Python实现两个txt文档连接的方法包括:文件读取、字符串拼接、文件写入。本文将详细介绍如何使用Python实现两个txt文档的连接,并提供代码示例和实践建议。
一、文件读取
在实现两个txt文档的连接之前,首先需要将这两个文档的内容读取到内存中。Python提供了许多便捷的方法来读取文件内容,其中最常用的方法是使用内置的open
函数。
1. 使用open
函数读取文件
open
函数是Python中用于打开文件的函数,通过它可以读取文件的内容并进行处理。以下是一个简单的示例,展示了如何使用open
函数读取文件内容:
with open('file1.txt', 'r', encoding='utf-8') as file1:
content1 = file1.read()
with open('file2.txt', 'r', encoding='utf-8') as file2:
content2 = file2.read()
在这个示例中,我们使用with
语句来打开文件,这样可以确保文件在使用完毕后自动关闭,避免资源泄露。'r'
表示以只读模式打开文件,encoding='utf-8'
确保文件内容按UTF-8编码读取。
2. 错误处理
在读取文件时,可能会遇到文件不存在或读取权限不足等问题。为了提高代码的健壮性,可以使用异常处理来捕获这些错误:
try:
with open('file1.txt', 'r', encoding='utf-8') as file1:
content1 = file1.read()
except FileNotFoundError:
print("File1 not found.")
except IOError:
print("An error occurred while reading file1.")
try:
with open('file2.txt', 'r', encoding='utf-8') as file2:
content2 = file2.read()
except FileNotFoundError:
print("File2 not found.")
except IOError:
print("An error occurred while reading file2.")
通过这种方式,可以确保在文件读取过程中出现错误时,程序不会直接崩溃,而是输出相应的错误信息。
二、字符串拼接
在成功读取两个文件的内容后,下一步是将这两个字符串拼接在一起。Python提供了多种字符串拼接的方法,最常用的方法是使用加号(+
)操作符。
1. 基本拼接
以下是一个简单的示例,展示了如何拼接两个字符串:
combined_content = content1 + content2
这种方法非常直观,可以直接将两个字符串连接在一起。
2. 添加分隔符
在实际应用中,可能需要在拼接的字符串之间添加一些分隔符,例如换行符或特定的标记符。以下是一个示例,展示了如何在两个字符串之间添加换行符:
combined_content = content1 + '\n' + content2
通过这种方式,可以确保拼接后的内容更加清晰易读。
三、文件写入
在完成字符串拼接后,最后一步是将拼接后的内容写入新的文件中。Python同样提供了便捷的方法来进行文件写入操作。
1. 使用open
函数写入文件
以下是一个简单的示例,展示了如何使用open
函数将拼接后的内容写入新文件:
with open('combined_file.txt', 'w', encoding='utf-8') as combined_file:
combined_file.write(combined_content)
在这个示例中,我们使用'w'
模式打开文件,这表示以写入模式打开文件。如果文件不存在,则会创建一个新文件;如果文件已经存在,则会覆盖原有内容。
2. 错误处理
同样地,在文件写入过程中,也可能会遇到权限不足等问题。可以使用异常处理来捕获这些错误:
try:
with open('combined_file.txt', 'w', encoding='utf-8') as combined_file:
combined_file.write(combined_content)
except IOError:
print("An error occurred while writing to the file.")
通过这种方式,可以确保在文件写入过程中出现错误时,程序不会直接崩溃,而是输出相应的错误信息。
四、综合示例
以下是一个综合示例,展示了如何将上述步骤结合在一起,实现两个txt文档的连接:
try:
with open('file1.txt', 'r', encoding='utf-8') as file1:
content1 = file1.read()
except FileNotFoundError:
print("File1 not found.")
content1 = ''
except IOError:
print("An error occurred while reading file1.")
content1 = ''
try:
with open('file2.txt', 'r', encoding='utf-8') as file2:
content2 = file2.read()
except FileNotFoundError:
print("File2 not found.")
content2 = ''
except IOError:
print("An error occurred while reading file2.")
content2 = ''
combined_content = content1 + '\n' + content2
try:
with open('combined_file.txt', 'w', encoding='utf-8') as combined_file:
combined_file.write(combined_content)
except IOError:
print("An error occurred while writing to the file.")
