如何用Python处理n个数据库
要用Python处理多个数据库,可以使用以下几种方法:使用数据库连接池、利用ORM工具、编写自定义的数据库管理脚本。在这篇文章中,我们将详细探讨这几种方法,并提供示例代码以供参考。使用数据库连接池、利用ORM工具、编写自定义的数据库管理脚本,其中,我们将详细描述如何利用ORM工具进行数据库操作。
一、使用数据库连接池
数据库连接池是一种用于管理数据库连接的技术。它可以有效地减少连接和断开数据库的开销,提高应用程序的性能和可靠性。常见的数据库连接池库包括SQLAlchemy
和DBUtils
。
1、SQLAlchemy连接池
SQLAlchemy
是Python中一个流行的SQL工具包和ORM库。它提供了一个功能强大的连接池,可以轻松管理多个数据库连接。
from sqlalchemy import create_engine
创建数据库引擎
engine1 = create_engine('mysql+pymysql://user:password@host1/dbname1')
engine2 = create_engine('mysql+pymysql://user:password@host2/dbname2')
执行数据库操作
with engine1.connect() as connection1:
result1 = connection1.execute("SELECT * FROM table1")
for row in result1:
print(row)
with engine2.connect() as connection2:
result2 = connection2.execute("SELECT * FROM table2")
for row in result2:
print(row)
2、DBUtils连接池
DBUtils
是另一个流行的数据库连接池库,它支持多种数据库类型。下面是一个使用DBUtils
的示例:
from DBUtils.PooledDB import PooledDB
import pymysql
创建数据库连接池
pool1 = PooledDB(pymysql, 5, host='host1', user='user', passwd='password', db='dbname1')
pool2 = PooledDB(pymysql, 5, host='host2', user='user', passwd='password', db='dbname2')
获取连接并执行数据库操作
connection1 = pool1.connection()
cursor1 = connection1.cursor()
cursor1.execute("SELECT * FROM table1")
for row in cursor1.fetchall():
print(row)
connection1.close()
connection2 = pool2.connection()
cursor2 = connection2.cursor()
cursor2.execute("SELECT * FROM table2")
for row in cursor2.fetchall():
print(row)
connection2.close()
二、利用ORM工具
ORM(对象关系映射)工具可以将数据库表映射为Python类,使得我们可以使用面向对象的方式操作数据库。常用的ORM工具有SQLAlchemy
和Django ORM
。
1、SQLAlchemy ORM
SQLAlchemy
不仅是一个连接池库,还是一个功能强大的ORM工具。下面是一个使用SQLAlchemy ORM
的示例:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Base = declarative_base()
定义映射类
class Table1(Base):
__tablename__ = 'table1'
id = Column(Integer, primary_key=True)
name = Column(String)
class Table2(Base):
__tablename__ = 'table2'
id = Column(Integer, primary_key=True)
name = Column(String)
创建数据库引擎
engine1 = create_engine('mysql+pymysql://user:password@host1/dbname1')
engine2 = create_engine('mysql+pymysql://user:password@host2/dbname2')
创建会话
Session1 = sessionmaker(bind=engine1)
session1 = Session1()
Session2 = sessionmaker(bind=engine2)
session2 = Session2()
查询数据
results1 = session1.query(Table1).all()
for row in results1:
print(row.name)
results2 = session2.query(Table2).all()
for row in results2:
print(row.name)
2、Django ORM
Django ORM
是Django
框架自带的ORM工具。如果你正在使用Django
开发一个Web应用,可以使用Django ORM
来管理多个数据库。
在Django
的settings.py
文件中配置多个数据库:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'dbname1',
'USER': 'user',
'PASSWORD': 'password',
'HOST': 'host1',
'PORT': '3306',
},
'db2': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'dbname2',
