在Python中,可以通过使用base64模块将图片进行Base64加密、首先读取图片内容,然后将其编码为Base64字符串、使用base64.b64encode函数进行编码。
详细描述:
Base64是一种基于64个可打印字符来表示二进制数据的编码方式。在Python中,使用base64模块可以轻松地将图片进行Base64编码。首先,我们需要读取图片的二进制数据,然后使用base64.b64encode函数对读取的数据进行编码,并将编码后的数据转换为字符串。
一、读取图片并进行Base64编码
在读取图片并进行Base64编码之前,我们需要确保已经安装了所需的Python模块。以下是实现该功能的代码示例:
import base64
def image_to_base64(image_path):
with open(image_path, "rb") as image_file:
encoded_string = base64.b64encode(image_file.read())
return encoded_string.decode('utf-8')
image_path = 'path_to_your_image.jpg'
encoded_image = image_to_base64(image_path)
print(encoded_image)
在上述代码中,我们定义了一个名为image_to_base64
的函数,该函数接受图片的路径作为参数。首先,使用open
函数以二进制模式读取图片文件,接着使用base64.b64encode
函数对读取的二进制数据进行编码,最后将编码后的数据转换为字符串并返回。
二、解码Base64字符串并保存图片
有时我们还需要将Base64编码的字符串解码回图片。以下是实现该功能的代码示例:
def base64_to_image(encoded_string, output_path):
decoded_image = base64.b64decode(encoded_string)
with open(output_path, "wb") as image_file:
image_file.write(decoded_image)
output_path = 'decoded_image.jpg'
base64_to_image(encoded_image, output_path)
在上述代码中,我们定义了一个名为base64_to_image
的函数,该函数接受Base64编码的字符串和输出图片的路径作为参数。首先,使用base64.b64decode
函数对编码的字符串进行解码,接着使用open
函数以二进制写入模式将解码后的数据保存为图片文件。
三、处理大图片文件
对于较大的图片文件,直接读取整个文件可能会导致内存占用过多。我们可以使用分块读取的方式来处理大图片文件。以下是实现该功能的代码示例:
def image_to_base64_chunked(image_path, chunk_size=1024):
encoded_chunks = []
with open(image_path, "rb") as image_file:
while chunk = image_file.read(chunk_size):
encoded_chunks.append(base64.b64encode(chunk).decode('utf-8'))
return ''.join(encoded_chunks)
image_path = 'large_image.jpg'
encoded_image_chunked = image_to_base64_chunked(image_path)
print(encoded_image_chunked)
在上述代码中,我们定义了一个名为image_to_base64_chunked
的函数,该函数接受图片的路径和分块大小作为参数。首先,使用open
函数以二进制模式读取图片文件,并使用while
循环分块读取文件数据。每次读取的数据块都会被编码为Base64字符串,并添加到encoded_chunks
列表中。最后,将所有编码的字符串块拼接成一个完整的Base64编码字符串。
四、将Base64编码结果嵌入HTML中
将图片转换为Base64编码字符串后,我们可以将其嵌入到HTML中,以便在网页上显示图片。以下是实现该功能的代码示例:
def image_to_base64_for_html(image_path):
encoded_string = image_to_base64(image_path)
return f'data:image/jpeg;base64,{encoded_string}'
image_path = 'path_to_your_image.jpg'
encoded_image_for_html = image_to_base64_for_html(image_path)
html_content = f'<img src="{encoded_image_for_html}" alt="Base64 Image"/>'