这个综合示例展示了如何通过文件读取、字符串拼接和文件写入实现两个txt文档的连接,同时还包括了基本的错误处理逻辑。
五、实践建议
1. 文件路径管理
在实际项目中,通常需要处理多个文件,并且这些文件可能位于不同的目录下。为了简化文件路径的管理,可以使用os
模块中的path
功能。例如:
import os
file1_path = os.path.join('path', 'to', 'file1.txt')
file2_path = os.path.join('path', 'to', 'file2.txt')
combined_file_path = os.path.join('path', 'to', 'combined_file.txt')
通过这种方式,可以确保文件路径的拼接更加规范,减少路径错误的发生。
2. 大文件处理
对于大文件的处理,直接将整个文件内容读取到内存中可能会导致内存不足的问题。可以考虑使用逐行读取和写入的方法来处理大文件。例如:
with open('file1.txt', 'r', encoding='utf-8') as file1, \
open('file2.txt', 'r', encoding='utf-8') as file2, \
open('combined_file.txt', 'w', encoding='utf-8') as combined_file:
for line in file1:
combined_file.write(line)
combined_file.write('\n')
for line in file2:
combined_file.write(line)
通过这种方式,可以有效地处理大文件,避免内存不足的问题。
3. 日志记录
在实际项目中,为了方便调试和维护,可以使用日志记录来跟踪程序的执行情况。Python提供了内置的logging
模块,可以方便地进行日志记录。例如:
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
logging.info('Starting to read file1...')
try:
with open('file1.txt', 'r', encoding='utf-8') as file1:
content1 = file1.read()
except FileNotFoundError:
logging.error('File1 not found.')
content1 = ''
except IOError:
logging.error('An error occurred while reading file1.')
content1 = ''
logging.info('Finished reading file1.')
logging.info('Starting to read file2...')
try:
with open('file2.txt', 'r', encoding='utf-8') as file2:
content2 = file2.read()
except FileNotFoundError:
logging.error('File2 not found.')
content2 = ''
except IOError:
logging.error('An error occurred while reading file2.')
content2 = ''
logging.info('Finished reading file2.')
combined_content = content1 + '\n' + content2
logging.info('Starting to write combined file...')
try:
with open('combined_file.txt', 'w', encoding='utf-8') as combined_file:
combined_file.write(combined_content)
except IOError:
logging.error('An error occurred while writing to the file.')
logging.info('Finished writing combined file.')
通过这种方式,可以在程序运行时记录详细的日志信息,有助于快速定位和解决问题。
六、结论
本文详细介绍了如何使用Python实现两个txt文档的连接,包括文件读取、字符串拼接和文件写入等步骤,并提供了综合示例和实践建议。通过学习这些内容,相信读者可以更好地掌握文件操作的基本方法,并能够在实际项目中灵活应用这些技巧。
相关问答FAQs:
如何在Python中读取和合并两个TXT文件的内容?
在Python中,可以使用内置的文件操作功能来读取和合并TXT文件的内容。首先,使用open()
函数以读取模式打开两个文件,然后读取它们的内容。接着,可以将两个文件的内容合并,并将结果写入一个新的TXT文件中。以下是一个简单的示例代码:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged_file.txt', 'w') as merged_file:
merged_file.write(file1.read())
merged_file.write(file2.read())
这样,merged_file.txt
中将包含两个文件的内容。
在合并TXT文件时,如何处理文件内容中的空行和重复行?
在合并文件时,可以通过读取内容并进行处理来避免空行和重复行的出现。可以在读取每一行时使用条件语句检查行是否为空,或者使用集合来存储已读取的行,确保不再添加重复的行。例如:
unique_lines = set()
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged_file.txt', 'w') as merged_file:
for line in file1:
if line.strip(): # 检查是否为空行
unique_lines.add(line)
for line in file2:
if line.strip():
unique_lines.add(line)
for line in unique_lines:
merged_file.write(line)
这段代码将有效地合并两个文件,避免空行和重复行。
如何在合并TXT文件时保留原文件的格式和换行?
合并TXT文件时,如果希望保留原文件的格式与换行,可以直接将读取的内容写入新的文件,而不进行任何处理。可以使用readlines()
方法来读取文件的每一行,确保格式不变。例如:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged_file.txt', 'w') as merged_file:
merged_file.writelines(file1.readlines())
merged_file.writelines(file2.readlines())
这样,合并后的文件将完全保留原文件的格式和换行。
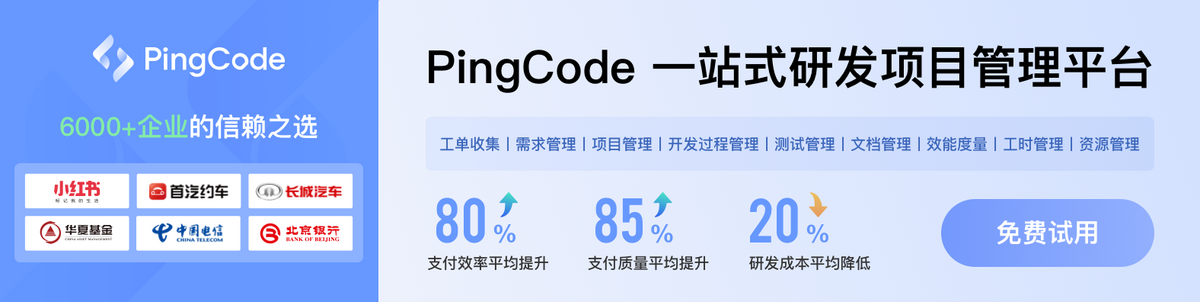