在Python中实现步长为2的卷积有几种方法,其中最常用的一种是使用深度学习框架,比如TensorFlow或PyTorch,这些库提供了高度优化和易于使用的卷积操作。使用深度学习框架、手动实现卷积操作、优化代码性能,在这篇文章中,我们将详细介绍如何使用Python实现步长为2的卷积操作,并探讨每种方法的细节。
一、使用深度学习框架
1. TensorFlow
TensorFlow是一个广泛使用的深度学习框架,它提供了许多方便的函数来实现卷积操作。以下是一个示例代码,演示如何使用TensorFlow实现步长为2的卷积:
import tensorflow as tf
创建输入张量(例如,一个4D张量)
input_tensor = tf.random.normal([1, 28, 28, 3]) # (batch_size, height, width, channels)
创建卷积核
filters = tf.random.normal([5, 5, 3, 32]) # (filter_height, filter_width, in_channels, out_channels)
执行卷积操作,步长为2
conv_layer = tf.nn.conv2d(input_tensor, filters, strides=[1, 2, 2, 1], padding='SAME')
print(conv_layer.shape)
在这个代码示例中,我们创建了一个随机输入张量和一个随机卷积核,然后使用tf.nn.conv2d
函数执行卷积操作。我们通过设置strides
参数的值为[1, 2, 2, 1]
来指定步长为2。
2. PyTorch
PyTorch是另一个流行的深度学习框架,它也提供了类似的卷积操作。以下是一个示例代码,演示如何使用PyTorch实现步长为2的卷积:
import torch
import torch.nn as nn
创建输入张量(例如,一个4D张量)
input_tensor = torch.randn(1, 3, 28, 28) # (batch_size, channels, height, width)
创建卷积层
conv_layer = nn.Conv2d(in_channels=3, out_channels=32, kernel_size=5, stride=2, padding=2)
执行卷积操作
output_tensor = conv_layer(input_tensor)
print(output_tensor.shape)
在这个代码示例中,我们创建了一个随机输入张量和一个卷积层,然后通过调用卷积层来执行卷积操作。我们通过设置stride
参数的值为2来指定步长为2。
二、手动实现卷积操作
除了使用深度学习框架之外,我们还可以手动实现卷积操作。这种方法可以帮助我们更深入地理解卷积操作的工作原理。以下是一个示例代码,演示如何手动实现步长为2的卷积:
import numpy as np
def convolve2d(input, kernel, stride=2, padding=0):
input_padded = np.pad(input, ((padding, padding), (padding, padding)), mode='constant')
output_shape = ((input_padded.shape[0] - kernel.shape[0]) // stride + 1,
(input_padded.shape[1] - kernel.shape[1]) // stride + 1)
output = np.zeros(output_shape)
for y in range(output_shape[0]):
for x in range(output_shape[1]):
output[y, x] = np.sum(input_padded[y*stride:y*stride+kernel.shape[0], x*stride:x*stride+kernel.shape[1]] * kernel)
return output
创建输入数组和卷积核
input_array = np.random.rand(28, 28)
kernel = np.random.rand(5, 5)
执行卷积操作
output_array = convolve2d(input_array, kernel, stride=2, padding=2)
print(output_array.shape)
在这个代码示例中,我们手动实现了一个2D卷积函数convolve2d
,并使用numpy
库来进行数组操作。我们通过设置stride
参数的值为2来指定步长为2。
三、优化代码性能
手动实现卷积操作虽然可以帮助我们理解其工作原理,但在实际应用中通常不如使用深度学习框架高效。为了提高代码性能,我们可以使用一些优化技巧,比如:
1. 使用NumPy进行矢量化操作
NumPy提供了高效的矢量化操作,可以显著提高代码性能。以下是一个示例代码,演示如何使用NumPy进行矢量化操作:
import numpy as np
def convolve2d_optimized(input, kernel, stride=2, padding=0):
input_padded = np.pad(input, ((padding, padding), (padding, padding)), mode='constant')
output_shape = ((input_padded.shape[0] - kernel.shape[0]) // stride + 1,
(input_padded.shape[1] - kernel.shape[1]) // stride + 1)
output = np.zeros(output_shape)
kernel_flipped = np.flipud(np.fliplr(kernel))
for y in range(0, output_shape[0] * stride, stride):
for x in range(0, output_shape[1] * stride, stride):
output[y//stride, x//stride] = np.sum(input_padded[y:y+kernel.shape[0], x:x+kernel.shape[1]] * kernel_flipped)
