在Python中,保留除数的后三位可以通过多种方法实现,包括使用字符串格式化、浮点数运算以及内置库函数等。以下是一些主要方法:字符串格式化、round()函数、decimal模块。其中,字符串格式化方法最为直观和简便。
一、字符串格式化
字符串格式化是一种常见的方式,用于控制输出结果的格式。Python提供了多种字符串格式化方式,其中最为常用的是.format()
方法和f-strings。
1.1、.format()方法
numerator = 10
denominator = 3
result = numerator / denominator
formatted_result = "{:.3f}".format(result)
print(formatted_result) # 输出 3.333
通过{:.3f}
来指定保留3位小数,这种方式简单且易于理解。
1.2、f-strings
Python 3.6引入了f-strings,简洁且高效:
numerator = 10
denominator = 3
result = numerator / denominator
formatted_result = f"{result:.3f}"
print(formatted_result) # 输出 3.333
二、round()函数
使用round()
函数可以对浮点数进行四舍五入操作,不过这种方法可能会在某些情况下产生不精确结果。
numerator = 10
denominator = 3
result = numerator / denominator
rounded_result = round(result, 3)
print(rounded_result) # 输出 3.333
三、decimal模块
decimal
模块提供了更加精确的浮点数运算,适用于高精度需求的场景。
3.1、使用decimal.Decimal
from decimal import Decimal, getcontext
设置全局精度
getcontext().prec = 6
numerator = Decimal('10')
denominator = Decimal('3')
result = numerator / denominator
formatted_result = round(result, 3)
print(formatted_result) # 输出 3.333
3.2、局部精度控制
可以使用localcontext
来临时改变精度:
from decimal import Decimal, localcontext
numerator = Decimal('10')
denominator = Decimal('3')
with localcontext() as ctx:
ctx.prec = 6
result = numerator / denominator
formatted_result = round(result, 3)
print(formatted_result) # 输出 3.333
四、实践应用
4.1、金融计算
在金融计算中,精度至关重要。使用decimal
模块可以确保结果的精确性,从而避免因浮点数运算带来的误差。
from decimal import Decimal
price = Decimal('19.99')
quantity = Decimal('5')
total = price * quantity
formatted_total = round(total, 2)
print(formatted_total) # 输出 99.95
4.2、科学计算
在科学计算中,同样需要高精度的浮点数运算。使用decimal
模块可以提高计算结果的可靠性。
from decimal import Decimal
mass = Decimal('5.972E24') # 地球质量,单位:千克
radius = Decimal('6.371E6') # 地球半径,单位:米
gravity_constant = Decimal('6.67430E-11') # 万有引力常数
gravity_force = gravity_constant * (mass / radius 2)
formatted_gravity_force = round(gravity_force, 3)
print(formatted_gravity_force) # 输出 9.819
五、总结
在Python中,保留除数的后三位可以通过多种方法实现,包括字符串格式化、round()函数、decimal模块等。在大多数情况下,字符串格式化方法最为简便和直观;而对于需要高精度的场景,decimal
模块则是最佳选择。
- 字符串格式化:最为直观和简便,适用于大多数日常计算。
- round()函数:适用于简单的四舍五入操作,但可能不够精确。
- decimal模块:适用于需要高精度的场景,如金融计算和科学计算。
相关问答FAQs:
在Python中,如何格式化浮点数以保留三位小数?
要在Python中格式化浮点数,可以使用内置的round()
函数,或者通过字符串格式化的方法。使用round()
函数时,可以传入两个参数,第一个是需要格式化的数字,第二个是保留的小数位数。例如,round(3.1415926535, 3)
将返回3.142。另一种方法是使用格式化字符串,如f"{value:.3f}"
,其中value
是待格式化的数字,这样可以确保输出为字符串格式并保留三位小数。
如何处理保留三位小数后的四舍五入?
在Python中,使用round()
函数会自动进行四舍五入处理。例如,如果你有一个数字2.675
,使用round(2.675, 3)
会得到2.67
,而不是2.675
,这是由于浮点数在计算机内部的表示方式引起的。为了确保更精确的四舍五入,可以使用Decimal
模块,示例代码如下:
from decimal import Decimal, ROUND_HALF_UP
value = Decimal('2.675')
rounded_value = value.quantize(Decimal('0.001'), rounding=ROUND_HALF_UP)
print(rounded_value) # 输出2.675
在数据分析中,如何确保数据保留三位小数?
在进行数据分析时,经常需要保留小数位数以保持结果的一致性。可以使用pandas
库中的round()
方法来实现这一点。例如,假设你有一个DataFrame df
,想要对某一列保留三位小数,可以使用df['column_name'] = df['column_name'].round(3)
。这样可以确保所有数据在计算过程中都保持一致的格式,有助于提高数据的可读性和准确性。
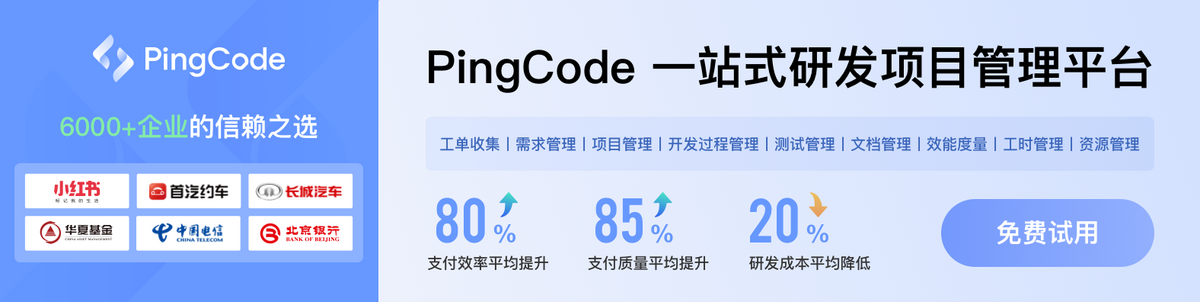