Python如何打开外部可执行文件,使用os.system、subprocess模块、os.startfile
Python提供了多种方法来打开和执行外部可执行文件,主要包括os.system、subprocess模块、os.startfile等。选择哪种方法取决于具体需求和环境。例如,os.system可以快速执行简单命令,而subprocess模块则提供了更复杂的功能,如捕获输出和错误处理。下面我们将详细介绍这几种方法,并提供代码示例和实际应用场景。
一、使用os.system方法
os.system
是一个简单直接的方式来调用外部命令。它在执行命令时不会返回输出结果,这意味着它更适合用于不需要捕获返回信息的简单任务。
import os
command = "notepad.exe"
os.system(command)
在这个例子中,os.system
方法打开了系统自带的记事本应用程序。虽然这种方法简单方便,但它有一些局限性,比如无法捕获命令的输出和错误信息。
os.system的优缺点
优点
- 简单直接:适合执行不需要获取返回值的简单命令。
- 跨平台:在不同操作系统上使用同样的调用方式。
缺点
- 无法捕获输出:执行结果不会返回,无法进行进一步处理。
- 安全性问题:容易受到命令注入攻击,不适合用于处理用户输入的命令。
二、使用subprocess模块
subprocess
是Python推荐的模块,用于执行外部命令。它提供了更强大的功能,包括捕获输出、错误处理、与子进程交互等。
示例代码
import subprocess
command = ["notepad.exe"]
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
if process.returncode == 0:
print("Command executed successfully")
else:
print(f"Error occurred: {stderr.decode()}")
在这个例子中,使用了 subprocess.Popen
来执行命令,并捕获了标准输出和标准错误。这种方法适合于需要获取执行结果的复杂任务。
subprocess模块的优缺点
优点
- 功能强大:支持捕获输出、错误处理、与子进程交互等。
- 安全性更高:可以使用列表形式传递命令,避免命令注入攻击。
缺点
- 相对复杂:需要更多的代码来实现简单任务。
- 兼容性问题:某些系统上的行为可能有所不同,需要进行额外测试。
三、使用os.startfile方法(仅适用于Windows)
os.startfile
是一种仅适用于Windows操作系统的方法,用于打开与文件类型关联的应用程序。例如,可以使用它来打开文本文件、网页等。
示例代码
import os
file_path = "C:\\Path\\To\\Your\\File.txt"
os.startfile(file_path)
在这个例子中,os.startfile
方法根据文件的扩展名自动选择合适的应用程序来打开文件。这种方法非常方便,但仅限于Windows环境。
os.startfile的优缺点
优点
- 便捷:无需指定具体的应用程序,系统会自动选择合适的程序。
- 简单:代码量少,易于实现。
缺点
- 仅限于Windows:无法在其他操作系统上使用。
- 不可控:无法捕获输出或错误信息,适用场景有限。
四、实际应用场景分析
1. 自动化办公任务
在自动化办公任务中,可能需要打开特定的应用程序来处理文件。例如,可以使用 os.startfile
来打开Excel文件,自动生成报告。
import os
file_path = "C:\\Path\\To\\Your\\Report.xlsx"
os.startfile(file_path)
这种方法简单直接,适合用于需要快速打开文件的场景。
2. 系统维护和监控
在系统维护和监控中,可能需要执行一些系统命令来获取状态信息。例如,可以使用 subprocess
模块来执行 ping
命令,并捕获输出结果。
import subprocess
command = ["ping", "www.google.com"]
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
if process.returncode == 0:
print(f"Ping successful: {stdout.decode()}")
else:
print(f"Ping failed: {stderr.decode()}")
这种方法适合需要获取执行结果并进行进一步处理的场景。
3. 安全性考虑
在处理用户输入的命令时,必须考虑安全性问题。subprocess
模块提供了更高的安全性,因为它支持使用列表形式传递命令,避免命令注入攻击。
import subprocess
user_input = "notepad.exe"
command = [user_input]
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
if process.returncode == 0:
print("Command executed successfully")
else:
print(f"Error occurred: {stderr.decode()}")
这种方法适合处理用户输入的命令,确保系统安全。
五、综合比较与选择
在选择方法时,需要根据具体需求和环境进行权衡。以下是几个关键点的比较:
方法 | 简单性 | 功能性 | 安全性 | 平台兼容性 |
---|---|---|---|---|
os.system | 高 | 低 | 低 | 跨平台 |
subprocess | 中 | 高 | 高 | 跨平台 |
os.startfile | 高 | 低 | 低 | 仅限Windows |
1. 如果只是执行简单命令且不需要获取返回结果,os.system
是一个不错的选择。
2. 如果需要更复杂的功能,如捕获输出和错误处理,subprocess
