要把两列数用点连接在Python中,可以使用以下几种方法:字符串拼接、列表推导式、zip
函数和pandas
库。其中,pandas
库是最常用和最强大的方法之一,因为它提供了丰富的数据操作功能。下面,我们将详细介绍这几种方法,并提供相关代码示例。
一、字符串拼接
字符串拼接是最基本的方法之一。你可以遍历两个列表,然后将其元素用点连接起来。
# 两列数
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
结果列表
result = []
遍历两个列表
for a, b in zip(list1, list2):
result.append(f"{a}.{b}")
print(result)
输出: ['1.5', '2.6', '3.7', '4.8']
在这个例子中,我们使用了zip
函数来同时遍历两个列表,并使用f-string
来进行字符串拼接。
二、列表推导式
列表推导式是另一种简洁的方法来实现这一功能。
# 两列数
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
使用列表推导式
result = [f"{a}.{b}" for a, b in zip(list1, list2)]
print(result)
输出: ['1.5', '2.6', '3.7', '4.8']
这种方法相对于传统的for循环更加简洁和易读。
三、使用pandas
库
pandas
是Python中常用的数据分析库,它提供了丰富的数据操作功能。我们可以使用pandas
来实现两列数的点连接。
import pandas as pd
创建DataFrame
df = pd.DataFrame({
'col1': [1, 2, 3, 4],
'col2': [5, 6, 7, 8]
})
使用pandas的apply函数
df['combined'] = df.apply(lambda row: f"{row['col1']}.{row['col2']}", axis=1)
print(df['combined'].tolist())
输出: ['1.5', '2.6', '3.7', '4.8']
在这个例子中,我们使用pandas
的apply
函数来遍历DataFrame的每一行,并将两列数用点连接起来。
四、使用numpy
库
numpy
是另一个常用的数据处理库,可以用来进行数组操作。
import numpy as np
创建numpy数组
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
使用numpy的vectorize函数
vec_func = np.vectorize(lambda a, b: f"{a}.{b}")
result = vec_func(arr1, arr2)
print(result)
输出: ['1.5' '2.6' '3.7' '4.8']
在这个例子中,我们使用numpy
的vectorize
函数将两个数组的元素用点连接起来。
五、itertools
库
itertools
库提供了很多高效的迭代器工具。我们可以使用itertools.zip_longest
来处理不等长的列表。
import itertools
两列数
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7]
使用itertools.zip_longest
result = [f"{a}.{b}" for a, b in itertools.zip_longest(list1, list2, fillvalue=0)]
print(result)
输出: ['1.5', '2.6', '3.7', '4.0']
在这个例子中,我们使用itertools.zip_longest
来处理长度不等的列表,并用fillvalue
参数填充缺失值。
六、map
函数
map
函数也是一个高效的工具,可以用来对列表进行操作。
# 两列数
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
使用map函数
result = list(map(lambda a, b: f"{a}.{b}", list1, list2))
print(result)
输出: ['1.5', '2.6', '3.7', '4.8']
在这个例子中,我们使用map
函数将两个列表的元素用点连接起来。
七、处理大数据集的优化策略
在处理大数据集时,效率是一个重要的考量因素。以下是一些优化策略:
1. 使用pandas
的矢量化操作
pandas
的矢量化操作可以显著提高性能。
import pandas as pd
创建大DataFrame
df = pd.DataFrame({
'col1': range(1000000),
'col2': range(1000000, 2000000)
})
使用矢量化操作
df['combined'] = df['col1'].astype(str) + '.' + df['col2'].astype(str)
print(df['combined'].head())
输出: 0 0.1000000
1 1.1000001
2 2.1000002
3 3.1000003
4 4.1000004
Name: combined, dtype: object
2. 使用numpy
的矢量化操作
同样,numpy
也提供了矢量化操作。
import numpy as np
创建大numpy数组
arr1 = np.arange(1000000)
arr2 = np.arange(1000000, 2000000)
使用numpy的矢量化操作
result = np.char.add(np.char.add(arr1.astype(str), '.'), arr2.astype(str))
print(result[:5])
输出: ['0.1000000' '1.1000001' '2.1000002' '3.1000003' '4.1000004']
3. 多线程处理
对于极大规模的数据集,多线程处理可以显著提高效率。
import concurrent.futures
两列数
list1 = range(1000000)
list2 = range(1000000, 2000000)
def combine(a, b):
return f"{a}.{b}"
使用多线程处理
with concurrent.futures.ThreadPoolExecutor() as executor:
result = list(executor.map(combine, list1, list2))
print(result[:5])
输出: ['0.1000000', '1.1000001', '2.1000002', '3.1000003', '4.1000004']
结论
在Python中,将两列数用点连接起来的方法多种多样,可以根据具体需求选择合适的方法。对于小规模数据,字符串拼接、列表推导式和map
函数都是不错的选择;对于大规模数据,pandas
和numpy
的矢量化操作以及多线程处理则更为高效。通过了解和掌握这些方法,可以更灵活地处理不同规模和复杂度的数据任务。
相关问答FAQs:
如何在Python中将两列数字连接为点?
