Python找出列表里的特定元素:使用列表方法、使用条件表达式、使用列表推导式、使用filter函数。其中,通过列表方法查找是最直接的方法,例如使用.index()
方法。
在Python中,找出列表里的特定元素可以通过多种方法实现。最直接的方法是使用列表的.index()
方法,这个方法会返回特定元素在列表中的第一个匹配项的索引。如果元素不存在,则会抛出一个ValueError
。例如:
my_list = [1, 2, 3, 4, 5]
element = 3
try:
index = my_list.index(element)
print(f"Element {element} found at index {index}")
except ValueError:
print(f"Element {element} not found in the list")
除了直接使用列表方法查找外,还可以通过条件表达式、列表推导式或filter
函数来实现更复杂的查找逻辑,下面我们将详细展开这些方法。
一、列表方法
1、使用 index()
方法
index()
方法是最常用的方法之一,它用于查找元素在列表中的第一个匹配项的索引。如果元素不存在,则会抛出一个 ValueError
。
my_list = ['apple', 'banana', 'cherry', 'date']
element = 'cherry'
try:
index = my_list.index(element)
print(f"Element {element} found at index {index}")
except ValueError:
print(f"Element {element} not found in the list")
2、使用 count()
方法
count()
方法可以用来确定元素在列表中出现的次数。如果返回值大于 0,则说明元素存在于列表中。
my_list = ['apple', 'banana', 'cherry', 'date', 'cherry']
element = 'cherry'
count = my_list.count(element)
if count > 0:
print(f"Element {element} appears {count} times in the list")
else:
print(f"Element {element} not found in the list")
二、条件表达式
条件表达式可以用于更灵活的查找逻辑。例如,查找列表中所有大于某个值的元素。
my_list = [10, 20, 30, 40, 50]
threshold = 25
elements = [x for x in my_list if x > threshold]
print(f"Elements greater than {threshold}: {elements}")
三、列表推导式
列表推导式是一种简洁的方式来创建新的列表。它可以用于查找符合条件的所有元素,并返回一个新的列表。
my_list = ['apple', 'banana', 'cherry', 'date']
element = 'cherry'
indices = [i for i, x in enumerate(my_list) if x == element]
print(f"Indices of element {element}: {indices}")
四、使用 filter
函数
filter
函数用于过滤序列,返回一个迭代器。它需要两个参数:一个函数和一个序列。函数用于判断元素是否满足条件,序列是要过滤的列表。
my_list = [10, 20, 30, 40, 50]
threshold = 25
filtered_elements = list(filter(lambda x: x > threshold, my_list))
print(f"Elements greater than {threshold}: {filtered_elements}")
五、结合 map
和 filter
函数
map
和 filter
函数结合使用,可以对列表中的元素进行变换和过滤。
my_list = [1, 2, 3, 4, 5]
result = list(map(lambda x: x2, filter(lambda x: x % 2 == 0, my_list)))
print(f"Squares of even numbers: {result}")
六、使用 numpy
库查找元素
如果处理的是数值列表,numpy
库提供了更高效的查找方法。
import numpy as np
my_list = [10, 20, 30, 40, 50]
np_list = np.array(my_list)
threshold = 25
elements = np_list[np_list > threshold]
print(f"Elements greater than {threshold}: {elements}")
七、使用 pandas
库查找元素
对于数据分析任务,pandas
库提供了非常强大的查找功能。
import pandas as pd
