39.90816,116.397477"
direction_info = direction(origin, destination, api_key)
if direction_info['status'] == 0:
routes = direction_info['result']['routes']
for route in routes:
print(f"Distance: {route['distance']} meters, Duration: {route['duration']} seconds")
# 地点搜索
query = "餐厅"
region = "北京"
place_info = place_search(query, region, api_key)
if place_info['status'] == 0:
places = place_info['results']
for place in places:
print(f"Name: {place['name']}, Address: {place['address']}")
else:
print("Failed to get location info.")
通过以上步骤,您已经掌握了如何在Python中使用百度地图API接口。希望这些内容对您有所帮助!
相关问答FAQs:
如何在Python中获取百度地图API的密钥?
要使用百度地图API,您需要首先注册一个百度开发者账号,并创建一个应用来获取API密钥。登录百度云控制台,选择“地图服务”,然后创建应用,系统会生成一个API密钥。确保将此密钥保存在安全的地方,并在后续的API请求中使用。
Python如何发送HTTP请求到百度地图API?
在Python中,您可以使用requests
库发送HTTP请求。首先安装该库(如果尚未安装),然后使用requests.get()
方法构造请求URL并附上您的API密钥和所需参数。例如,您可以构建一个获取地理编码(将地址转换为坐标)的请求,URL格式为:http://api.map.baidu.com/geocoding/v3/?address=您的地址&output=json&ak=您的AK
。
如何解析百度地图API返回的JSON数据?
百度地图API返回的数据通常是JSON格式。在Python中,您可以使用json
库来解析这些数据。通过调用response.json()
方法,可以将返回的JSON字符串转换为Python字典。接下来,您可以通过字典的键获取所需的信息,例如经纬度、地址等。确保处理可能出现的错误,如地址未找到的情况,以确保程序的稳健性。
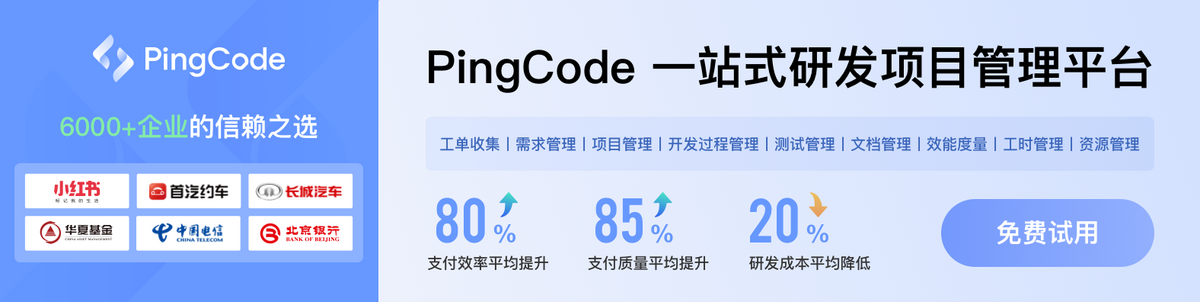