在Python中,可以使用matplotlib
库来实现将两列数字用点连接的功能。首先,确保安装了matplotlib
库。接着,您可以使用plt.plot()
函数将这两列数据以点的形式连接起来,示例代码如下:
import matplotlib.pyplot as plt
# 示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y, marker='o') # 使用圆点连接
plt.title('两列数的连接图')
plt.xlabel('X 轴')
plt.ylabel('Y 轴')
plt.show()
在Python中如何处理缺失值以连接两列数?
在处理数据时,缺失值可能会影响连接的结果。可以使用pandas
库中的dropna()
方法来清除缺失值,或者用fillna()
方法填充缺失值。这样可以确保在绘制图形时不会出现错误。以下是一个示例:
import pandas as pd
import matplotlib.pyplot as plt
# 创建数据框
data = {'x': [1, 2, None, 4, 5], 'y': [2, 3, 5, None, 11]}
df = pd.DataFrame(data)
# 清除缺失值
df = df.dropna()
plt.plot(df['x'], df['y'], marker='o')
plt.title('处理缺失值后的连接图')
plt.xlabel('X 轴')
plt.ylabel('Y 轴')
plt.show()
是否可以自定义连接的样式和颜色?
在Python中,matplotlib
提供了丰富的参数来定制图形的样式和颜色。您可以在plt.plot()
函数中使用color
、linestyle
和marker
等参数来改变线条颜色、样式和点的形状。例如:
plt.plot(x, y, marker='s', color='red', linestyle='--') # 使用方形点和红色虚线
plt.title('自定义样式的连接图')
plt.xlabel('X 轴')
plt.ylabel('Y 轴')
plt.show()
通过这些参数,您可以轻松创建出个性化的图形。
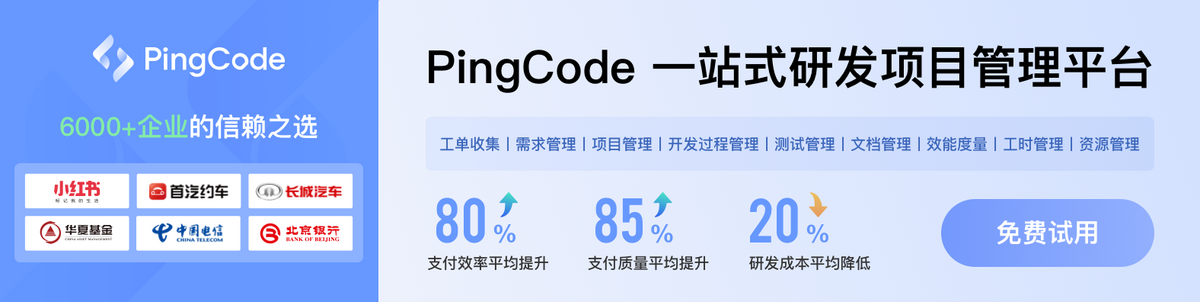