return output
创建输入数组和卷积核
input_array = np.random.rand(28, 28)
kernel = np.random.rand(5, 5)
执行卷积操作
output_array = convolve2d_optimized(input_array, kernel, stride=2, padding=2)
print(output_array.shape)
在这个代码示例中,我们通过翻转卷积核来简化卷积操作,并使用NumPy进行矢量化操作,从而提高了代码性能。
2. 使用并行计算
为了进一步提高代码性能,我们还可以使用并行计算。以下是一个示例代码,演示如何使用并行计算来优化卷积操作:
import numpy as np
from joblib import Parallel, delayed
def convolve2d_parallel(input, kernel, stride=2, padding=0, n_jobs=-1):
input_padded = np.pad(input, ((padding, padding), (padding, padding)), mode='constant')
output_shape = ((input_padded.shape[0] - kernel.shape[0]) // stride + 1,
(input_padded.shape[1] - kernel.shape[1]) // stride + 1)
output = np.zeros(output_shape)
kernel_flipped = np.flipud(np.fliplr(kernel))
def convolve_single_pixel(y, x):
return np.sum(input_padded[y:y+kernel.shape[0], x:x+kernel.shape[1]] * kernel_flipped)
results = Parallel(n_jobs=n_jobs)(delayed(convolve_single_pixel)(y, x)
for y in range(0, output_shape[0] * stride, stride)
for x in range(0, output_shape[1] * stride, stride))
for idx, value in enumerate(results):
y, x = divmod(idx, output_shape[1])
output[y, x] = value
return output
创建输入数组和卷积核
input_array = np.random.rand(28, 28)
kernel = np.random.rand(5, 5)
执行卷积操作
output_array = convolve2d_parallel(input_array, kernel, stride=2, padding=2)
print(output_array.shape)
在这个代码示例中,我们使用joblib
库来实现并行计算,从而进一步提高了代码性能。
总结
在这篇文章中,我们详细介绍了如何使用Python实现步长为2的卷积操作,包括使用深度学习框架、手动实现卷积操作和优化代码性能。通过这些方法,我们可以高效地实现卷积操作,并进一步提高代码性能。无论是使用现成的深度学习框架还是手动实现卷积操作,都可以帮助我们更好地理解卷积操作的工作原理,并在实际应用中灵活运用。
相关问答FAQs:
卷积操作的步长设置是什么?
卷积操作的步长是指在进行卷积时,卷积核在输入数据上滑动的步幅。步长为2意味着卷积核每次移动2个单位,这样可以减少输出特征图的尺寸,从而提取更具代表性的特征。在实现过程中,适当的步长设置有助于控制计算量和提高模型的效率。
在使用Python进行卷积时,如何选择合适的卷积库?
在Python中,常用的卷积库包括NumPy、TensorFlow和PyTorch等。NumPy适合于基础的数值计算和自定义卷积操作,而TensorFlow和PyTorch则提供了更高效的深度学习框架,支持自动求导和GPU加速。如果目标是构建深度学习模型,建议选择TensorFlow或PyTorch,这样可以利用它们丰富的功能和社区支持。
如何在代码中实现步长为2的卷积操作示例?
实现步长为2的卷积操作可以使用以下代码示例(以NumPy为例):
import numpy as np
def convolution2d(input, kernel, stride=2):
kernel_height, kernel_width = kernel.shape
input_height, input_width = input.shape
output_height = (input_height - kernel_height) // stride + 1
output_width = (input_width - kernel_width) // stride + 1
output = np.zeros((output_height, output_width))
for y in range(0, input_height - kernel_height + 1, stride):
for x in range(0, input_width - kernel_width + 1, stride):
output[y // stride, x // stride] = np.sum(input[y:y + kernel_height, x:x + kernel_width] * kernel)
return output
# 示例输入和卷积核
input_data = np.array([[1, 2, 3, 0],
[0, 1, 2, 3],
[1, 0, 0, 1],
[2, 3, 1, 0]])
kernel = np.array([[1, 0],
[0, 1]])
result = convolution2d(input_data, kernel)
print(result)
该代码展示了如何使用自定义的卷积函数实现步长为2的卷积操作。通过调整步长参数,可以灵活控制卷积的移动策略。
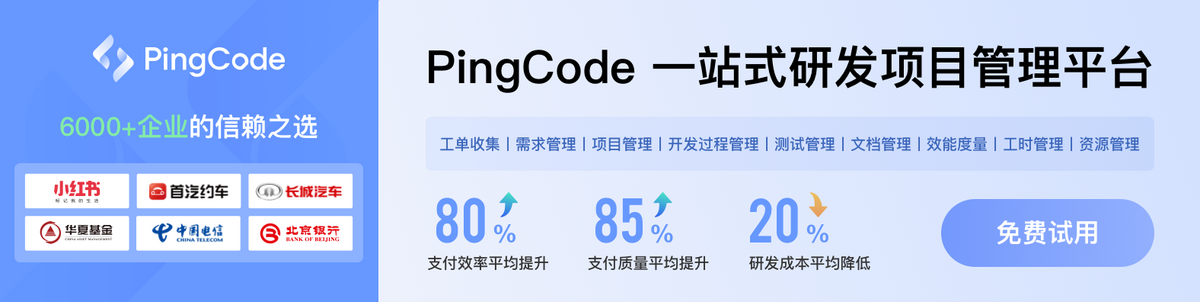