my_list = [10, 20, 30, 40, 50]
series = pd.Series(my_list)
threshold = 25
elements = series[series > threshold]
print(f"Elements greater than {threshold}: {elements}")
八、查找嵌套列表中的元素
查找嵌套列表中的元素稍微复杂一些,可以使用递归的方法。
def find_in_nested_list(nested_list, element):
for i, item in enumerate(nested_list):
if isinstance(item, list):
result = find_in_nested_list(item, element)
if result is not None:
return [i] + result
elif item == element:
return [i]
return None
nested_list = [1, [2, 3], [4, [5, 6]], 7]
element = 6
index_path = find_in_nested_list(nested_list, element)
if index_path is not None:
print(f"Element {element} found at index path {index_path}")
else:
print(f"Element {element} not found in the nested list")
九、查找对象列表中的元素
在实际应用中,列表中的元素可能是对象,可以通过对象的属性进行查找。
class Fruit:
def __init__(self, name, color):
self.name = name
self.color = color
my_list = [Fruit('apple', 'red'), Fruit('banana', 'yellow'), Fruit('cherry', 'red')]
element_name = 'cherry'
element_color = 'red'
found_elements = [f for f in my_list if f.name == element_name and f.color == element_color]
print(f"Found elements: {[f.name for f in found_elements]}")
十、查找复杂条件的元素
有时需要查找符合复杂条件的元素,可以结合多个条件进行查找。
my_list = [{'name': 'apple', 'color': 'red'}, {'name': 'banana', 'color': 'yellow'}, {'name': 'cherry', 'color': 'red'}]
element_name = 'cherry'
element_color = 'red'
found_elements = [item for item in my_list if item['name'] == element_name and item['color'] == element_color]
print(f"Found elements: {found_elements}")
十一、总结
在Python中,找出列表里的特定元素的方法有很多,选择哪种方法取决于具体的需求和场景。常用的方法包括:使用列表方法(如 index()
和 count()
)、使用条件表达式、使用列表推导式、使用 filter
函数、结合 map
和 filter
函数、使用 numpy
库、使用 pandas
库、查找嵌套列表中的元素、查找对象列表中的元素、查找复杂条件的元素。根据不同的需求,可以灵活地选择和组合这些方法来实现高效的查找操作。
相关问答FAQs:
如何在Python中检查列表是否包含特定元素?
在Python中,可以使用in
关键字来检查一个元素是否存在于列表中。例如,如果有一个名为my_list
的列表,您可以通过if element in my_list:
来判断element
是否在my_list
中。如果存在,您可以执行相应的操作。
在Python中如何获取特定元素的索引?
如果您想找到列表中特定元素的索引,可以使用list.index()
方法。比如,index_value = my_list.index(element)
将返回element
在my_list
中的索引。如果该元素不在列表中,将引发ValueError
异常,因此建议使用in
关键字先检查元素是否存在。
Python中如何找到所有匹配特定条件的元素?
要找出列表中所有满足特定条件的元素,可以使用列表推导式或filter()
函数。例如,若您希望找到所有大于10的数字,可以使用filtered_list = [x for x in my_list if x > 10]
或filtered_list = list(filter(lambda x: x > 10, my_list))
。这将返回一个新列表,其中包含所有符合条件的元素。
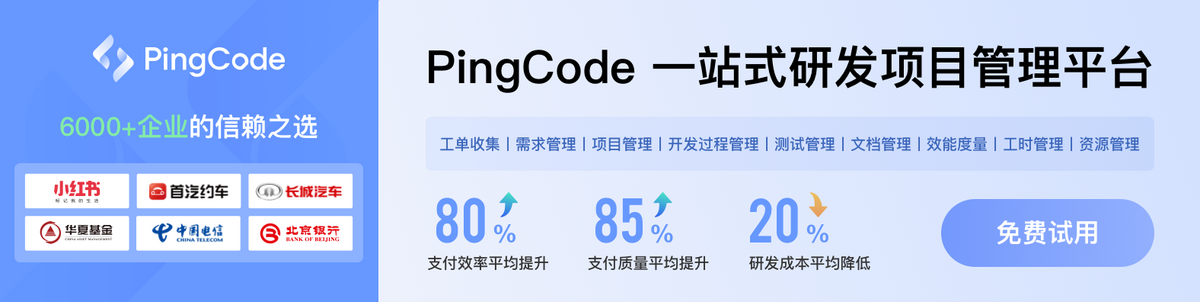