在Python中,去重字符串的几种方法包括使用集合、使用字典、使用列表解析、使用OrderedDict、使用set()和sorted()函数。 在这篇文章中,我们将详细讨论每种方法,并给出实际的代码示例。具体来说,使用集合(set)是一种非常有效的方法,因为集合本身就是无序且无重复元素的结构。我们可以通过将字符串转换为集合,然后再将其转换回字符串来实现去重。
一、使用集合(Set)去重
集合是一种无序且无重复元素的集合。通过将字符串转换为集合,我们可以轻松地去除重复的字符。
def remove_duplicates(input_string):
return ''.join(set(input_string))
input_string = "aabbcc"
print(remove_duplicates(input_string)) # 输出: "abc"
优点:这种方法非常简洁,适用于去除字符串中重复的字符且不关心字符的顺序。
缺点:集合是无序的,因此这种方法无法保留原字符串中的字符顺序。
二、使用字典(Dict)去重
字典在Python 3.7及以后的版本中是有序的,因此我们可以使用字典来去重并保留原字符串的顺序。
def remove_duplicates(input_string):
return ''.join(dict.fromkeys(input_string))
input_string = "aabbcc"
print(remove_duplicates(input_string)) # 输出: "abc"
优点:可以保留字符的顺序。
缺点:代码稍微复杂一些,但性能通常是可接受的。
三、使用列表解析去重
列表解析是一种非常Pythonic的方式,可以在一行代码内完成去重操作。
def remove_duplicates(input_string):
seen = set()
return ''.join([char for char in input_string if not (char in seen or seen.add(char))])
input_string = "aabbcc"
print(remove_duplicates(input_string)) # 输出: "abc"
优点:清晰且Pythonic,可以保留字符的顺序。
缺点:这种方法可能对新手来说不太直观。
四、使用OrderedDict去重
在Python的collections模块中,有一个OrderedDict类,它可以用来保持字典的插入顺序。
from collections import OrderedDict
def remove_duplicates(input_string):
return ''.join(OrderedDict.fromkeys(input_string))
input_string = "aabbcc"
print(remove_duplicates(input_string)) # 输出: "abc"
优点:可以保留字符的顺序,非常直观。
缺点:需要导入collections模块,稍微复杂一些。
五、使用set()和sorted()函数去重
如果我们希望去重并将字符按字母顺序排序,可以使用set()和sorted()函数。
def remove_duplicates(input_string):
return ''.join(sorted(set(input_string)))
input_string = "aabbcc"
print(remove_duplicates(input_string)) # 输出: "abc"
优点:去重并排序,非常直观。
缺点:无法保留原字符串的字符顺序。
总结
去重字符串在Python中有多种方法可以实现,每种方法都有其独特的优点和缺点。通过集合(set)可以快速去重但无法保留顺序,使用字典(Dict)和OrderedDict可以去重且保留顺序,列表解析提供了一种Pythonic的解决方案,而set()和sorted()函数可以去重并排序。根据具体需求选择合适的方法,可以有效地实现字符串去重。
希望这篇文章能帮助你更好地理解和应用Python中的字符串去重技术。
相关问答FAQs:
如何在Python中去除字符串中的重复字符?
在Python中,可以使用集合(set)来去除字符串中的重复字符。首先将字符串转换为集合,这样会自动过滤掉重复的字符。接着,可以将集合转换回字符串。示例代码如下:
input_string = "hello"
unique_string = ''.join(set(input_string))
print(unique_string) # 输出可能是 "ehlo" 或 "lohe",顺序不固定
注意,集合是无序的,因此字符的顺序可能会改变。
是否可以保留字符串中字符的原始顺序?
确实可以保留字符的原始顺序,方法是使用列表推导式和一个辅助集合来跟踪已经添加的字符。例如:
input_string = "hello"
seen = set()
unique_string = ''.join([char for char in input_string if not (char in seen or seen.add(char))])
print(unique_string) # 输出 "helo"
这种方法确保了字符的顺序与原字符串一致。
在处理长字符串时,有没有性能优化的建议?
处理长字符串时,可以考虑使用collections.OrderedDict
。这个类可以在保持顺序的同时去重,适合较长字符串的处理。示例代码如下:
from collections import OrderedDict
input_string = "hello world"
unique_string = ''.join(OrderedDict.fromkeys(input_string))
print(unique_string) # 输出 "helo wrd"
使用OrderedDict
可以有效地去除重复字符,同时保留其最初顺序,适用于更复杂的字符串处理。
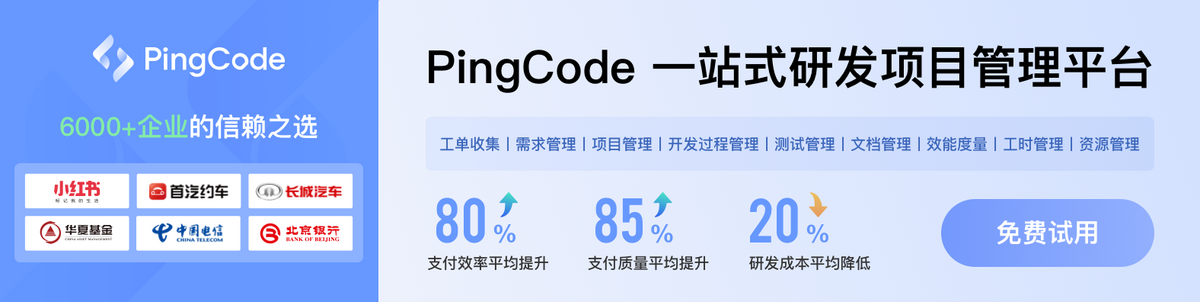