模块是最佳选择。
3. 如果在Windows上需要打开与文件类型关联的应用程序,os.startfile
是最便捷的方法。
六、最佳实践与代码优化
在实际应用中,通常需要考虑代码的可维护性和可扩展性。以下是一些最佳实践和优化建议:
1. 使用上下文管理器
在处理子进程时,使用上下文管理器可以确保资源的正确释放。
import subprocess
command = ["notepad.exe"]
with subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE) as process:
stdout, stderr = process.communicate()
if process.returncode == 0:
print("Command executed successfully")
else:
print(f"Error occurred: {stderr.decode()}")
这种方法可以确保在子进程执行完毕后,资源能够及时释放,避免潜在的内存泄漏问题。
2. 捕获输出并写入日志
在执行外部命令时,捕获输出并写入日志文件可以方便后续调试和问题排查。
import subprocess
command = ["ping", "www.google.com"]
with subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE) as process:
stdout, stderr = process.communicate()
with open("log.txt", "w") as log_file:
if process.returncode == 0:
log_file.write(f"Command executed successfully: {stdout.decode()}\n")
else:
log_file.write(f"Error occurred: {stderr.decode()}\n")
这种方法可以帮助开发者在出现问题时,快速定位和解决问题。
3. 参数化命令
在处理用户输入的命令时,使用参数化命令可以提高代码的安全性。
import subprocess
def execute_command(command):
with subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE) as process:
stdout, stderr = process.communicate()
return process.returncode, stdout, stderr
user_input = "notepad.exe"
command = [user_input]
returncode, stdout, stderr = execute_command(command)
if returncode == 0:
print("Command executed successfully")
else:
print(f"Error occurred: {stderr.decode()}")
通过将命令封装成函数,可以提高代码的可维护性和可扩展性。
七、总结
Python提供了多种方法来打开和执行外部可执行文件,主要包括os.system、subprocess模块、os.startfile等。根据具体需求和环境,可以选择合适的方法来实现。对于简单任务,os.system
是一个不错的选择;对于需要捕获输出和错误处理的复杂任务,subprocess
模块是最佳选择;在Windows上需要打开与文件类型关联的应用程序时,os.startfile
是最便捷的方法。
通过遵循最佳实践和优化建议,可以提高代码的可维护性和可扩展性,确保在不同场景下的稳定运行。希望本文能为你提供有价值的参考,助你在Python编程中更好地处理外部可执行文件的打开和执行问题。
相关问答FAQs:
如何使用Python打开外部可执行文件?
使用Python打开外部可执行文件通常可以通过subprocess
模块实现。该模块允许你启动新进程,并与其进行交互。你可以使用subprocess.run()
或subprocess.Popen()
等方法来执行可执行文件。以下是一个简单的示例:
import subprocess
# 使用subprocess.run()打开可执行文件
subprocess.run(['path_to_executable.exe', 'arg1', 'arg2'])
确保将path_to_executable.exe
替换为可执行文件的实际路径。
在Python中如何处理打开的可执行文件的输出?
为了捕获外部可执行文件的输出,可以在subprocess.run()
中设置stdout
参数为subprocess.PIPE
。这使得你可以在Python程序中处理可执行文件的标准输出和错误输出。例如:
import subprocess
result = subprocess.run(['path_to_executable.exe'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
output = result.stdout.decode() # 获取标准输出
error = result.stderr.decode() # 获取错误输出
这样可以方便地获取到程序的返回信息,便于进一步处理。
是否可以在Python中以管理员权限打开可执行文件?
是的,可以通过subprocess
模块以管理员权限打开可执行文件。在Windows系统中,可以使用runas
命令来实现这一点。示例如下:
import subprocess
subprocess.run(['runas', '/user:Administrator', 'path_to_executable.exe'])
执行时会要求输入管理员密码。请确保在使用此功能时遵循安全规范。
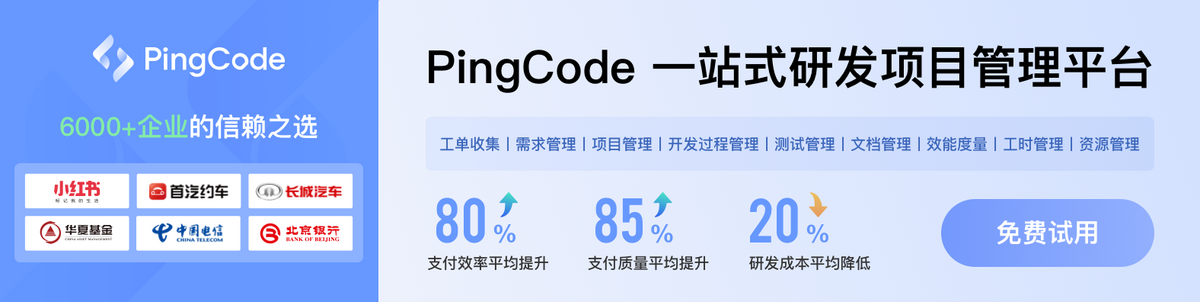