'USER': 'user',
'PASSWORD': 'password',
'HOST': 'host2',
'PORT': '3306',
},
}
在模型中使用不同的数据库:
from django.db import models
class Table1(models.Model):
name = models.CharField(max_length=100)
class Meta:
db_table = 'table1'
app_label = 'default'
class Table2(models.Model):
name = models.CharField(max_length=100)
class Meta:
db_table = 'table2'
app_label = 'db2'
查询数据:
from myapp.models import Table1, Table2
results1 = Table1.objects.using('default').all()
for row in results1:
print(row.name)
results2 = Table2.objects.using('db2').all()
for row in results2:
print(row.name)
三、编写自定义的数据库管理脚本
如果你不想使用第三方库,可以编写自定义的数据库管理脚本来处理多个数据库。这种方法可以灵活地满足你的需求,但需要更多的工作量。
1、使用PyMySQL连接MySQL数据库
PyMySQL
是一个纯Python实现的MySQL客户端库。下面是一个使用PyMySQL
连接多个数据库的示例:
import pymysql
连接到第一个数据库
connection1 = pymysql.connect(host='host1', user='user', password='password', db='dbname1')
cursor1 = connection1.cursor()
cursor1.execute("SELECT * FROM table1")
for row in cursor1.fetchall():
print(row)
connection1.close()
连接到第二个数据库
connection2 = pymysql.connect(host='host2', user='user', password='password', db='dbname2')
cursor2 = connection2.cursor()
cursor2.execute("SELECT * FROM table2")
for row in cursor2.fetchall():
print(row)
connection2.close()
2、使用psycopg2连接PostgreSQL数据库
psycopg2
是一个用于连接PostgreSQL数据库的Python库。下面是一个使用psycopg2
连接多个数据库的示例:
import psycopg2
连接到第一个数据库
connection1 = psycopg2.connect(host='host1', user='user', password='password', dbname='dbname1')
cursor1 = connection1.cursor()
cursor1.execute("SELECT * FROM table1")
for row in cursor1.fetchall():
print(row)
connection1.close()
连接到第二个数据库
connection2 = psycopg2.connect(host='host2', user='user', password='password', dbname='dbname2')
cursor2 = connection2.cursor()
cursor2.execute("SELECT * FROM table2")
for row in cursor2.fetchall():
print(row)
connection2.close()
四、总结
在这篇文章中,我们探讨了如何用Python处理多个数据库。我们介绍了使用数据库连接池、利用ORM工具、编写自定义的数据库管理脚本等方法。使用数据库连接池可以提高应用程序的性能和可靠性,利用ORM工具可以方便地进行面向对象的数据库操作,而编写自定义的数据库管理脚本则可以灵活地满足特定需求。希望这篇文章对你有所帮助,能够让你更好地处理多个数据库。
相关问答FAQs:
如何选择合适的Python库来处理多个数据库?
在处理多个数据库时,选择合适的Python库非常关键。常用的库包括SQLAlchemy、Pandas和Django ORM等。SQLAlchemy提供了强大的数据库抽象和操作功能,适合复杂的应用场景;Pandas则在数据分析方面表现优异,适合从不同数据库中提取数据进行分析;Django ORM则适合于基于Django框架的应用,能够简化数据库操作。根据项目需求选择合适的库,可以提高开发效率。
如何在Python中连接和操作不同类型的数据库?
要在Python中连接不同类型的数据库,通常需要使用相应的数据库驱动程序。例如,MySQL可以使用mysql-connector-python,PostgreSQL则可以使用psycopg2,SQLite则内置在Python中。连接时,需要提供数据库的地址、用户名和密码等信息。操作数据库时,可以使用SQL语句或ORM方法进行增、删、改、查等操作。确保对每种数据库的特定操作有清晰的了解,以避免因数据库特性差异导致的问题。
在处理多个数据库时,如何确保数据的一致性和完整性?
确保数据一致性和完整性是处理多个数据库时的重要考量。可以采用事务管理来保证在多个数据库操作中的原子性,即要么全部成功,要么全部失败。此外,定期进行数据备份和使用数据验证规则可以帮助维护数据质量。使用数据同步工具或中间件也能有效实现不同数据库之间的数据一致性,尤其是在分布式系统中。务必对数据流向和数据依赖关系进行监控,以便及时发现和解决潜在问题。
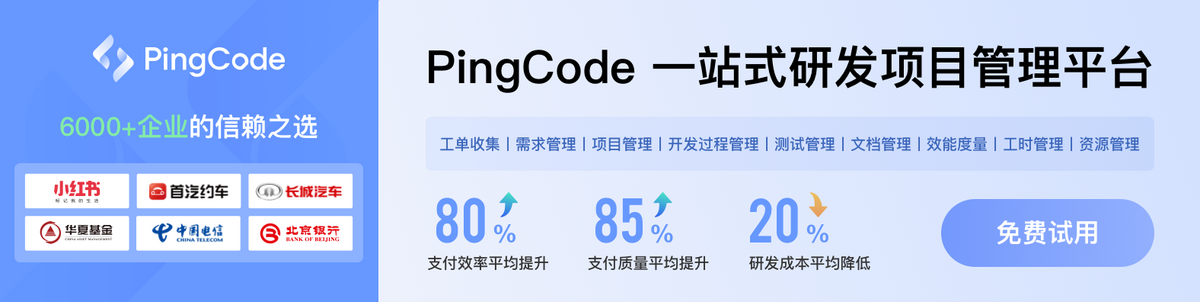