print(html_content)
在上述代码中,我们定义了一个名为image_to_base64_for_html
的函数,该函数接受图片的路径作为参数。首先,使用image_to_base64
函数将图片编码为Base64字符串,接着将编码后的字符串嵌入到HTML的img
标签中。
五、使用PIL库处理图片
在处理图片时,我们还可以使用PIL(Pillow)库对图片进行预处理,例如调整图片大小、裁剪等。以下是实现该功能的代码示例:
from PIL import Image
import io
def image_to_base64_with_pil(image_path):
with Image.open(image_path) as image:
buffer = io.BytesIO()
image.save(buffer, format="JPEG")
encoded_string = base64.b64encode(buffer.getvalue())
return encoded_string.decode('utf-8')
image_path = 'path_to_your_image.jpg'
encoded_image_with_pil = image_to_base64_with_pil(image_path)
print(encoded_image_with_pil)
在上述代码中,我们定义了一个名为image_to_base64_with_pil
的函数,该函数接受图片的路径作为参数。首先,使用Image.open
函数打开图片,并将其保存到内存中的缓冲区。接着,使用base64.b64encode
函数对缓冲区中的数据进行编码,并将编码后的数据转换为字符串并返回。
六、处理不同格式的图片
在实际应用中,我们可能需要处理不同格式的图片,例如PNG、GIF等。我们可以根据图片的格式动态设置MIME类型,并将其嵌入到HTML中。以下是实现该功能的代码示例:
def image_to_base64_with_format(image_path):
image_format = image_path.split('.')[-1].lower()
mime_type = f'image/{image_format}'
encoded_string = image_to_base64(image_path)
return f'data:{mime_type};base64,{encoded_string}'
image_path = 'path_to_your_image.png'
encoded_image_with_format = image_to_base64_with_format(image_path)
html_content = f'<img src="{encoded_image_with_format}" alt="Base64 Image"/>'
print(html_content)
在上述代码中,我们定义了一个名为image_to_base64_with_format
的函数,该函数接受图片的路径作为参数。首先,提取图片的格式,并根据格式动态设置MIME类型。接着,使用image_to_base64
函数将图片编码为Base64字符串,并将编码后的字符串嵌入到HTML的img
标签中。
总结
通过上述示例,我们介绍了如何在Python中将图片进行Base64编码,并将编码结果嵌入到HTML中。我们还介绍了如何处理大图片文件、使用PIL库对图片进行预处理以及处理不同格式的图片。希望这些示例对您有所帮助。
相关问答FAQs:
如何将在线图片转换为Base64编码?
要将在线图片转换为Base64编码,可以使用Python的requests
库下载图片,再使用base64
库进行编码。具体步骤包括发送HTTP请求获取图片数据,读取这些数据,并将其转化为Base64格式。示例代码如下:
import requests
import base64
url = '图片的URL' # 替换为实际的图片链接
response = requests.get(url)
image_data = response.content
base64_encoded = base64.b64encode(image_data).decode('utf-8')
转换后的Base64编码有什么用途?
Base64编码常用于将二进制数据转换为文本格式,方便在网页中嵌入图片或在API中传输。它在数据传输中减少了二进制数据的复杂性,确保在不同系统间的兼容性。常见应用场景包括电子邮件附件、数据嵌入HTML或CSS中等。
有哪些Python库可以帮助处理Base64编码?
Python自带的base64
库是处理Base64编码的标准选择。此外,Pillow
库可以用来处理图像文件,方便与Base64编码结合使用。对于需要更复杂图像处理的场景,OpenCV
也是一个不错的选择,可以与Base64结合使用来实现图像的读取和编码。
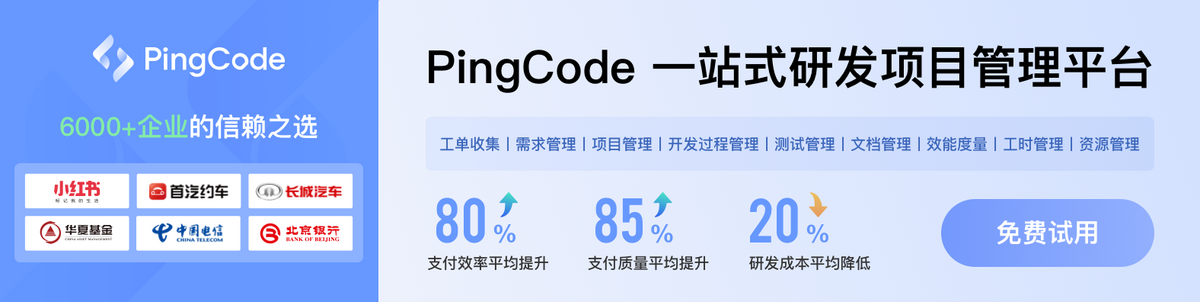